22 Java Interview Questions and Answers for 2025
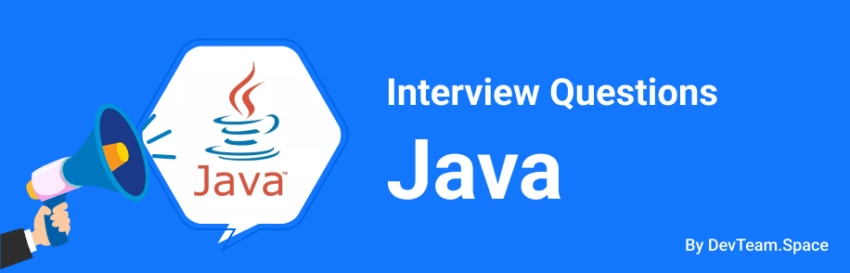
Whether you're an entrepreneur, a project manager in a large enterprise, or a CTO, you are well aware that the success of your project rests on your ability to hire great developers.
In this guide, you'll find example interview questions and answers that you can use when seeking a new Java developer. You'll also get some practical advice on how to use these questions to reliably identify the best people for the job.
Java and Its Continued Relevance
Let me start with a hypothetical question: why would you look for Java developers? After all, aren’t there highly modern programming languages around? The answer lies in the continued relevance of Java even after decades.
When James Gosling at Sun Microsystems first came up with Java in 1995, not many might have foreseen its sustained popularity. This class-based, object-oriented programming language continues to have a profound impact in the world of software development though.
A series of releases over the decades have added powerful features to Java. The latest stable release of this statically-typed language was Java SE 13, which was released in September 2019.
Java offers a wide range of advantages, which are as follows:
- Developers can learn it easily, thanks to its simplicity.
- While Java uses a lot of best practices from languages like C and C++, it removed complexities like pointers.
- Java supports object-oriented programming.
- It’s a robust language, thanks to features like automatic heap and stack memory garbage collection, simple memory management, and generics.
- Being a statically-typed language, Java is highly secure.
- Java compiles into a Java bytecode, therefore, the Java Virtual Machine (JVM) can execute it fast. As a result, Java helps you to code performant apps.
- This powerful object-based programming language allows multi-threading. Threads are created by extending the thread class and overriding the run method. Code synchronization is done using locks on a Java object.
- Its portability is a great advantage of Java since you can run it in any system with a JVM.
- You already know about the rich ecosystem that supports Java, don’t you? We don’t think that we need to elaborate on that!
- Java enables you to develop highly scalable apps.
- You can easily develop cross-platform applications with Java.
- Java has several in-built security features.
Want to read about these advantages in more detail? Check out our guide “Why should you use Java for your backend infrastructure?”.
The Popularity of Java
Even after decades of its advent, Java continues to enjoy a lot of popularity. The Stack Overflow developer survey 2022 report states that 33.27% of surveyed developers use it. Java remains one of the 5 most popular languages, according to this report. Let me start with a hypothetical question: why would you look for Java developers?
The 2023 Hacker Rank developer skills report has identified Java as a popular language too. Despite having so many modern languages as its competitors, it was the 2nd most popular language that developers learned in 2018. 70.69% of the surveyed developers learned this language in 2018.
First Things First: Select Your Job Requirements
Ultimately, an interview is about finding someone capable of producing the Java code you need while working effectively with your team. To do that, you need to figure out your unique job requirements.
Some example requirements include:
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
- Programming skills - E.g. Object-Oriented Design
- Java-specific skills
- Library/toolkit experience - E.g. JUnit
- Design skills - Performance optimization, building scalable applications, concurrency
- Communication skills - Discussing problems and constraints
- Being a self-starter - if they'll have to figure out solutions by themselves
Avoid making a shopping list of your perfect Java developer. Instead, focus on what your candidate will really be doing day-to-day. You should keep your requirements list as short as possible. Cut anything they can do without or learn on the job.
With clearly stated requirements, you'll be able to choose the right Java coding interview questions and have a much better idea of what kinds of responses you are looking for.
In this article, we‘ll categorize the Java coding interview questions based on the level of expertise you‘re looking for - Junior, Mid-level, or Senior. Some of the questions will overlap of course, but it‘s really important that you pay enough attention to the specifics of each of these categories and arrange your interview accordingly.
