- Developers
- Hiring Interview Tips
- 34 PHP Interview Questions and Answers for 2024
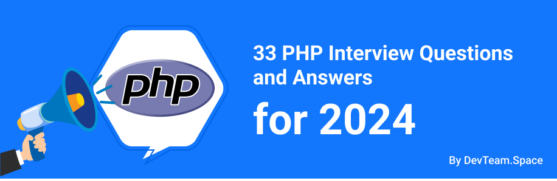
profile

By Aran Davies
Verified Expert
8 years of experience
Aran Davies is a full-stack software development engineer and tech writer with experience in Web and Mobile technologies. He is a tech nomad and has seen it all.
Carefully analyze your job requirements first
Create an appealing job posting first, which includes the following:
Company descriptions
Introduce your company with facts, yet make the introduction appealing. Explain the growth opportunities you offer. Provide information about skill development opportunities, organizational culture, and work environment. Finally, explain your compensation and benefits policies.
Job descriptions for PHP developers
Describe the job requirements when you hire PHP developers. Explain whether you have both development and maintenance projects. Talk about how these projects help you to meet your strategic objectives and explain how the contribution of the new developers will help you.
Roles and responsibilities of a PHP developer
You need a PHP developer to carry out the following job responsibilities:
- Studying the functional and non-functional requirements;
- Understanding the technical solutions;
- Assisting the testing and DevOps teams to establish an effective “Continuous Integration” (CI)/”Continuous Delivery” (CD) environment;
- Create specifications for new web development projects;
- Coding and unit testing new web applications;
- Working with the testing and DevOps teams for effective testing and deployment;
- Maintaining existing web applications;
- Communicating effectively and reporting the project status;
- Participating in continuous improvement projects.
Skills and competencies that you need in a PHP developer
You need a PHP developer to have a bachelor’s degree in computer science or related fields. Look for the following skills:
- Good knowledge of PHP and the key features of this programming language;
- Knowledge of PHP syntax;
- Sufficient familiarity with frameworks like Laravel;
- Experience with a server-side scripting language;
- Understanding of front-end technologies like JavaScript, HTML, CSS, etc.;
- Familiarity with the concepts of programming languages like Perl;
- Sufficient knowledge of operating systems like Windows, Linux, Unix, etc.;
- Familiarity with popular design patterns like MVC (Model View Controller);
- Understanding of “Object-Oriented Programming System” (OOPS);
- Experience with popular SQL databases like MySQL, PostgreSQL, etc., that PHP supports;
- Familiarity with well-known NoSQL databases like MongoDB, Cassandra, etc.;
- Knowledge of integrating multiple data sources and databases into a PHP application;
- Knowledge of static and dynamic websites;
- Experience in developing scalable and performant web apps;
- Understanding of the limitations of PHP;
- Familiarity with tools like Git;
- Experience in developing RESTful APIs;
- Knowledge of writing maintainable PHP code.
You should expect a PHP programmer to have the following competencies:
- Problem-solving skills;
- Passion for excellence;
- Commitment;
- Communication skills;
- Teamwork;
- The ability to see the bigger picture.
PHP interview questions for junior developers
Use the following questions when you interview junior PHP programmers:
Question 1: How does “$message” differ from “$$message” in PHP?
Answer: In PHP, the “$message” has a fixed name and value. It’s a regular PHP variable. On the other hand, the “$$message” is a reference variable. It holds data about the variable in question. The value in the “$$message” changes when the value in the main variable changes.
Question 2: Mention the various data types in PHP.
Answer: PHP had the following data types:
- “String”: This is a set of characters.
- “Float”: This is a floating point, i.e., a decimal number.
- “Integer”: As the name suggests, this is an integer.
- “Boolean”: This can hold one of the following two states, namely, “True”, and “False”.
- “Object”: This can store values of different data types into one entity, e.g., “Audi = new Car()”.
- “Array”: This can store multiple values of the same types, e.g., “array(“Audi”, “Mercedes”, “Lexus”).
- “NULL”: If a program doesn’t assign a value to a variable, then “NULL” can be assigned to it.
Question 3: How can you get the “Boolean” value of a variable in PHP?
Answer: A PHP programmer needs to use the “boolval” function for this. Consider the following code snippet:
boolval ( mixed $variable ) : bool
This code returns the “bool” value of “Variable”. It converts the scalar value to a “bool”.
Question 4: How does the “isset()” function work in PHP?
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
Answer: The functionality of the “isset()” function is to check whether a variable is set and has a non-NULL value. This function returns a Boolean. It returns a “False” if the variable is not set, and it returns a “True” if the variable is set.
The “isset()” function is used to check multiple variables. The code will look like the following in that case:
Isset(Variable1, Variable2, Variable3)
Question 5: Mention the various array functions in PHP.
