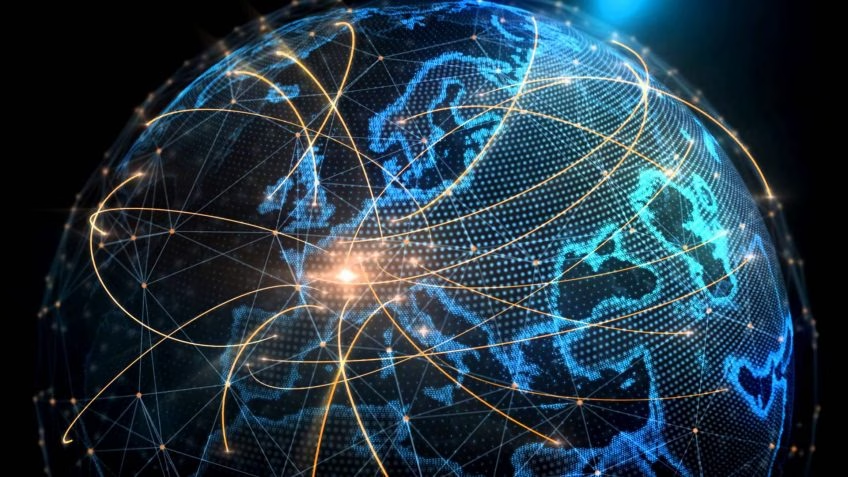
How to Build a REST API for Mobile App?
Want to know how to build a REST API for your mobile app? You've come to the right place. Read our detailed step-by-step guide for developing RESTful APIs for mobile apps.
In this article
- Skills to create a RESTful API for mobile app
- Tools to consider when you build your API for mobile apps
- Hosting decision for your mobile app
- Data security in your RESTful API for mobile app
- Manage environments for your API for mobile app
- Get a robust database solution to develop your API
- Design of URL paths for the RESTful API for mobile app
- Frequently Asked Questions on Rest API for mobile app
In this article, we will explore how to build Rest API (application programming interface) for a mobile app. We will start by looking at the skills required for developing Rest API development. Let's start.
Skills to create a RESTful API for mobile app
I assume you have already assembled a team for your mobile frontend, including UI/UX skills. You have likely onboarded testers too, hence I am only listing skills for RESTful API development here.
You can use any of the below programming languages to develop it:
- C#, VB.Net: You should use the ASP.Net framework;
- Java: The famous Spring framework is just fine;
- PHP: I recommend that you use the Slim framework;
- Python: Flask framework is good enough;
- Ruby: You can use the well-known Sinatra framework;
- js: Using any one of the Express or Strongloop frameworks would be fine.
I also recommend “Learn REST API Design” for your team as a good learning resource.
Tools to consider when you build your API for mobile apps
I recommend that you use the right tools when you build APIs to provide web services for mobile apps. You should consider using a good project tracking tool. Also, consider a good testing framework and a helpful API documentation tool.
Following are my recommendations:
- Trello: It's a popular SaaS product for project tracking and collaboration, and you only need to visit the Trello website to see why! Remember that your REST API development project is one of the several components of your overall mobile app development. Frontend developers and testers will have dependencies on the API development workstream. Transparency about the project status is important, and so is the ability to collaborate. Equip your team well!
- Your choice of the testing framework depends on your choice of programming language. I provide a few examples, as follows:
- If you are coding in Java, ’REST-assured‘ is a good option. It‘s easy to integrate with Java-based automation frameworks.
- Postman is a complete API development tool, which you can use at every stage of your API development workstream, including testing.
- Chakram is another REST API testing framework that runs on Node.js. An advantage of it is that you can write very clear and comprehensive tests.
- APIs are far more important than most people imagine because they allow you to monetize the information you hold over a sustained period. However, successful APIs need excellent documentation so that mobile app developers find it easy to use them. You need to document all error codes, and messages for successful and failed calls very clearly, and for this, you need a good tool. I recommend Swagger, it supports many languages.
