- Developers
- Hiring Interview Tips
- JavaScript Interview Questions and Answers for 2024
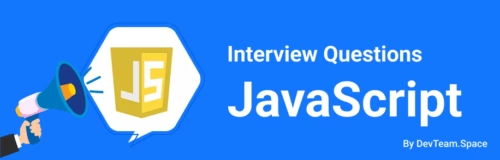
profile

By Aran Davies
Verified Expert
8 years of experience
Aran Davies is a full-stack software development engineer and tech writer with experience in Web and Mobile technologies. He is a tech nomad and has seen it all.
Whether you’re an entrepreneur, a project manager in a large enterprise, or a CTO, you are well aware that the success of your JavaScript project rests on your ability to find top developers.
In this guide, you‘ll find example interview questions and answers you can refer to when seeking a new JavaScript developer to make your dynamic user interfaces come to life. You‘ll also get some practical advice on how to use these questions to reliably identify the best people for the job.
First Things First: Select Your Job Requirements
The biggest mistake you can make as an interviewer is not knowing exactly what kind of JavaScript developer you are looking for before you sit down with your candidates. And no, “a great JavaScript developer with lots of experience” is not specific enough.
You need to be able to reliably identify the right candidates capable of completing the JavaScript-specific tasks for your project with your team. To do that, you need to figure out your specific job requirements.
Some example requirements for a JavaScript developer include:
- Essential web development skills – HTML, CSS
- JavaScript-specific skills – Libraries and toolkits, testing code
- Design skills – Building scalable applications, security
- Communication skills – Discussing problems and constraints
- Initiative – Figuring out solutions by themselves
Avoid making a laundry list of non-essential skills for your perfect JavaScript developer. Instead, focus on what your candidate will actually be doing day-to-day. Keep your requirements list as short as possible. Cut anything they can do without or learn on the job.
With clearly stated requirements, you‘ll be able to choose the right JavaScript coding interview questions and have a much better idea of what kinds of responses you are looking for.
The questions in this guide are broken down into two sections: Junior Developer Interview Questions and Senior Developer Interview Questions. You should use the Junior Developer Basic JavaScript Interview Questions in this section if you‘re looking for a junior or entry-level developer with less experience. Skip to the Senior Developer sections for JavaScript interview questions and answers for experienced developers.
The job description of a JavaScript developer
You will typically need a JavaScript developer to work on web or cross-platform mobile development projects. They might need to work on maintenance projects too.
You need robust skills and experience when you hire a JavaScript developer. Web or mobile apps are typically customer-facing applications. You need to offer the best user experience while delivering functionality. Therefore, hiring experienced developers is important.
Organizations periodically undertake process improvement initiatives, and your organization might do that too. You would need the JavaScript developer to participate in such initiatives.
Job responsibilities of a JavaScript developer
You will need a JavaScript developer to fulfill the following responsibilities:
- Understanding business requirements by working closely with business analysts (BAs);
- Understanding the technical solutions by working with the architects;
- Designing technical specifications for new JavaScript-based applications;
- Coding new applications including the front and backend;
- Unit testing new applications;
- Working with the testing and DevOps teams to ensure smooth testing and deployment;
- Maintaining existing applications;
- Communicating with all relevant stakeholders effectively;
- Collaborating with the larger team;
- Participating in process improvement initiatives.
JavaScript Junior Developer Interview Questions
A Junior developer is someone just starting their career with less than 2 years of experience. They demand the lowest salary, but they‘ll need guidance from more experienced JavaScript developers who can delegate tasks and cross-check their code.
Skill Requirements for Junior JavaScript Developers
- Essential web technologies (HTML, CSS);
- Foundational JavaScript programming knowledge;
- Learning on the job;
- Following instructions and receiving feedback;
- Thinking like a programmer.
Example JavaScript Junior Developer Interview Questions and Answers
Note: Important keywords are underlined in the answers. Bonus points if the candidate mentions them!
Or save yourself time and request a team to match your needs right away.
Question 1: What is JavaScript?
Requirement: Foundational JavaScript Knowledge
Answer: JavaScript is a high-level, object-based, dynamically typed, weakly typed, interpreted,
multi-paradigm programming language. It is one of the three core web technologies alongside HTML and CSS. It was originally a client-side object-oriented scripting language but can also be used server-side.
Question 2: What are JavaScript Data Types?
Requirement: Foundational JavaScript Knowledge
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
Answer: Number, String, Boolean, Function, Object, and Undefined.