Answer: Developers can use many PHP array functions. A few prominent ones are as follows:
- “array()”: This function creates an array.
- “array_diff()”: It compares different arrays. This function returns the differences in the values.
- “array_keys()”: This function returns the keys in an array.
- “array_search()”: Programmers can use this function to search for a value in an array, and this function returns the key for that value.
- “array_slice()”: This function returns a specific part of an array.
- “array_sum()”: PHP developers can use this function to sum all the values in an array.
- “count()”: As the name suggests, this function counts the number of elements in a PHP array.
Question 6: How do indexed arrays differ from associative arrays in PHP?
Answer: The differences between indexed and associative arrays in PHP are as follows:
- Indexed arrays have their keys or indexes. However, each key in an associative array has its value.
- PHP automatically assigns value to indexes in an indexed array. An index of an array starts from “0”. Associative arrays need the manual assignment of keys, and these keys can hold even strings.
Question 7: What is the syntax to code a PHP script?
Answer: PHP developers can code a PHP script anywhere within a document. They need to start a PHP script with “”. It looks like the following code snippet:
<?php
//Code your required PHP script here
?>
Question 8: What is the syntax to create a PHP constructor?
Answer: PHP programmers can use “constructors” to initialize the properties of an object after creating the object. These are special methods offered by PHP.
They can use the “__construct()” function for this. PHP automatically calls this function when an object is created from a class. Programmers need to start this function with two underscores, i.e., “__”.
The following code snippet provides an example of it:
<?php
class Car {
public $name;
public $color;
function __construct($name) {
$this->name = $name;
}
function get_name() {
return $this->name;
}
}
$Marcedes = new Car(“Marcedes”);
echo $Marcedes->get_name();
?>
Question 9: What is the syntax to create a PHP destructor?
Answer: While PHP developers can use a “constructor” to create an object, they can use a “destructor” to destroy it. Like a “constructor”, a “destructor” is a special method. Programmers need to start a “destructor” with two underscores, i.e., “__”.
The following code snippet provides an example:
<?php
class Car {
function __construct() {
//Initialize the object properties
}
function __destruct($name) {
//clears the object reference
}
}
?>
Question 10: How does a “require” in PHP differ from “include”?
Answer: PHP offers constructs like “require” and “include”. Web developers using PHP can call them without using a parenthesis, e.g. “include thisfile.php”.
The “include” construct issues a warning if the file to be included isn’t found. The PHP script will continue to run.
However, “require” behaves differently. If the program doesn’t find the file, then “require” will issue a fatal error. It will also stop the PHP script.
Programmers should use the “require” construct if the file is crucial for the script. In this way, they will get a fatal error as soon as the program detects the absence of the file. Developers can then resolve the underlying problem that resulted in a missing file.
Question 11: Provide a few examples of popular PHP string functions.
Answer: PHP offers many string functions, and a few popular ones are as follows:
- “echo()”: This function writes one or more strings in the output.
- “explode()”: Programmers can use it to break a string variable into an array.
- “Itrim()”: This function eliminates the extra characters or blank spaces from the left side of a string.
- “parse_str()”: Developers can use this function to parse a query string into PHP variables.
- “str_replaced()”: This function replaces the characters of a string that the program asks for.
- “str_split()”: Programmers can use this function split strings into arrays containing characters.
- “strlen()”: This function calculates the length of a string.
Question 12: What does “PEAR” stand for in PHP?
Answer: “PEAR” is the acronym for “PHP Extension and Application Repository”. PHP developers can utilize reusable components when developing an app. They can use PEAR, a framework for distribution systems for such reusable PHP components.
PEAR offers various kinds of PHP code snippets, furthermore, it includes useful libraries. Developers can also use the PHP “Command Line Interface” (CLI) offered by PEAR to install various “PHP packages” easily.
Question 13: Explain whether PHP is a case-sensitive programming language.
Answer: PHP is case-sensitive but not fully. Variable names in PHP are case-sensitive. However, PHP function names aren’t case-sensitive. Programmers can define a function name using lowercase, and they can call these functions using uppercase. The other aspects of PHP are case-sensitive.
Question 14: What are the various types of errors in PHP?
Answer: A PHP program can have the following 4 types of errors:
- Warning errors: These errors don’t stop the execution of a program, however, they provide warning messages. Programmers should investigate them to prevent bigger errors in the future.
- Notice errors: These are minor errors, and they don’t stop the execution of a PHP script. Developers should analyze these errors to fix the underlying issues.
- Parse errors: These errors occur due to syntax-related issues. Missing or misused symbols can cause these errors, and the compiler catches them.