Hosting decision for your mobile app
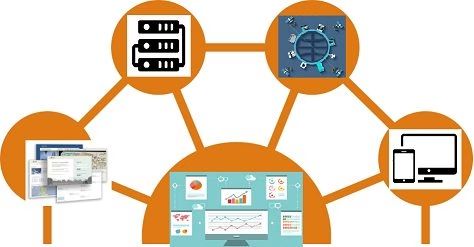
It‘s not just about the RESTful API you are building, but a good hosting solution is imperative for your mobile application. You have a wide range of options, as follows:
- Amazon Web Services: Amazon's expertise with Cloud computing doesn‘t need an introduction. They provide a free tier; however, you may need to upgrade it depending on your app.
- Google Compute Engine: This is Google's ’Infrastructure as a Service‘ (IaaS). Highly reliable, performant, and scalable, this also allows you to create and run your virtual machines.
- IBM Cloud: Highly competitive on all parameters like network, bandwidth, management, and customer support, IBM Cloud offers you both bare metal and virtual server options.
- Digital Ocean: With this IaaS provider, you get good processes, methods, and tools (PM&T) for scaling, management, security, etc.
- Rackspace: Gartner Magic Quadrant report for 2018 lists Rackspace as a leader among Public Cloud Infrastructure Managed service providers.
Read a detailed comparison of your hosting options in “Where to Host Mobile app Backend?”.
Data security in your RESTful API for mobile app
Securing users' data is your responsibility. Make no mistake, your users will not take kindly to any breach of their data security. If you need proof, compare Facebook stock price between 25th July and 26th July! The 20% drop is directly attributed to the Facebook Cambridge Analytics data privacy scandal.
Basic HTTP requests are simply not enough. Well, HTTPS is certainly better, and the hosting providers I listed above will all enable you to use that. However, it may not be enough.
Most mobile applications ask for user permission for certain access, and your multiple APIs will most likely need to do that as well. While the security improves with HTTPS, it doesn‘t enable your app APIs (application programming interface) to ask for user permission.
You need OAuth 2.0 for that. It‘s a reputed authentication framework, and version 2 has significant improvement and simplification over OAuth 1.0. You need HTTPS as a precondition, for communication between a client and an authorization server because of sensitive data.
Read more about OAuth 2.0 in “The Simplest Guide To OAuth 2.0”.
Please pay special attention to how you store your users‘ passwords. You should strongly consider encryption of it, and use well-established password-encryption approaches, e.g. ’salted password hashing‘. Read more about it in “Salted Password Hashing — Doing it Right”.
Manage environments for your API for mobile app
You need to plan for three environments when you develop your API, as follows:
- Development: This is where you will do all your changes and bug fixes. It's okay to use test data generated by your team in this environment. However, consider automating the test data population with scripts. You need to mirror your entire workflow so that you can do complete integration testing, even if with less data.
- Staging: Once your testers have approved the test results in the development environment, the code needs to move to the staging environment. Here you need to keep data as close as possible to the production environment with a similar volume. However, you need to mask sensitive data. I recommend using ’HushHush‘, which is a good data masking tool. It works with the most prominent databases like Oracle, DB2, MySQL, and PostgreSQL. Read more about this tool on the ’Mask-me‘ website.
- Production: I recommend that you build a team to manage your production environment according to the ITIL standards. Get professional help if you need to.
Get a robust database solution to develop your API
The kind of database you use depends on the design of your apps. Explore the following options:
- MySQL: It‘s a very popular relational database management system (RDBMS). It deals with structured data and it‘s very stable. There is a rich ecosystem of tools and frameworks, however, scaling requires in-depth knowledge.
- MariaDB: It‘s another open-source RDBMS, with many similarities with MySQL. There are many libraries, and your development team will find them easy to use.
- MongoDB: This is a non-relational database, hence there are no tables. While you can scale it easier than an RDBMS, your team should be knowledgeable enough to design the database well.
- PostgreSQL: It‘s an Object-Relational database, and it‘s becoming increasingly popular. There is a vibrant ecosystem, learning opportunities are plentiful, and the database is robust and reliable.