Question 3: Describe the difference between undeclared and undefined variables.
Requirement: Foundational JavaScript Knowledge
Answer: Undeclared variables have not been declared in the scope of the program and don‘t exist. If a JavaScript program tries to read an undeclared variable, a runtime error will occur.
Undefined variables have been declared but haven‘t been assigned a value. If a JavaScript program tries to read an undefined variable there won‘t be an error, but an undefined value will be returned for an undefined var.
Question 4: Write some example JavaScript code that creates a new HTML <p> element with some text, and appends it to the document body. Use jQuery syntax if needed.
Requirement: Foundational JavaScript Knowledge
Answer:
var para = document.createElement("P"); // Create a <p> element
var t = document.createTextNode("This is a paragraph"); // Create a text node
para.appendChild(t); // Append the text to <p>
document.body.appendChild(para); // Append <p> to <body>
Question 5: Write an example definition of a JavaScript object for a ’person‘. The object in JavaScript should have a first and last name, an id number, and a function definition to retrieve the full name of the person.
Requirement: Foundational JavaScript Knowledge
Answer: Here you‘re looking for basic knowledge of how to turn spoken requirements into working JavaScript code. Look out for things like naming conventions, neat code structure, correct use of keywords, correct semicolon use, and syntax error. An acceptable answer should look something like this:
var person = {
firstName : "John",
lastName : "Doe",
id : 0001,
fullName : function() {
return this.firstName + " " + this.lastName;
}
}
Question 6: Describe what ’dynamic typing‘ means for JavaScript. Give code examples.
Requirement: Foundational JavaScript Knowledge
Answer: Dynamic typing means that the type of variables can change at runtime. For example, this is perfectly ok in JavaScript:
n = 1;
n = “Some string”;
This is opposed to static typing, where the programmer must explicitly state the type of each variable, and conversions to unrelated types must be done explicitly.
Question 7: Write an example of a simple HTML document with some header information and some page content.
Requirement: Basic HTML skills
Answer: HTML documents are different, but they follow a basic structure of head and body. The different sections in html pages are marked with tags such as DOCTYPE, html, head, title, meta, body, h1, p.
For example:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<meta charset="UTF-8">
<meta name="description" content="Page description">
</head>
<body>
<h1>Interview Example Web Page</h1>
<p>Some content goes here</p>
</body>
</html>
Question 8: Explain JavaScript callback functions and provide a simple example.
Requirement: Basic JavaScript skills
Answer: A callback function is a function that is called after another function has finished executing. A callback function is passed to another function as an argument and is invoked after some operation has been completed. For example:
function modifyArray(arr, callback) {
arr.push(100);
callback();
}
var arr = [1, 2, 3, 4, 5];
modifyArray(arr, function() {
console.log("array has been modified", arr);
});
Question 9: How would you learn about a new JavaScript library?
Requirement: Learning on the job
Answer: Web development is changing all the time, and developers need to be able to learn constantly. Here you are finding out how the candidate approaches the learning process. You want to see that they have an idea of what information they will need and where to find it. For JavaScript libraries, that means looking up online tutorials and digging into the documentation.
Question 10: Describe a time you received feedback on a programming task. How did it help you become a better programmer?
Requirement: Following instructions and receiving feedback
Answer: This is a typical open-ended question. The candidate should demonstrate they can accept, understand and act on feedback.
Question 11: What will be the output when the following code is executed? Explain.
console.log(false == '0')
console.log(false === '0')
Answer:
true
false
Explanation: == equal to, === equal to with datatype check
JavaScript Senior Developer Interview Questions
Here are some more advanced and technical interview questions and answers for experienced JavaScript developers. Use them to pick out the right JavaScript developers with the skills to build your web app.
Modern web development is all about understanding how to use tools, libraries, and toolkits and integrate many technologies together. Expert developers need to know more than just how to write JavaScript code. They need to know how that code will work in the context of the application and the entire web.
For this reason, you should include a lot of open-ended, ’messy‘ interview questions. These should spark a discussion and you should tailor them to fit your own job requirements. Don‘t be afraid to ask follow-up questions!
Skill Requirements for Senior JavaScript Developers
- Expert JavaScript knowledge;
- Using a range of web technologies;
- Designing for specific requirements (e.g. security, scalability);
- Node.js and server-side JavaScript;
- Maintaining and upgrading JavaScript applications;
- Experience in frameworks/toolkits/libraries you use;
- Efficient programming and clean code;
- IIFEs (immediately invoked function expressions);
- HTML parser for HTML code’s parsing;
- Debugging;
- Automated testing;
- Leadership skills;
- Clear communication skills;
- Mentoring less experienced developers.