- Junior Developer Interview Questions
- Mid-level Developer interview questions
- Senior Developer interview questions
Junior Developer Basic Java Interview Questions
A Junior developer is someone that‘s just starting their career with less than 2 years of experience. Junior developers demand the lowest salary, but they are limited in the tasks they can take on, make more frequent mistakes and take more time to solve problems. When hiring a junior, you should consider that they'll need guidance of a more experienced Java professional, who can delegate the tasks and then cross-check the execution done by the junior.
Common Skill Requirements for Junior Java Developers
- Good basic programming skills;
- Foundational Java knowledge;
- Object-Oriented Programming;
- Learning on the job;
- Following instructions and receiving feedback;
- Thinking like a programmer.
Example Java Basic Interview Questions and Answers
Note: Important keywords are underlined in the answers. Bonus points if the candidate mentions them!
Or save yourself time and request a team to match your needs right away.
Question 1: What is inheritance?
Requirement: Object-Oriented Programming
Answer: It's the means by which a new class is derived from an existing class. These new classes can inherit or acquire the properties and methods of other classes. In other words, a subclass takes on the characteristics of its superclass or ancestor. Java does not support multiple inheritances where the parent classes have the same method names.
Question 2: Explain public static void main(String args[]).
Requirement: Foundational Java knowledge
Answer: This is a good basic Java interview question since it's one of the first things you have to memorize when you code in this language.
public: This is an access modifier, which means it specifies who can access this method. It means that this method is accessible by any class. Public members are visible in the same package.
static: This identifies your program as class-based - that is, it can be accessed without first creating the instance of a class. A static block of code is used to initialize the static data members.
void: This defines the method's return type, and it says that the method won't return any value.
main: This is the name of the method. JVM searches for this method as a starting point for an application with only this particular signature.
String args[]: The parameters that can be passed to the main method.
Question 3: What is an object?
Requirement: Object-Oriented Programming
Answer: In Java, an object is an instance of a class. It has a state (which is stored in fields, or variables), and a behavior (stored in methods, or functions).
Question 4: What is object cloning in Java?
Requirement: Foundational Java knowledge, Object-Oriented Programming
Answer: Object cloning means creating an exact copy of an object using the Clone() method. It creates a new instance of the object and initializes all fields to be the same.
Question 5: How would you learn about a new Java class?
Requirement: learning on the job
Answer: Here you are finding out how the candidate approaches learning. You want to see that they have an idea of what information they will need and where to find it. For Java classes, that means looking up tutorials and opening the documentation.
This is a fairly open-ended question. The candidate could talk about their favorite tutorial website or a book they would refer to. They should also mention checking the docs for things like the class member variables, methods, and constructors.
Question 6: Tell me about a time you received feedback on a task.
Requirement: Following instructions and receiving feedback
Answer: This is an open-ended question. The candidate should demonstrate they can accept, understand and act on feedback.
Question 7: Describe how you would approach solving (some problem) on a high level.
Requirement: Thinking like a programmer
Answer: In this question, the problem should be directly related to the work the candidate will actually be doing. You aren‘t looking for a perfect answer. Instead, listen to how the candidate approaches problem-solving, their ability to break a problem down into parts, and if they can anticipate problems well.
Mid-Level Developer Interview Questions
Skip to the Senior developer section for the Java advanced interview questions.
Next, we have interview questions and answers for experienced Java developers. Mid-level developers are the workhorses of the software development world. Whatever Java app or website you‘re using, chances are that most of the code was written, checked, and tested by a mid-level developer.
Typically with 2-10 years of experience, mid-level developers demand a higher salary than juniors. In return, you want developers that can handle most technical tasks on their own and produce excellent code, especially for routine tasks. However, you generally can't expect high-level design skills from them, like designing a new type of application from scratch.
Common Skill Requirements for Mid-Level Java Developers
- Deep knowledge of Java;
- Relevant Java frameworks and toolkits;
- Object-Oriented Programming;
- Data structures;
- Efficient programming and clean code;
- Debugging;
- Testing;
- Breaking a problem into parts.
Example Java Interview Questions and Answers
Note: Important keywords are underlined in the answers. Look out for them in interviews!
Question 8: What are the differences between vectors and arrays?
Requirement: Deep Knowledge of Java, Data structures
Hire expert developers for your next project
1,200 top developers
us since 2016
Answer: In Java, the main difference is that vectors are dynamically allocated. They contain dynamic lists of references to other objects and can hold data from many different data types. This means their size can easily change as needed. Arrays, on the other hand, are static. Arrays group data with a fixed size and a fixed primitive data type.
Question 9: Since Java has a garbage collection feature, does this mean your program can never run out of memory?