- Fatal errors: These are critical errors that crash a PHP program. Undefined classes or functions often result in such errors. Fatal errors in PHP are of 3 types, namely, “start-up fatal errors”, “compile-time fatal errors”, and “runtime fatal errors”.
Question 15: How to define a PHP class?
Answer: A PHP developer uses the “class” keyword to define a PHP class. The following code snippet has an example:
Hire expert developers for your next project
1,200 top developers
us since 2016
<?php
class Car {
//provide the relevant code here
}
?>
Question 16: What is the utility of the PHP “tmp” directory during the file-uploading process?
Answer: PHP uses a directory named “upload_tmp_directory” in php.ini. All temporary files are stored here during the file upload process. It’s temporary, and it needs to be writable by the user running PHP. PHP uses the default folder of the system if this directory isn’t specified.
Questions and answers for interviewing a mid-level PHP developer
Use the following questions when you interview mid-level PHP developers:
Question 17: What do the “GET” and “POST” methods in PHP do?
Answer: The “GET” method in PHP sends the encoded user information, and it appends to the page request. PHP separates the page and the encoded information using the “?” character. The following code snippet provides an example:
http://www.samplePHPcode.com/index.htm?car1=value1&car2=value2
On the other hand, the “POST” method transfers information via HTTP headers. Binary data is also allowed. PHP encodes this information as it does for the “GET” method. It puts this encoded information into a header called “QUERY_STRING”.
Question 18: What does the “require_once” expression do in PHP?
Answer: PHP developers use constructs like “include” and “require” to include files. The “require_once” expression has some similarities with “require”. However, this expression checks whether the specified file is already included. It doesn’t include the file if that’s already included.
Question 19: What does the “Unlink” function in PHP do?
Answer: Programmers can delete the “unlink()” function to delete a file in PHP. This function accepts only the file name as the argument. The following code snippet is an example:
bool unlink (string $filename)
Here, $filename is the name of the file that you want to delete. PHP returns a Boolean value of “TRUE” if the file is deleted. It returns a “FALSE” if the file isn’t deleted, furthermore, it creates an error at the “E_WARNING” level.
Question 20: What does the “setcookie” function do in PHP?
Answer: PHP developers can use a “PHP cookie”, a small piece of information. A PHP program stores it in the browser of a user. It uses this cookie to recognize a particular user.
A PHP program creates a cookie on the server side, subsequently, it stores it in the browser of a client. Each request from the client to the server embeds the cookie. This allows the PHP program to receive the cookie on the server side.
Programmers can use the PHP “setcookie()” function to set the cookie with an HTTP response. After that, the program can access the cookie using the “$_COOKIE” variable. This is a PHP “superglobal” variable.
Question 21: What would you use for parsing XML in PHP?
Answer: PHP offers an extension named “SimpleXML” for parsing XML. This enables PHP developers to manipulate and get XML data easily. SimpleXML, a tree-based PHP parser enables a program to get the name of an element easily. It allows programs to get the attributes and textual content too.
This extension converts an XML document into a data structure. A program can iterate through this data structure as it would do for a set of arrays and objects. The PHP core includes the SimpleXML functions since PHP 5, therefore, developers don’t need to install anything.
Question 22: How to reset a variable in PHP?
Answer: PHP offers a function called “unset” to reset the value of a variable. A program can call this “unset()” function from user-defined functions. In this case, this function resets a local variable.
Developers planning to “unset” the value of a global variable need to use the “$GLOBALS” array. The “unset()” function in PHP doesn’t return any value.
Question 23: Provide a few examples of PHP numeric functions.
Answer: PHP offers a wide range of numeric functions. They include arithmetic, logarithmic, and trigonometric manipulation functions. The following are a few examples of PHP numeric functions:
- “ceil()”: This function rounds a number up.
- “floor()”: A program can round a number down by using this function.
- “abs()”: This PHP function finds the absolute value of a number.
- “pow()”: This function raises one number to the power of another number.
- “rand()”: A program can generate a random number using this function.
Question 24: How to start a new session or resume an existing session in PHP?
Answer: PHP offers a function named “session_start()”. This function can create a session. Alternatively, this function can resume a current session.
A PHP program needs to pass a session identifier to the “session_start()” function to resume a current session. The program can use a GET or POST request for this, alternatively, it can use a cookie.
The “session_start()” function returns a Boolean value of “TRUE” if it starts a session successfully. It returns the “FALSE” value in the case of a failure.
Question 25: Provide examples of comparison operators in PHP.
Answer: Comparison operators in PHP enable you to compare two different values. The following are a few examples:
- “Equal”: This compares if two values are equal after type juggling. The syntax for this is “$a == $b”.