Example JavaScript Senior Developer Interview Questions and Answers
Note: Important keywords are underlined in the answers. Look out for them in interviews!
Question 12: List some advantages and disadvantages of JavaScript.
Requirement: Expert JavaScript knowledge
With this question, you‘re looking for knowledge of how programming languages work, and why there are always tradeoffs to any specific web technology or language.
Answer:
Advantages:
- Being able to compile and run immediately within the browser makes it fast in many cases;
- It‘s interoperable with many other languages and can be inserted into any webpage;
- A huge number of tools, libraries, and frameworks are available;
- Most programmers know at least some JavaScript and extensive resources available to learn JavaScript;
- It‘s a highly versatile language;
- You can use JavaScript as a server-side language with Node.js;
- Allows web pages to have interactive elements and interfaces.
Disadvantages:
- JavaScript has some security vulnerabilities. Code compiled and run on the client side with predefined objects can be exploited by attackers;
- Features like dynamic typing can make JavaScript code prone to errors;
- JavaScript may run differently on different web browsers.
Question 13: What will be the output of the following code?
Requirement: Expert JavaScript knowledge
var foo = true;
console.log(!foo);
console.log(foo + 0);
console.log(foo + "xyz");
console.log(foo + false);
console.log(foo && foo);
Answer: Here you‘re looking for knowledge of how variable typing and the logical operators work in JavaScript. It will output the following to the console:
true
1
truexyz
1
true
Question 14: What will the following code output? Why?
Requirement: Expert JavaScript knowledge
function foo(){
function bar() {
return ’a‘;
}
return bar();
function bar() {
return ’b‘;
}
}
console.log(foo());
Answer: Here you‘re testing detailed knowledge of a critical JavaScript concept called Hoisting.
Counterintuitively, the program will output b. This is because both of the bar() functions in the code snippet will be hoisted to the top of the scope. The second one returning the string ’b‘ will be hoisted after the first, so that function will be called in the return statement.
Question 15: What is the ‘this’ keyword in JavaScript? How do you use it?
Requirement: Expert JavaScript knowledge
Hire expert developers for your next project
1,200 top developers
us since 2016
Answer: The ‘this’ keyword refers to the object from where it was called. In their answer, the candidate should tell about the call, bind, and apply.
The functions .call() and .apply() are very similar in their usage, except for one small difference. .call() is used when the number of the function’s arguments is known to the programmer, as they have to be mentioned as arguments in the call statement. On the other hand, .apply() is used when the number is not known. The function .apply() expects the argument to be an array object.
.bind returns a new function(does not invoke it immediately) with the new context
Question 16: How do you explain closures in JavaScript? When are they used?
Requirement: Expert JavaScript knowledge
Answer: A closure is a locally declared variable related to a function that stays in memory when the function has returned. They give access to an outer function’s scope from the inner function’s scope. Take a look here for a deeper understanding: https://medium.com/dailyjs/i-never-understood-javascript-closures-9663703368e8
For example:
function greet(message) {
console.log(message);
}
function greeter(name, age) {
return name + " says howdy!! He is " + age + " years old";
}
// Generate the message
var message = greeter("James", 23);
// Pass it explicitly to greet
greet(message);
this function can be better represented by using closures
function greeter(name, age) {
var message = name + " says howdy!! He is " + age + " years old";
return function greet() {
console.log(message);
};
}
// Generate the closure
var JamesGreeter = greeter("James", 23);
// Use the closure
JamesGreeter();
Question 17: How do you copy an object by reference? How do you copy one by value?
Requirement: Expert JavaScript knowledge
Answer:
a = {'w': 1}
b = a // copies reference to an object
c = Object.assign({}, a) // creates new object which copies the value of A
Question 18: Why is namespacing important when coding in JavaScript? Give an example of how you would implement namespacing to group this sample configuration data:
Requirement: Namespacing
var DEBUG = true;
var MAX_SIZE = 100;
var FEED_URL = "http://myfeed.com/feed/";
var POST_IDS = [1, 2, 3];
Answer: Namespacing is used to group things like variables, functions, and objects together with unique names. It helps to avoid naming conflicts, logically separate code, and make code more reusable.