Readiness to support multiple platforms
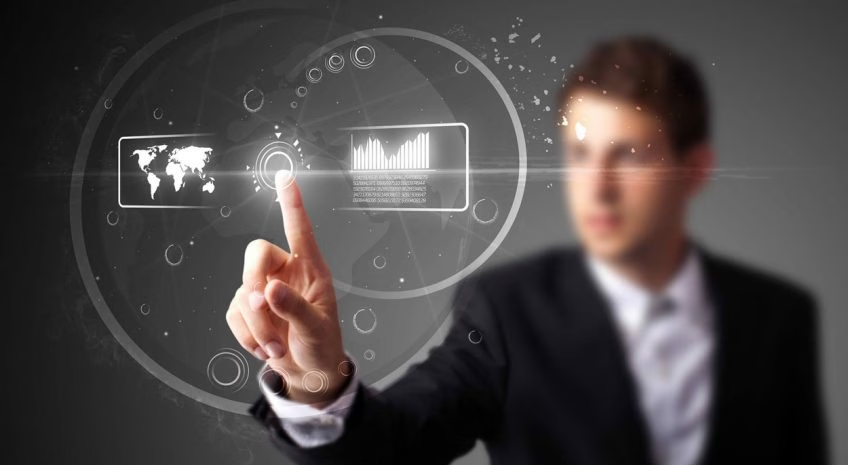
The RESTful API you are building should be flexible and support multiple platforms like Android and iOS. You should minimize the amount of processing in the client, and let the server do the bulk of the data processing, like sorting, filtering, etc.
Also, design your request header template in such a way that it captures mobile device names, device types, and OS versions. This will help you a lot in future debugging since you can get this information in the logs.
Design of URL paths for the RESTful API for mobile app
Your API URL endpoints should clearly indicate what the request is about. This will help you in maintaining your API in the future. For this, you need to effectively use verbs and nouns.
Verbs are actions like GET, POST, PUT, DELETE, etc. Use them to instruct the server about the action it should take. Also, the URL should contain the nouns, which correspond to the resource types.
Check out this guide "Creating a REST Web Service With Java and Spring (Part 1)” for more details.
Design effective rules for requests and responses
You need to follow certain best practices when setting up rules for requests and responses. I cite a few such rules; however, this isn‘t an exhaustive list:
- Let the client send the full object in the request but design your server-side logic to handle a request even if it has fewer data values. Don't assume "null" for missing values, instead you need to have code to identify null.
- Design your status codes with consistency and clarity. Create an effective naming convention.
- Be consistent with date and time formats, use ISO 8601 format and UTC values. The client should show the date and time value to the user according to the user's time zone, and the server shouldn't calculate it.
A RESTful API for your mobile app is a significant investment on your part. You are developing it on your own because you have valuable information that you can monetize. Otherwise, you would have probably used a suitable one from many APIs already available.
Now that you have decided to develop it on your own, plan the project well, and execute the plan with diligence. Get professional help if you need to.
Planning to develop a Rest API for your mobile app?
Rest mobile APIs are now important development tools as they add flexibility to the business processes. One functionality can be used by multiple programs. They support the easy scalability of software services too.
If you, as a business CEO or CTO, are planning to build Rest APIs for your mobile or web applications that contribute towards the growth of your business in the market, you are making a sound technical and business decision.
For this project to complete successfully, no doubt, you will need professional API developers who know different API architectures, their implementations, etc.
If you do not find such technical expertise on your project team, DevTeam.Space can help you with its expert software developers community. Write to us your initial rest API development requirements via this form and we will see how to best help you out.
Frequently Asked Questions on Rest API for mobile app
REST or Representational State Transfer is a type of API that is designed to work on all existing protocols. As such, it is a very popular type of API.
REST APIs are created on HTTP and adherer to standard HTTP verbs and include uniform interfaces.
If you have never written a REST API before then you should read this article to find out exactly how to do it. If you are still not confident by the end then you should onboard a professional developer to do it for you.