- “Identical”: This operator compares if two values of the same type are also equal, and the syntax is “$a === $b”.
- “Less than”: This operator determines if one value is less than the other. The syntax is “$a < $b”.
PHP operators compare numerically if both operands are “numeric strings”. If one operand is a number and the other is a numeric string, the comparison is numerical.
Hire expert developers for your next project
Senior PHP developer interview questions
Hiring senior PHP developers? Use the following interview questions:
Question 26: How can you increase the execution time of a PHP script?
Answer: PHP sets the default execution time for a PHP script to 30 seconds. It stops PHP scripts that run longer than that, and PHP returns an error. However, PHP developers can change this.
They need to update the “php.ini” file for this. PHP developers need to change the “max_execution_time” directive in this file, which changes the time allowed for running a PHP script file.
PHP programmers can set the function called “set_time_limit” to “0sec”. This allows unlimited execution time for a PHP script.
Question 27: Explain the importance of the PHP MySQLi functions with a few examples.
Answer: PHP MySQLi functions enable PHP programmers to access a MySQL server in a PHP program. Developers need to use the MySQLi extension for this.
PHP provides installation guides for this extension. These guides cover operating systems like Linux and Windows, furthermore, they cover relevant PHP versions. PHP also provides runtime configuration guides for the MySQLi extension. Programmers need to compile their PHP programs with the support of this extension.
The following are a few examples of PHP MySQLi functions:
- “affected_rows()”: This function returns the number of rows affected due to the MySQL database operation or an SQL query executed previously.
- “autocommit()”: This function can turn on the auto-commit feature for database modifications, furthermore, it can turn it off.
- “begin_transaction()”: This function starts a transaction involving MySQL.
- “change_user()”: A program changes the user of a database connection that’s specified in this function.
- “commit()”: This PHP MySQLi function commits the current transaction.
Question 28: How to use PHP and Node.js on the same server?
Answer: Some projects might need to use both PHP and Node.js. Developers can use them on the same server. However, PHP and Node.js can’t “listen” to the same port. By default, HTTP runs on “port 80”. Only one process can “listen” on one port at a time, which causes the above-mentioned issue.
To solve this challenge, programmers need to run them on different ports. They can then run Node.js on a different port, e.g., “port 8081”. That can create another challenge since users restricted to “port 80” can’t access the Node.js app.
Developers should use a web server like Nginx to solve this. They can configure Nginx to proxy all the Node.js requests through to “localhost:8081”. Nginx has the right capabilities to execute such requests securely.
Question 29: How to loop through each key/value pair in a PHP array?
Answer: PHP developers can use the “PHP foreach loop” to loop through each key/value pair in an array. The “foreach loop” works on arrays only. Programmers use the following syntax to code it:
foreach ($array as $value) {
//write the code block that needs to be executed
}
This assigns the value of the current array element to “$value”, and it moves the array pointer by 1. This process continues for each loop iteration until the last element of the array.
Question 30: Explain the PHP file upload process steps at a high level.
Answer: PHP programmers do the following to upload files to servers:
- Configure the ini file.
- Create an HTML form that allows users to choose the file to upload.
- Create a PHP script to upload files.
- Check the uploaded files to determine if the file already exists. Use appropriate PHP functions for this.
- Set a limit for the file size.
- Specify the file types you would allow users to upload.
- Complete the script to upload a file and execute it.
Question 31: Provide a few examples of built-in algorithms in PHP.
Answer: The following are examples of built-in PHP algorithms:
- “bin2hex”: This algorithm takes a binary string as a parameter, and converts it to a hexadecimal string.
- “bindec”: This algorithm converts a binary string to a decimal number.
- “decbin”: This algorithm converts decimal numbers to binary.
Question 32: Write the code necessary to calculate the total number of days between two dates.
Answer: The following code will calculate the total number of days between two dates:
<?php
$dateM = “2021-02-01”; # date M
$dateN = “2021-02-05”; # date N
$days = (strtotime($dateM) –strtotime($dateN))/(60*60*24);
Echo $days;
?>
Question 33: What are the differences between the “echo” and “print” statements in PHP?
Answer: Both “echo” and “print” statements produce outputs in PHP. Their differences are as follows:
- The “echo” statement can accept multiple parameters, however, the “print” statement accepts only one argument.
- The “echo” statement doesn’t have a return value, however, the “print” statement returns a value of 1.
- The “echo” statement works faster than the “print” statement.
Question 34: Explain “escaping to PHP”?
Answer: The mechanism that the PHP parsing engine follows to differentiate PHP code from other page elements is called escaping to PHP.
Summary
We hope that you can conduct effective interviews with the help of these PHP interview questions and answers. Please contact us at DevTeam.Space if you need more help hiring competent developers.