Namespacing in JavaScript is often done using JavaScript objects. For the example above:
var Config = {
DEBUG: true,
MAX_SIZE: 100,
FEED_URL: "http://myfeed.com/feed/",
POST_IDS: [123, 456, 789]
};
A variable in this namespace can be accessed like this:
Config.MAX_SIZE;
All JavaScript objects inherit properties from a prototype. For example, Date objects inherit properties from the Date prototype, Math objects inherit properties from the Math prototype, Array objects inherit properties from the Array prototype, etc.
Question 19: Give some ideas to implement automated testing on our JavaScript code.
Requirement: Testing JavaScript applications
Answer: Automated testing is essential when building robust applications. Great developers are keenly familiar with and can implement:
- Unit testing – Testing individual functions and classes
- Integration testing – Testing that components work together as expected
- UI/Functional testing – Testing the interface and functioning of the actual application
Some examples of JavaScript testing tools include Jest, Mocha, Jasmine, Karma, Cucumber, Chai, Unexpected, enzyme, Ava, Protractor, Casper, Phantom, and many more.
Question 20: What are your favorite JavaScript frameworks and technology stacks? Why?
Requirement: Using a range of web technologies
Answer: Effective web development requires using and combining the many different technologies available. Here you‘re getting an idea of your candidate’s depth and breadth of experience in these tools. You should probe for specific technical details about the answers.
Question 21: What architectures and design patterns have you used for building JavaScript applications? What were the tradeoffs?
Requirement: Web application design
Answer: This question is about architecture and design patterns and is distinct from the question above. It‘s for senior candidates that will be part of the design process for your application. Ideally, a candidate will have experience working with multiple software architectures and understand the tradeoffs of each.
Some examples they might talk about include model-view-controller pattern, flux architecture, n-layered, microkernel architecture, microservices, monolithic, client-server, peer-to-peer, master-slave pattern, pipe-filter pattern, broker pattern, event-bus pattern, interpreter pattern, etc.
Question 22: What‘s your experience with using server-side JavaScript?
Requirement: Node.js and server-side JavaScript
Answer: You‘ll need to ask this if your job requires server-side JavaScript using Node.js. Server-side JavaScript requires different skills than client-side JavaScript.
Question 23: If you could use whatever tools you like to build our ______ application, what would you use?
Requirement: Design skills, understanding of requirements
Answer: In this question, you are getting a feel for the type of developer you are talking to and how they like to code. You are especially looking for developers that try to understand the requirements first. It‘s a big red flag if a developer gives a list of libraries and tools without understanding the task. Take note to see if their answer is the same as their ’favorite‘ technology stack.
Question 24: How are you involved in the JavaScript/Web development community?
Requirement: Passion for web development
Answer: This is a very popular question for coding interviews because community and open-source involvement are clear indicators of a passionate developer.
Question 25: Describe a time you fixed a particularly difficult error in a web application. How did you approach the problem? What debugging tools did you use? What did you learn from this experience?
Requirement: Debugging, Breaking down a problem into parts
Debugging is one of the key skills for any web developer. However, the real skill is in breaking the problem down practically rather than finding small errors in code snippets. Debugging often takes hours or even days, so you don‘t have time in an interview setting. Asking these questions will give you an idea of how your candidate approaches errors and bugs.
Answer: In the candidate‘s response you should look out for things like:
- A measured, scientific approach;
- Breaking down the problem into parts;
- Finding out how to reproduce the error;
- Knowledge of debugger keyword;
- Expressing and then testing assumptions;
- Looking at stack traces;
- Getting someone else to help/take a look;
- Searching the internet for others that have had the same problem;
- Writing tests to check if the bug returns;
- Checking the rest of the code for similar errors;
- Turn problems into learning experiences.
Question 26: What’s the most important thing to look for or check when reviewing another team member’s code?
Requirement: Mentoring less experienced developers, Leadership skills
Answer: Here you‘re checking for analysis skills, knowledge of mistakes that less experienced developers make, keeping in mind the larger project, and attention to detail.
A good answer might mention code functionality, readability and style conventions, security flaws that could lead to system vulnerabilities, simplicity, regulatory requirements, or resource optimization.
Question 27: What tools & practices do you consider necessary for the Continuous Integration and Delivery of a web application?
Requirement: DevOps systems design, Maintain and upgrade applications
Intricacies in JavaScript that you should focus on while choosing JavaScript interview questions
JavaScript has its share of intricacies and complexities. You need to keep them in mind when interviewing candidates. Keep the following in mind:
1. Handling “Null” in JavaScript
“Null” should mean “nothing”. That’s the case with JavaScript too. However, the data type “null” is an object in this programming language. You need JavaScript programmers that know the implications of this. E.g., they should know that they can set this object to “null”, which empties the object.