Requirement: Deep Knowledge of Java
Answer:
Not necessarily. Although Java does have an automatic garbage collection feature, this won't do you any good if you're creating objects faster than your garbage collector can work. So while garbage collection does mean it's harder for your Java programs to run out of memory, don't push your luck - it can still happen.
Question 10: What is an abstract class? Why are they useful and when would you use one?
Requirement: Object-Oriented Programming
Answer: Abstract classes contain one or more abstract methods (that is, an abstract method that is declared, but contains no implementation). You can't instantiate your abstract classes, so you need subclasses if you want implementations for your abstract methods.
Question 11: What is method overriding in Java?
Requirement: Object-Oriented Programming, Knowledge of Java
Answer: Method overriding is a kind of run-time polymorphism or a dynamic method dispatch. In Java (and any object-oriented language) method overriding allows a sub-class (or derived class/extended class/child class) to provide a different implementation of a Java method from one of its superclasses (or base class/parent class). In Java, you can override a private or static method. To override a method in Java, a subclass method must have the same class name, signature, and return type as the method in its superclass. A final class in Java cannot be inherited or overridden. The super keyword in Java refers to the immediate parent class object. It is used to refer to an immediate parent class method or an immediate parent class instance variable and is used to call the immediate parent class constructor.
Method overriding always requires inheritance. Here's an example:
class Car {
void run(){
System.out.println('car is running');
}
Class Audi extends Car{
void run()
{
System.out.println('Audi is running safely with 100km');
}
public static void main( String args[])
{
Car b=new Audi();
b.run();
}
}
Question 12: What is the finalize()?
Requirement: Efficient programming and clean code, Knowledge of Java
Answer: The finalize() method is called by the Java garbage collector when it determines there are no references to an object and it can be discarded. The finalize method of the Object class doesn't do anything, but you can override it to do clean-up tasks like disposing of system resources or breaking I/O connections.
Question 13: Write a sample unit testing method for testing exception named as IndexOutOfBoundsException when working with ArrayList.
Requirement: Testing, Java JUnit Package
Answer:
@Test(expected=IndexOutOfBoundsException.class)
public void outOfBounds() {
new ArrayList<Object>().get(1);
}
Question 14: Describe a time you fixed a bug in a complex system. How did you approach the problem? What debugging tools did you use? What did you learn from this experience?
Requirement: Debugging, Breaking down a problem into parts
Debugging is one of the key skills for any software developer. However, the real skill is in breaking the problem down practically rather than finding small errors in code snippets. Debugging often takes hours or even days, so you don't have time in an interview setting. Asking these questions will give you an idea of how your candidate approaches errors and bugs.
Answer: In the candidate's response you should look out for things like:
- A measured, scientific approach;
- Breaking down the problem into parts;
- Finding out how to reproduce the error such as a compile-time error;
- Expressing and then testing assumptions;
- Looking at stack traces;
- Getting someone else to help/take a look;
- Searching the internet for others that have had the same problem;
- Writing tests to check if the bug returns;
- Checking the rest of the code for similar errors;
- Turn problems into learning experiences;
Senior Java Developer Interview Questions
Senior developers are the most experienced and skilled and they are experts in the Java programming language. But with the highest salary range, you're looking for much more than that.
A great senior Java developer has mastered the entire software lifecycle, including planning, designing, building, testing, deploying, and maintaining Java applications. You should also be looking for leadership skills and an interest in the business and legal context of whatever they are developing. To do this, you'll need to go further than just asking Java programming interview questions.
Common Skill Requirements for Senior Java Developers
- Expert Java knowledge;
- Object-Oriented Design Patterns;
- High-level design skills;
- Designing for specific requirements (e.g. security, scalability, optimization);
- DevOps;
- Distributed/parallel programming;
- Maintaining and upgrading applications;
- Leadership skills;
- Clear communication skills;
- Mentoring junior and mid-level developers;
- Understanding business and legal context.
Example Java Advanced Interview Questions and Answers
Note: Important keywords are underlined in the answers. Listen out for them!
Question 15: What's the difference between unit, integration, and functional testing?
Requirement: Expert Java knowledge
Answer: A unit test works on an isolated piece of code, usually a method. An integration test tests how your code integrates with other systems, for example, a database or a third-party application. A functional test tests the actual functionality of an application and uses automated tools to carry out and simulate user interactions.
Question 16: What is the difference between a factory and an abstract factory pattern?
