- Developers
- Developer Blog
- Best Programming Languages
- Why Should You Use Java for Your Backend Infrastructure?
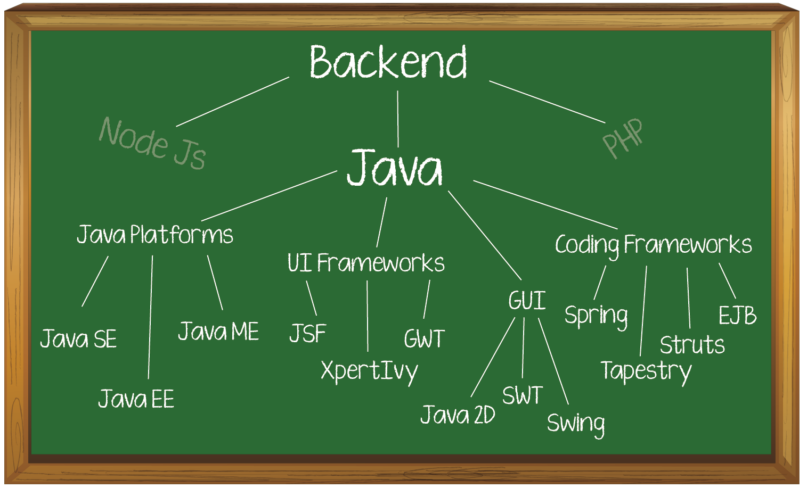
profile

By Aran Davies
Verified Expert
8 years of experience
Aran Davies is a full-stack software development engineer and tech writer with experience in Web and Mobile technologies. He is a tech nomad and has seen it all.
Wondering why you should use the popular programming language Java for your backend infrastructure?
This is an important question if you are planning to build a new web or mobile application.
Benefits of Using Java for Backend Infrastructure
Some of the benefits of Java as backend infrastructure are the following:
Scalability
Java is highly scalable. Take the case of Java EE. Assuming you have done the right planning and used the right kind of application server, Java EE can transparently cluster instances. It also allows multiple instances to serve requests.
In Java, separation concerns allow better scaling. When processing or the number of Input-Output (IO) requests increases, you can easily add resources, and redistribute the load. Separation of concerns makes this transparent to the app.
Java components are easily available, making scaling large web apps easy. The language is flexible, and you need to do less invasive coding to improve scalability. Read more about it in this StackOverflow thread on Java scalability.
Cross-platform usage
One great advantage of Java is “Write Once, Run Everywhere”. We also call this feature ’portability’. You can execute a compiled Java program on all platforms that have a corresponding Java virtual machine (JVM).
This effectively includes all major platforms, e.g. Windows, Mac OS, and Linux. Read more about the cross-platform feature of Java in this StackOverflow thread titled “Is Java cross-platform”.
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
You first write your Java program in the “.java” file. Subsequently, you compile it using the Eclipse IDE or ’javac‘, and thereby you create your “.class” files. While it isn‘t mandatory, you can also bundle your “.class” file into a “.jar” file, i.e. an executable.
You can now distribute your “.jar” file to Windows, Mac OS, and Linux, and run it there. There may be occasional confusion, because you may find different set-up files for different platforms for one Java program. However, these have nothing to do with Java.
There are applications that depend on specific features certain platforms provide. For such apps, you need to bundle your Java “.class” files with libraries specific to that platform.
Powerful memory management
Java’s automatic memory management is a significant advantage. I will describe it briefly here to show how it improves the effectiveness and speed of web or mobile applications.
In programming parlance, we divide memory into two parts, i.e. the ’stack’ and the ’heap’. Generally, the heap has a much larger memory than the stack.
Java allocates stack memory per thread, and we will discuss threads a little later in this article. For the time being, note that a thread can only access its own stack memory and not that of another thread.
The heap stores the actual objects, and the stack variables refer to these. The heap memory is one only in each JVM, therefore it‘s shared between threads. However, the heap itself has a few parts that facilitate garbage collection in Java. The stack and heap sizes depend on the JVM.
Now, we will analyze the different types in which the stack references the heap objects. The different types have different garbage collection criteria. Read more about it in “Java Memory Management”.
The following are the reference types:
- Strong: It‘s the most popular, and it precludes the object in the heap from garbage collection.
- Weak: An object in the heal with a weak reference to it from the stack may not be there in the heap after a garbage collection.
- Soft: An object in the heap with a soft reference to it from the stack will be left alone most of the time. The garbage collection process will touch it only when the app is running low on memory.
- Phantom reference: We use them only when we know for sure that the objects aren‘t there in the heap anymore, and we need to clean them up.
The garbage collection process in Java runs automatically, and it may pause all threads in the app at that time. The process looks at the references that I have explained above and cleans up objects that meet the criteria.
It leaves the other objects alone. This entire process is automated; therefore, the programmers can concentrate on their business logic if they follow the right standards for using reference types.
Multi-threading
Multi-threading allows multiple users to run one application program simultaneously for their individual tasks. However, multi-threading isn‘t a new concept; for example, IBM Customer Information Control System (CICS) has championed it for a long time in the centralized computing environment.
Hire expert developers for your next project
1,200 top developers
us since 2016
However, in the network computing environment, Java was the first programming language to offer this to developers. Users can simultaneously execute multiple tasks using a Java program, which has separate execution paths.
There are several advantages of multi-threading with Java, and they are as follows:
- Responsive servers: Irrespective of the time a process takes the server remains responsive and hence there are fewer issues.
- Quick application response: A Java app processes user inputs quickly irrespective of the number of concurrent users.
- You can run multiple operations simultaneously.
- The app uses cache and CPU resources optimally, thus delivering better performance.