2. Good knowledge of Java can help
JavaScript and Java are two different languages, however, good knowledge of Java can help JavaScript programmers. Your project will involve server-side development. Java, an object-oriented programming language, can help greatly there with its object-oriented programming abilities. JavaScript developers with Java code skills can create apps that run on a virtual machine or browser. Look for Java skills when you interview JavaScript developers.
3. In-depth understanding of DOM (Document Object Model)
You need JavaScript programmers with deep knowledge of DOM. It’s an important interface that’s platform-agnostic. DOM is one of the W3C (World Wide Web Consortium) standards like browser object model, therefore, it’s not specific to JavaScript only. It’s a language-agnostic standard.
DOM allows your program to access the content of a web page or document dynamically. Your program can update the content, structure, and style of the document. Programmers with good knowledge of DOM will add significant value to your project. They should know how to use methods like “getElementByClass” for getting all the elements with the given class name.
4. The importance of knowing about the “strict mode”
Remember that JavaScript is a dynamically-typed programming language. That offers plenty of freedom to developers, however, it has a drawback too. Programmers don’t need to define types of variables in JavaScript. This can result in variable type-related bugs, which makes debugging hard.
Hire expert developers for your next project
However, you can use the JavaScript “strict mode”. You might have coded using “bad syntax”, which was accepted earlier. The “strict mode” won’t accept them though. It will show them as errors. Therefore, you can’t create a new global variable as a result of variable type-related errors anymore.
You can eliminate common bugs by using the “strict mode”. This helps you to create more secure programs too. Hackers commonly exploit common programming bugs, therefore, reducing bugs mitigates this risk. Look for JavaScript developers with a good understanding of the “strict mode”.
5. Using the “Not-a-Number” (NaN) or “isNaN” function in JavaScript
You should expect a JavaScript developer to know how to handle the “Not-a-Number” (NaN) function. JavaScript has a specific function for this, which is called “isNaN”. This function identifies whether a value is an illegal number. The “isNaN” function returns a “Boolean” value, i.e., “True” or “False”.
6. Utilizing the “const” keyword in JavaScript
You need a JavaScript developer to have good knowledge of keywords. One such important keyword is “const”. Developers should know that this keyword doesn’t define a constant value.
The “const” keyword defines a constant reference to a value. Developers can’t change the primitive values, however, they can change the properties of the object. The knowledge of using this keyword can help developers considerably.
7. Focus on the nature of a global variable as part of the questions and answers during the interview
When you interview a JavaScript developer, focus on the concepts of the global variable declared outside a function scope. Developers should know that JavaScript global variables are defined outside a JavaScript function.
One might even declare a global variable with a “window object”. Any function in the program can access such a global variable since these variables have a global scope.
JavaScript developers should know how to declare global variables. They should know the appropriate syntax for using a “window object”. A JavaScript programmer needs to know how to use the properties of a global object, e.g., the “Infinity” property.
8. Understanding the “hoisting” concept in JavaScript
You need JavaScript developers with sufficient experience. We talked about the fact that JavaScript has plenty of intricacies and complexities. These make the language very versatile. Developers might struggle with these powerful features though if they don’t understand how these work.
A good example is a concept of “hoisting” in JavaScript. You can use a variable in JavaScript even before you declare it. Sounds odd, doesn’t it?
For example, hoistedVariable = 9; console.log(hoistedVariable); // outputs 9 though the variable is declared after it is initialized vat hoistedVariable;. How can a programming language allow you to declare a variable after you use it?
The “hoisting” concept of JavaScript comes into the picture here. JavaScript moves all declarations to the top of the current scope by default. From the perspective of program execution, a declaration happens before the program uses a variable. Look for developers with in-depth conceptual knowledge.
9. The importance of knowing the JavaScript data types
Look for deep knowledge of JavaScript data types when you hire JavaScript coders. JavaScript has the following types of data types:
- Primitive data types;
- Non-primitive (reference) data types (to store multiple and complex values).
Developers should know how to use types.
The primitive data types in JavaScript are as follows:
- String;
- Number;
- Boolean;
- Undefined.
On the other hand, the non-primitive data types are as follows:
- Object;
- Array.
10. Using the “callback” function in JavaScript
You will surely judge how well a developer understands the various JavaScript functions. That’s an important part of the interview. Focus on the “callback” function when you test the knowledge of the candidate.
