25 Swift Interview Questions and Answers for 2024
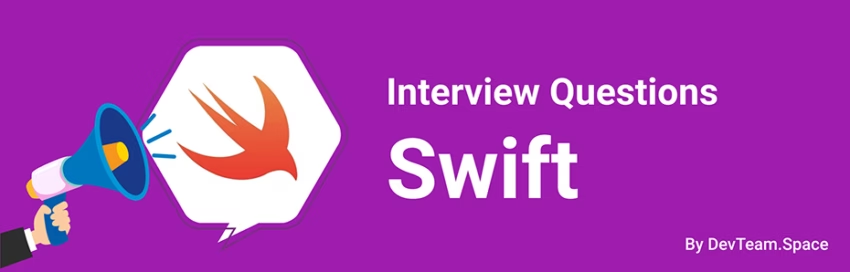
The First Priority is to Define the Job Requirements
The first step in the hiring process requires you to define what you need from your developer. Consider the following questions in this regard:
- Which platforms from Apple are you targeting?
- How complex is your project?
- How many developers do you need, and what are the seniority levels required?
- What kind of projects do you have, e.g., development, maintenance, or both?
Use your project specification to find exact answers to these questions in order so that you can outline the job description, responsibilities, and skills required from your developer.
Swift Developer Job Description
You most likely have one or more projects where you plan to develop apps targeting various platforms offered by Apple Inc. Your projects might involve developing new applications or maintaining existing applications. No matter what the project, you will likely need a Swift developer to effectively execute these projects.
The devices offered and supported by Apple, e.g., iPhone, iPad, Apple Watch, etc., are high-end and must meet Apple’s strict application guidelines. Consequently, the landscape of apps tends to be competitive. Naturally, you will want to offer the best possible user experience with the features. For this, you are definitely going to need top Swift developers.
Roles and Responsibilities for Swift Programming Language Developers
You will want a Swift developer to fulfill the following responsibilities:
- Understand the functional requirements by working with stakeholders like project managers, project owners, business analysts (BAs), etc.;
- Understand the technical solutions by collaborating with the architect;
- Develop program specifications;
- Code apps using Swift and unit-test them;
- Work with the testing and DevOps teams for testing and deployment of new apps;
- Maintain and enhance existing apps;
- Participate in process improvement activities;
- Communicate effectively with all stakeholders.
Skills and Competencies That you Need in a Swift Developer
You need a Swift developer with the following skills:
- In-depth knowledge of Swift and its rich ecosystem;
- Great understanding of object-oriented programming;
- Sufficient familiarity with Xcode;
- Sound knowledge of Cocoa and Cocoa Touch;
- Deep knowledge of memory management and multi-threading in languages like Swift, Java, etc.;
- Robust experience in popular relational database management systems (RDBMSs), NoSQL databases, embedded databases, key-value pairs databases, etc.;
- Familiarity with multi-paradigm programming languages like Python, JavaScript, etc.;
- Years of experience in developing RESTful APIs;
- Good understanding of user interface design including the “Human Interface Guidelines” used by Apple;
- Sufficient familiarity with C-based libraries;
- The experience of using cloud computing platforms;
- Async and Sync programming;
- Good knowledge of tools like GitHub;
- In-depth knowledge of test automation frameworks and popular DevOps tools;
- Sound understanding of “continuous integration” (CI)/”continuous delivery” (CD) concepts.
Now that you are familiar with these basics, we will now provide key interview questions for hiring Swift developers.
Since you might need questions for junior, mid-level, and senior developers, we have broken down the questions into these three types. Skip to whichever developer experience section applies to your project.
Swift Interview Questions for Junior Developers
Use the junior-level technical questions in a job interview if you plan to hire an entry-level or junior Swift developer.
Question 1: What is an “Optional” in Swift?
Answer: An optional value in Swift language enables any variable type to represent a “lack of value”. Swift extends the capabilities of Objective-C in this regard. Objective-C would allow an “absence of value” for reference types only. It would use the special value called “nil” for this.
Swift allows you to use the “lack of value” concept in both reference types and variable types, and it uses “optional” variables for that. You can have an “optional variable with a value or a “nil”.
Question 2: How does a “class” differ from a “structure”?
Answer: A “class” differs from a “structure” in the following ways:
- You can take the advantage of an “inheritance” when you use a “class”. A “structure” doesn’t support this.
- The Swift programming language defines “structures” as “value types” and “classes” as “reference types”.