Requirement: Object-Oriented Design Patterns
Answer: Abstract Factory provides an extra layer of abstraction. Consider different factories each extended from an Abstract Factory and responsible for the creation of different hierarchies of objects based on the type of factory. E.g. AbstractFactory extended by AutomobileFactory, UserFactory, RoleFactory, etc. Each individual factory would create objects of that specific type.
Question 17: What's the difference between path and classpath variables in Java?
Requirement: Expert Java knowledge
Answer: Both path and classpath are environment variables. However, the operating system uses a path to find the executables (which is why you always need to add the directory location in the path variable any time you install Java or want the OS to be able to find the executable). Classpath, on the other hand, is used by Java executables to locate class files.
Question 18: What's the most important thing to look for or check when reviewing another team member's code?
Requirement: Mentoring junior and mid-level developers, Leadership skills
Answer: Here you're checking for analysis skills, knowledge of mistakes that less experienced developers make, keeping in mind the larger project, and attention to detail.
A good answer might mention code functionality, readability and style conventions, security flaws that could lead to system vulnerabilities, simplicity, regulatory requirements, or resource optimization.
Open-Ended Questions
Once you've established that your senior dev is a Java expert, you should ask some open-ended questions. They should spark a discussion and you should tailor them to fit your own job requirements. Don't be afraid to ask follow-up questions!
Question 19: What tools & practices do you consider necessary for a Continuous Delivery solution?
Requirement: DevOps systems design, Maintain, and upgrade applications
Question 20: You want to synchronize 5 threads to start at the same time. Describe a solution.
Requirement: Parallel/concurrent programming for running multiple threads
Question 21: In a 3-tier application running a Java application server you notice freezes for several seconds during high load. What are the most likely reasons? How would you troubleshoot them?
Requirement: Problem-solving and troubleshooting, Communication skills, Analysis
Hire expert developers for your next project
Question 22: How would you implement a layered application?
Requirement: High-level design
Hiring Java developers: A challenging task
You can certainly make good use of the above-mentioned interview questions, however, is that enough? Well, depending on the local labor market in your geography, hiring competent Java developers can be hard!
You will certainly need Java skills, however, you need more than that. For e.g., you need the following:
- The knowledge of coding best practices;
- Familiarity with application security vulnerabilities;
- The ability to collaborate with your larger team.
Wondering how you can onboard competent Java developers? Our guide “How to find a good software developer” can help!
You also need to implement a robust code review process in your team, don’t you? It will help you catch defects earlier. However, you need very experienced reviewers for this, and they are hard to find! Once again, we at DevTeam.Space can help.
Key Java knowledge areas to focus on during the interview
The above-mentioned interview questions will help, however, you also need to prioritize the key Java knowledge areas. These are as follows:
1. Encapsulation in Java
You need Java programmers with good knowledge of object-oriented programming (OOP). OOP has a few fundamental concepts, and encapsulation is one of them. This concept involves wrapping the data and the code that acts on that data.
Other Java classes can’t access the variables of a class that’s subject to encapsulation. Smart Java programmers need to know this concept well.
2. OOP (Object-Oriented Programming) concepts in Java
Look for Java developers with good knowledge of OOP concepts in Java. They need to understand the following very well:
- Object;
- Class;
- Inheritance;
- Polymorphism;
- Abstraction.
OOP concepts make application development and maintenance easier. However, Java programmers need to understand them well to take the advantage of them.
3. Core Java interview questions
You need Java programmers with experience in developing general-purpose Java applications. This requires core Java skills. Look for Java developers that have a robust knowledge of the following:
- OOP;
- Data types;
- Operators;
- Functions;
- Loops;
- Exception handling.
Java programmers need to have in-depth knowledge of core Java before they can develop advanced Java applications.
4. “Method overloading” in Java
Check whether a candidate understands “method overloading” when you interview Java developers. This feature allows Java to have multiple methods with the same name. However, the list of arguments for these methods needs to be different. Programmers might often need to use this feature.
5. “Catch block” in Java
You need Java developers with sound knowledge of handling exceptions. A “catch block” is an exception handler. It will handle the kind of exception that’s indicated by its argument. Programmers need to write the code to be invoked when the exception handler is executed. They need to write this in the block.
6. “ClassLoader” in Java runtime environment (JRE)
Java programmers that you hire should know about the Java runtime environment (JRE) well. They need to know its various parts. The knowledge of “ClassLoader” becomes important in this context. A “ClassLoader” dynamically loads Java classes in the “Java Virtual Machine” (JVM). The JRE calls a “ClassLoader” when the application needs a class, and that’s why it’s important.