- Lower development time, simplified programming, and reduced application maintenance costs are some of the other advantages.
Note that your testing and debugging processes are more complex with multi-threading. However, the advantages of multi-threading in an enterprise or a mass-usage context vastly outweigh this disadvantage.
Read more about it in “Common Advantages And Disadvantages Of Multithreading In Java”.
A rich ecosystem facilitates backend Java development
I ask you to do a simple survey among programmers. Just ask them whether they have heard of Eclipse IDE, NetBeans, Maven, and Jenkins. The response will tell you how rich the Java ecosystem is!
I list a few important resources among many in the Java ecosystem, which are as follows:
- Eclipse IDE: Easily the most successful open-source IDE, Eclipse integrates with the Java compiler well. It highlights compilation errors, provides excellent facilities to organize your projects, and facilitates Git version control. Although Eclipse needs no introduction, if you need more details, check the Ecplise website.
- Maven: You can manage your software with it, and it‘s really an enterprise build tool. Maven offers you a standardized approach to building, testing, packaging, and deploying your code. It also allows you to document your project well. Check the Apache Maven project website for more details.
- Spring framework: The intention of creating this Java backend framework was to simply enterprise Java development. With over a million users now, it leads in the Java server framework space. It provides many useful modules and has other open-source frameworks like Spring Security and Spring Integration. It helps in a lot of ways like dependency injection, inversion of control, etc. to make the application’s response more flexible. Read the Spring Framework website for more details.
Apart from these, Java backend developer also uses tools like Java database connectivity (JDBC), Hibernate framework, spring boot, Java persistence API, cache server API, etc. for efficient server-side development.
I have touched upon only a few prominent tools and frameworks in the Java ecosystem for web and mobile application development. You can study more about it in the series of articles starting with “A Walk Through the Java Ecosystem”.
Java backend security
If you are a senior executive or a development manager in an Enterprise IT organization, this would be your first reason to use Java as a backend programming language. Java scores better than all other programming languages in making your app secure, due to the following:
- The security model isolates Java programs from potentially malicious programs that your users might download from unknown sources.
- Java doesn‘t use pointers, hence unauthorized access to memory blocks isn‘t possible.
- With the Java exception handling concept, you can handle a series of errors in one go, which reduces the risk of system failure.
- Different JVMs won’t execute code in different orders since Java defines all primitives with their predefined size. Java also sets the order of execution for operations.
- JVMs test Java bytecodes every time for viruses, etc. before execution.
- Java allows developers to use tested reusable code, which reduces risks.
- The language has an access-control mechanism to prevent unauthorized access requests from untested code.
- Java allows developers to declare classes as “Final”, which no one can override. This is another guarantee against hackers.
Read more about security mechanisms in Java in “Java application security features and measures”.
Availability of skilled manpower
Irrespective of your location, you will likely find Java developers very easily. This reduces your staffing risks for development and maintenance. The global availability of Java skills also allows you to outsource if you need to.
Should you use Java backend infrastructure for your startup?
According to a study, the mobile app market” is projected to reach $3,798 million by 2025, growing at a CAGR of 19.5% from 2018 to 2025“.
Getting your development right is the most important thing you can do if you want your product to be a hit with consumers.
Hire expert developers for your next project
If you are in a startup environment, some of your developers might want a trendier language like Ruby on Rails. I recommend that you approach such debates in a dispassionate manner keeping in mind that although developed in the 1990s, Java continues to evolve.
Also, consider the overwhelming evidence of the popularity that Java enjoys as a server-side programming language. It is among the top 3 programming languages according to the ’TIOBE programming community index‘ and ’IEEE Language Rankings‘.
Java ranks 3rd in GitHub, 4th in ’Stack Overflow‘, and 3rd in ’RedMonk‘ Programming language rankings. In each of these cases, Java closely follows JavaScript.
In addition to the advantages of Java backend development I have listed, these statistics are sufficient for you to trust Java for your start-ups, over other modern languages. Read more about the statistics in “DZone‘s Guide to The Java Ecosystem”.
Having a team of skilled and competent software developers is as important as selecting the right programing language and development tools for your next software development project.
Your software development team will ensure that your software application survives in the competitive market. For this, partner with a reputed software development agency that can help you in outsourcing field-expert software engineers for your project.
DevTeam.Space is one such platform with a community of experienced software developers who are vetted for their expertise and are also managed by a dedicated technical manager for delivering high-quality work.
You can easily partner with these software developers by sending us your initial project specifications via this quick form. One of our account managers will get back to you for further assistance.
Frequently Asked Questions on Java Backend
Java is an object-oriented programming language that is one of the main coding languages used in software development today. It was released back in 1995, also making it one of the oldest popular programming languages, which continues to receive regular updates.
Java is used all over the world and is the backbone of many leading mobile and web applications. It is a well-defined language that is known to most of the world’s programmers for mobile and web development.
There are lots of alternative languages to Java. Some of the most popular include Kotlin, Python, Javascript, Haskell, etc. All of these have their pros and cons. Read this article to find out more about Java backend development.
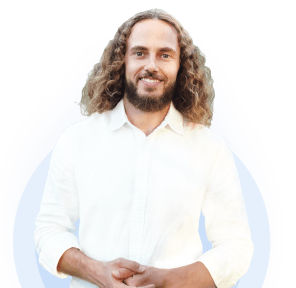
Alexey Semeney
Founder of DevTeam.Space
Hire Alexey and His Team To Build a Great Product
Alexey is the founder of DevTeam.Space. He is award nominee among TOP 26 mentors of FI's 'Global Startup Mentor Awards'.
Alexey is Expert Startup Review Panel member and advices the oldest angel investment group in Silicon Valley on products investment deals.