Question 3: What purpose do “generics” serve in Swift?
Answer: “Generics” in Swift allow you to combine multiple functions into one function, furthermore, you can prevent variable types-related errors. This allows you to achieve more by coding less.
Consider an example where you test equality. The following code allows you to check if two integers are equal:
func areIntEqual(_m: Int, _n: Int) -> Bool {
return m==n
Now, assume that you will check equality for other data types. You need to write the following code to check if two strings are equal:
func areStringsEqual(_p: String, _q: String) -> Bool {
return p==q
}
Notice how you needed to duplicate code for different data types. You can combine these two pieces of code into one if you use “generics”. You need to code the following:
func areTheyEqual(_a: T, _b:T) -> Bool {
return a==b
}
You can restrict the data types to types that implement the “Equitable” protocol. This allows you to achieve more by coding less while you avoid variable type-related errors.
Question 4: How to parse JSON in Swift?
Answer: JSON parsing in Swift happens to be a common requirement in iOS swift development projects. iOS apps might decode JSON to represent data visually, and an iOS developer can use the basic APIs of Swift to do this.
The Swift “Decodable” protocol can decode JSON. Swift automatically converts every type that conforms to this protocol. Programmers don’t need to define each property that comes with a JSON, furthermore, the “JSONDecoder” in Swift can handle “optionals”. Developers can decode JSON arrays too.
Question 5: Which Swift keywords you can use for declaring variables and constants?
Answer: Swift developers need to declare variables and constants before they use them in a program. The “Var” keyword allows them to declare variables. They can use the “Let” keyword to declare constants.
Question 6: Why does “unwrapping” an “optional” in Swift have importance?
Answer: Unwrapping an optional value in Swift has plenty of importance. Consider the example of an “optional” string. It might have a value, however, it might also be “nil”.
You will find a challenge with this if you try to use the “count” property. For a regular string, the “count” property will show the number of letters in the string. An “optional” string can just be “nil” though. It doesn’t contain anything, therefore, it won’t have a “count”.
By its very design, Swift doesn’t allow such unsafe programming practices. This is where Swift programmers use “unwrapping”. This process enables programs to look inside the “optional”.
E.g., a Swift developer can use the “if let” syntax. This “unwraps” an optional and provides the count of letters if there’s any value at all.
You can use the “else” condition to display a message of your choice if the “optional” string contains “nil”. That also promotes safe programming.
One has several ways to “unwrap” an “optional”, however, some of them aren’t very safe. E.g., “forced unwrapping” and declaring “implicitly unwrapped” variables can often be unsafe. Developers need to use appropriate judgment before using them.
Question 7: How to write a multiple-line comment in Swift?
Answer: A Swift programmer can start a multiple-line comment with a forward slash, which will be followed by an asterisk (“/*”). The comment ends with an asterisk, which will be followed by a forward slash (“*/”).
Question 8: Which are the “control transfer statements” in Swift?
Answer: Swift features several “control transfer statements”. Using these can transfer the control of the program from one statement to another without any conditions. These statements are as follows:
- “Break”;
- “Continue”;
- “Fallthrough”;
- “Return”;
- “Throw”.
Question 9: What are “initializers” and “deinitializers” in Swift?
Answer: “Initializers” in Swift help to execute the “initialization” process. This process sets up an initial value for each stored property in an instance of a class, structure, or enumeration. Programmers use the “init” keyword to use “initializers”.
“Initializers” in Swift don’t return a value. They correctly initialize the new instances of a type before a program uses them for the first time.
Unlike “initializers”, a “deinitializer” works only on class types. Programmers use them immediately before deallocating an instance of a class. Swift developers need to use the “deinit” keyword to use “deinitializers”.
Question 10: Explain the differences between “Structures” and “Classes” in Swift with an example.
Answer: In Swift, “Structures” differ from “Classes” at a fundamental level. A “Structure” or “Struct” is a value type (All basic data types are also value types). A “Class” is a reference type.
A class is a blueprint to create a particular data structure, initial values for remember variables, and member functions.
Assume that you are creating an app to measure the level of visibility on two different days. You will assign numeric values of “1” and “2” to measure and compare visibilities. The code could look like the following if you use “Structures”:
struct Day {
var visibility: Int = 1
}
var day1 = Day()
var day2 = day1
Day2.visibility = 2
In this instance, “day1.visibility” is 1. The value of “day2.visibility” will be 2. Since “Day” was a “Structure”, the value types were copied by value.