By using this function, a programmer can pass a number of arguments to another function. The program is actually passing a function as an argument. As a result, this allows one function to call another function. Developers with knowledge of such functions have a better chance to use JavaScript effectively.
11. The knowledge of function declarations in JavaScript
You need JavaScript developers with knowledge of a function declaration. Programmers need to define functions with the “function” keyword. JavaScript makes a note of the declared functions, and it executes them later. The execution of functions happens after invoking functions only.
12. Focus on the knowledge of function expressions in JavaScript
Smart JavaScript developers know sufficiently about function expressions. We already talked about the importance of functional declarations in JavaScript. JavaScript offers another way to create functions too, and it’s called a function expression.
Knowing various ways of creating functions can help developers. They can utilize the versatility of JavaScript better that way, therefore, you should look for this depth in knowledge.
13. The understanding of asynchronous programming
You need JavaScript developers that know the concepts of asynchronous programming. JavaScript offers this capability, and that’s an advantage.
Asynchronous programming enables JavaScript to execute statements without waiting for other statements to execute completely. Even if it’s executing a long-running JavaScript function, the UI and server will not become unresponsive.
Asynchronous programming helps developers to offer a great user experience. This makes the knowledge of this concept important when you hire JavaScript developers.
14. The knowledge of functional programming
JavaScript allows functional programming, which is a key advantage. You need to hire JavaScript developers with knowledge of functional programming. They should know about immutability, function composition, recursion, pure functions, etc.
Look for knowledge of algorithms in JavaScript. You need developers that know how to use arrays using the array literal, therefore, they should know methods like “forEach”. A JavaScript programmer should know about array iteration methods. Furthermore, they should know how to use functions like “FOO”, “eval”, etc.
15. In-depth understanding of undeclared variables
JavaScript allows undeclared variables. These are variables that are not initialized or declared earlier. Developers might not have declared them using the “var” keyword or the “const” keyword. Smart JavaScript developers should know how to use undeclared variables effectively, therefore, you should judge that during the interview.
16. The knowledge of using an arrow function
We talked about JavaScript functions earlier, and an arrow function is one of them. You can write a shorter function syntax when you use an arrow function. This helps developers, therefore, you should look for knowledge of arrow functions.
17. The knowledge of HTML and the “innerHTML” property
You need JavaScript programmers with good knowledge of HTML. Web application development projects require the external JavaScript skill of embedding JavaScript code into an HTML file and importing a JavaScript source file into an HTML document, furthermore, you should look for CSS skills too.
Your app might need to display the HTML content of an element, and developers should know how to do this. The “innerHTML” property of JavaScript helps with this. Look for programmers that understand this.
18. The knowledge of using an anonymous function
There are occasions when JavaScript developers need to use anonymous functions. These functions don’t have a name, therefore, developers should know how to call them. Look for programmers with this knowledge.
19. The understanding of using a constructor
You should look for JavaScript coders that know how to use a constructor. Using a constructor function involves certain rules and restrictions. E.g., you can create objects using an object literal and add object properties to them, however, this method doesn’t allow you to add a property to an object constructor.
20. Other key knowledge areas for a JavaScript developer
While interviewing, judge the knowledge in the following areas:
- Using console.log;
- Utilizing the “typeof” operator and its execution inside the if statement as the if statement code executes at run time;
- Writing JavaScript code that’s easy to maintain;
- Developing a RESTful API;
- Using an inner function or an outer function;
- Handling a local variable;
- Developing the back-end using a runtime environment like Node.js;
- “Object-Oriented Programming” (OOP);
- Using an operand;
- Utilizing methods like “setTimeout”, “str”, concatenation, etc.;
- Appropriate guidelines for assigning a JavaScript variable name;
- Using libraries like jQuery;
- Using a “Namespace”;
- Using rest parameter syntax to create functions;
- Knowledge of self-invoking functions;
- How a scripting language works;
- Using the JavaScript “return” function;
- How to avoid a “Reference Error”;
- JavaScript engines;
- Understanding the case-sensitive nature of JavaScript;
- Using objects like “SyntaxError”;
- Using expressions like “RegExp [abc]”.
Summary
Hiring the right people for your development team is critical to the success of your project. Remember that you should not be aiming to hire the perfect JavaScript developer, but rather the right person for the job at hand.
With the help of this information and sample interview questions on JavaScript, you can ensure that the hiring process goes smoothly and successfully – allowing you to hire a great programming team to get your project completed on time and on budget.
Happy Hiring!