7. The “platform-independent” nature of Java
The platform-independent nature of Java is one of its key advantages, and developers should know how to utilize it. The Java compiler creates “byte code” (that is then compiled into native machine code). It’s the output produced by it during the compile time from the Java code. “Byte code” can run on every operating system. Programmers should know how compilation works in the case of Java.
8. The working of “HashMap” in a Java program
You need Java developers with a good understanding of arrays. Here, the knowledge of a “HashMap” becomes important. Developers access items in an array using an index.
On the other hand, a “HashMap” stores items in “key/value” pairs. While the index for an array needs to be an “integer”, the index for a “HashMap” can be a “string”. Experienced Java programmers should know these powerful features. Developers should know about “Hashtable” too.
9. “Static methods” in Java
Look for knowledge of “static methods” when you interview Java developers. They should know how to use the “static” keyword to create such a static method. These methods exist independently, and they aren’t dependent on any instances for any class. A static method cannot be overridden like an instance method. Such methods don’t use any instance variable of any object of the class for which they are defined. A static variable is not a local variable, and the memory is allocated only once for static variables.
10. Java API (Application Programming Interface)
Java is an immensely powerful language, however, you need coders that know how to use it. They should know about the Java API. It contains all classes that are included in the “Java Development Kit” (JDK). The Java API includes packages, classes, interfaces, methods, fields, constructors, etc. Look for Java programmers that know this well.
11. “Java Development Kit” (JDK)
You should expect Java developers to know sufficiently about JDK (Java Development Kit). Apart from JVM and JRE, Java programmers need in-depth knowledge of JDK. It’s a package for developing Java applications. Coders need to download JDK and access the tools in this package. Different Java editions like “Java Enterprise Edition” (Java EE), “Java Standard Edition” (Java SE), and “Java Mobile Edition” (Java ME) have their individual JDKs.
12. Instance variables in Java
Look for Java developers with knowledge of instance variables. In Java, developers declare these variables in a class. However, they declare them outside a method, constructor, or block. Programmers use the keyword “new” to create instance variables. The destruction of the object brings the destruction of instance variables. An initial value for an object reference defined as an instance variable is null.
13. Local variables in Java
During the interview, you should check whether the candidates understand the concept of local variables in Java. Programmers should declare them within the body of a method. Developers should also understand that only that method can use this variable.
14. Classes like “StringBuilder” and “StringBuffer” in Java
Check whether a Java developer understands the “StringBuilder” class. This represents a mutable sequence of characters, and it’s an alternative to the “String Class”. On the other hand, the “StringBuffer” class creates a string that can be modified. Java developers should know the syntax of using these classes.
15. The “object class” in Java
Look for knowledge of the object class when you interview Java developers. By default, an object class is the parent of all Java classes. An in-depth understanding of the object class helps developers to refer to objects even if they don’t know their type.
16. Other important knowledge areas
You need competent developers with considerable experience in Java application development projects. All Java developers including a fresher need familiarity with the “Java.lang” documentation. Java programmers should also have familiarity with the Java “util” documentation.
Look for skills in the following areas:
- Java classes like “Linked list”, “Singleton class (with only one instance and all the methods and classes belong to this one instance)”, “HashSet”, “Inner Class”, etc.;
- Methods like “overridden”, “hashcode”, “notifyAll”, “class methods”, etc.;
- Objects like “iterator”, “string object”, etc.;
- Serialization and deserialization in Java and their default values;
- Java algorithms like sorting;
- Java expressions like “Boolean”;
- Wrapper classes;
- Checked exceptions;
- Unchecked exceptions;
- The default constructor provided by the Java compiler for object creation with a default value;
- Copy constructor to initialize an object through another object of the same class;
- Parameterized constructor;
- Java iteration statements;
- The “Runnable” interface;
- Using “String args” in Java;
- Synchronization;
- “Thread-safe” classes in Java;
- “TreeSet” in Java;
- The concept of “try block” in programming languages like Java and Python;
- Interfaces like “comparator”, “serializable”, etc., with only abstract methods;
- The “Runtime Exception” super class in Java is called by a reference variable;
- Java “String Pool” and the creation of a new object in the string pool.
In Summary
Hiring the right people for your development team is critical to the success of your project. Remember that you should not be aiming to hire the perfect Java developer, but rather the right person for the job at hand.
With the help of this information and sample interview questions on Java, you can ensure that the hiring process goes smoothly and successfully - allowing you to hire a great programming team to get your project completed on time and on budget.
Happy Hiring!