The statement “var day2 = day1” created a copy of “day1” and assigned it to “day2”. Even if you change “day2”, it won’t reflect in “day1”.
That would change completely if “Day” was a “Class”. It would be a reference type then. Changes to “Day2” would reflect in “Day1”, and it would work the other way around too.
Question 11: Is the last sentence in the following code snippet acceptable during the compile time? Why, or why not?
Code snippet:
Import UIKit
Var viewM = UIView()
viewM.alpha = 0.6
let viewN = UIView()
viewN.alpha = 0.6
viewN = viewM
The question is whether the line “viewN = viewM” will compile.
Answer: This line will not compile. You can reassign “viewM” to a new instance of “UIView” since “viewM” is a variable. On the other hand, “let” allows you to assign a value only once. This makes “viewN” immutable. You can’t assign the value of “viewM” to “viewN”. This is why the Swift compiler won’t accept it.
Question 12: What is a dictionary in Swift code?
Answer: A dictionary is a basic data collection type. It stores an unordered list of values with the same data type. Dictionaries are also called hash maps that store key-value pairs to efficiently store and retrieve values based on their unique identifiers.
iOS Swift Interview Questions for Mid-level Developers
Planning to hire a mid-level Swift developer? Such developers have considerable experience in different kinds of projects. They might have developed iOS apps, furthermore, they might have worked on Swift on platforms like Linux.
Use the following interview questions:
Question 13: Which one among “nil” and “.none” should you use in Swift?
Answer: Experienced Swift developers recommend using “nil”. Having said that, “nil” and “.none” work in the same way in Swift. “.none” is the short form of “Optional.none”. It does exactly what “nil” does. The common convention among Swift developers is to use “nil”.
Question 14: How is defining a constant in Swift different from that in Objective-C?
Answer: You can define a constant in Objective-C using a piece of code like the following:
const int marks = 0;
In Objective-C, a “const” is a variable that you initialize at the compile time. You do that with a value or an expression, and the Objective-C compiler resolves it.
In the case of Swift, you can use a piece of code like the following:
let marks = 0
The “let” word creates an immutable that’s determined at the runtime. Developers can initialize it with a static. They can initialize it with a dynamic expression too.
Question 15: How to handle runtime errors in Swift?
Answer: Swift offers various ways to handle a runtime and a compile-time error. A few examples of runtime error handling are as follows:
- The Swift “error protocol”: Developers can create a custom “Error” type. They can use an enumeration that conforms to the Swift error protocol.
- The “throws” and “throw” keywords: Developers can add the “throws” keyword in the definition of a function or an initializer. This would propagate the error from the function to the piece of code that calls the function. Programmers can then use the “throw” keyword for throwing errors from the error type defined.
- The “do-catch” block and “try” statement: Functions with a “throws” keyword should have a “try” statement since such functions might have errors. A “try” statement should reside within a “do-catch” for it to execute.
- “Try” with a question mark: Swift allows programmers to code “try” with a question mark, i.e., “try?”. This will cause the return value of the “throwable” function to be “optional”. If the function throws an error, the value will be “nil”. Otherwise, it will be the wrapped return value of the function.
Question 16: For a Swift “enum”, how do “raw values” differ from “associated values”?
Answer: Developers use “raw values” to associate constants to “enum” cases. Swift doesn’t allow duplicate values, and each “enum” case must have a unique value.
Programmers can use the “rawValue” property to convert the value of an “enum” to its raw value. On the other hand, they can use a dedicated initializer to convert a raw value to an “enum” instance.
Developers can use “associated values” to associate data to an “enum” case. These cases can have zero or more such values. Swift developers need to use a “tuple” in the case definition.
Question 17: What is “optional chaining” in Swift?
Answer: A Swift “optional” might have “nil” at one point. “Optional chaining” in Swift allows developers to query and call properties of the optional. It also allows querying and calling the methods and subscripts of that “optional”.
The “optional” might contain either a value or a nil. Calls to properties, methods, and subscripts succeed in that case. These calls return a value of “nil” if the “optional” contains “nil”.
An advantage of “optional chaining” in Swift is that programmers can create multiple queries together. The entire chain of queries will fail if any of them returns a “nil”. Swift allows developers to handle such failures effectively too.
Question 18: How to define a “base class” in Swift?
Answer: Swift has a concept of “superclass”. Other classes can inherit properties, methods, and functions from a superclass. A Swift developer can define a class without specifying its superclass, which makes this class a “base class”.
Question 19: Explain the difference between equality and identity operators.
Answer: The equality operator or == compares two values and checks if the two values are the same or equal. Identity operator or === checks if the two references point to the same instance (or same memory address). The identity operator returns false when comparing two references to different object instances, even if the two instances have the same value.
Question 20: Explain the use of inout parameter.
Answer: When a function takes a large value, memory-wise, as an argument and returns the same type, moreover, the return of the function always replaces the caller argument, then inout as an associated function parameter is preferred to use. Using inout means changing the local variable will also change the passed parameters. A good use case is a swap function.
Question 21: What is defer in Swift?
Answer: A defer statement executes code just before transferring program control outside the scope where the defer statement appears.
Question 22: What is a nil coalescing operator?
Answer: The double question mark operator ?? is known as the nil coalescing operator. It returns the value on the left-hand side if it's not nil. If the left-hand side is nil then it returns the value on the right-hand side. Nil coalescing can be used as a shorthand for checking if an optional value is a nil. A double question mark is also used to provide a default value to a variable.
Senior Swift Developer Interview Questions
Senior Swift developers have experience in delivering complex projects successfully, furthermore, they also know Swift algorithms well. They can even contribute to creating Swift tutorials.
Question 23: How to determine if an integer is a palindrome?
Answer: If an integer reads the same backward as forward, then it’s a palindrome. Note that “565” is a palindrome since it would read the same backward. However, “-565” isn’t a palindrome. It would read “565-“ if you read it backward.
The following code would determine if an integer is a palindrome:
Class Palindrome {
func isPalindrome(_a: Int) -> Bool {
if a < 0 || (a % 10 == 0 && a != 0) {return false }
var b = a
var result = 0
while(b != 0){
var mod = b % 10
b /= 10
result = result * 10 + mod
}
If ( a != result ) { return false }
return true
}
}
Question 24: How to resolve a circular reference in Swift?
Answer: In the case of the Swift language, a circular reference involves two instances holding a strong reference to each other. This causes a memory leak. That’s because neither of the 2 instances will be deallocated.
The rules of strong references in Swift prevent you from deallocating instances if there’s a strong reference to them. Each of the instances will keep the other alive. That makes deallocation impossible.
Developers need to replace one of the strong references with a “weak” reference. They can also use an “unowned” reference. These methods will enable them to resolve a circular reference.
Question 25: Explain how the “map” and “reduce” methods work in Swift with an example.
Answer: Let’s consider an array containing the names of a few fruits and their unit price. The following code snippet shows how the “map” and “reduce” methods will work:
struct Fruit {
let name: String
let Price: Double
}
let Fruits = [
Fruit(name: "Apple", Price: 0.322),
Fruit(name: "Orange", Price: 0.701),
Fruit(name: "Kiwi", Price: 1.02),
Fruit(name: "Pear", Price: 1.23),
Fruit(name: "Watermelon", Price: 1.44),
Fruit(name: "Fig", Price: 1.66),
Fruit(name: "Date", Price: 1.86),
Fruit(name: "Grape", Price: 1.97)
]
let exhibit1 = Fruits.map { $0.name }
let exhibit2 = Fruits.reduce(0) { $0 + $1.Price }
In the above-mentioned example, “exhibit1” is an array of strings. It would have the list of names of fruits. “exhibit2” is a “double”, which would show the sum of all unit prices.
The “map” method of the “Array” “struct” type transforms the source array into a different array that has another type. The method obtains these values by executing the closure passed as a parameter to each of the elements of the array.
Many functions in iOS accept multiple parameters, with the last parameter being a closure. The “closure” returns the “name” property. As a result, the “map” method returns the names of the fruits.
The “reduce” method of the “Array” “struct” type provides a single value. It does that by recursively applying the “closure” to each element of the array.
In this case, the “closure” takes the value calculated in the previous step. It takes the initial value for the 1st iteration. Furthermore, it takes the current array element. In this example, the “reduce” method will return the sum of the unit prices of all fruits.
Summary
The above questions should help you judge the skills of a candidate during a job interview. You can also print this swift questions-and-answer sheet.
If you wish to quickly and safely onboard Swift developers without the hassle of having to interview and vet them yourself then contact us at DevTeam.Space.
All of our developers are fully vetted and performance monitored throughout the project. You will only be matched with the most suitable ones who have experience in your industry. Since all their work is guaranteed by the DevTeam.Space, we provide a zero-risk solution to ensure your project succeeds.