- Developers
- Hiring Interview Tips
- ReactJS Interview Questions and Answers for 2024
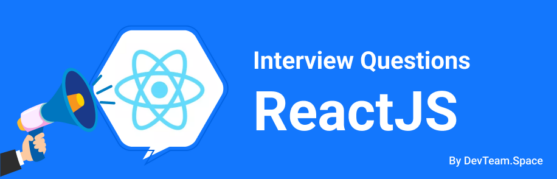
profile

By Aran Davies
Verified Expert
8 years of experience
Aran Davies is a full-stack software development engineer and tech writer with experience in Web and Mobile technologies. He is a tech nomad and has seen it all.
Whether you’re an entrepreneur, a project manager in a large enterprise, or a CTO, you are well aware that the success of your project rests on your ability to find top developers.
In this guide, you‘ll find example ReactJS interview questions and answers you can refer to when seeking a new ReactJS developer to make your dynamic user interfaces come to life. You‘ll also get some practical advice on how to use these questions to reliably identify the best developers for the job.
First Things First: Select Your Job Requirements
ReactJS is a flexible JavaScript library that can be used in different kinds of applications and alongside other web technologies. To find a ReactJS that’s a great fit for your project, you need to get clear on what your job requirements are.
Think about the type of application you are developing, and what technical skills are critical to its success. You also need to think of the softer skills that a candidate needs to work well with the rest of your team.
Some example ReactJS job requirements include:
- Essential web development skills – E.g. HTML, Javascript
- ReactJS-specific skills – E.g. JSX
- Library/toolkit experience – E.g. Redux/MobX
- Design skills – Building scalable applications, using the Flux architecture
- Communication skills – Discussing problems and constraints with your clients
- Being a self-starter – If you don‘t want to do any hand-holding
Avoid making a laundry list of non-essential skills for your perfect ReactJS developer. Instead, focus on what your candidate will actually be doing day-to-day. Keep your requirements list as short as possible. Cut anything they can do without or learn on the job.
With clearly stated requirements, you‘ll be able to choose the right ReactJS coding interview questions and have a much better idea of what kinds of responses you are looking for.
In this guide, we categorize the questions based on the level of expertise you‘re looking for. Junior Developer, Mid-level Developer, and Senior Developer. If you‘re unsure exactly which one you need, take a look at the different sections for a summary of what you can expect from each.
- Junior Developer interview questions
- Mid-level Developer interview questions
- Senior Developer interview questions
ReactJS Junior Interview Questions
Junior developers have the least experience and demand the lowest salary. It‘s important to note before hiring a junior that they need to work under the guidance of more experienced developers.
Skill Requirements for Junior ReactJS Developers
- Basic HTML, CSS, and Javascript skills
- Foundational ReactJS knowledge
- Learning on the job
- Following instructions and receiving feedback
- Thinking like a programmer
Example Basic React Interview Questions and Answers
Note: Important keywords are underlined in the answers. Bonus points if the candidate mentions them!
Or save yourself time and request a team to match your needs right away.
Question 1: Write an example of a simple HTML document with some header information and some page content.
Requirement: Basic HTML skills
Answer: HTML documents are different, but they follow a basic structure of head and body. The different sections are marked with tags such as DOCTYPE, html, head, title, meta, body, h1, p.
For example:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<meta charset="UTF-8">
<meta name="description" content="Page description">
</head>
<body>
<h1>Interview Example Web Page</h1>
<p>Some content goes here</p>
</body>
</html>
Question 2: What is the purpose of the following code?
Requirement: Using CSS with React
render() {
let className = 'menu';
if (this.props.isActive) {
className += ' menu-active';
}
return <span className={className}>Menu</span>
}
Answer: This code adds a CSS class to a component by passing a string as the className prop. Here the class depends on the component props or state which is common with ReactJS.
Question 3: Explain what a Javascript callback function is and provide a simple example.
Requirement: Basic Javascript skills
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
Answer: A callback function is a function that is called after another function has finished executing. A callback function is passed to another function as an argument and is invoked after some operation has been completed. For example:
function modifyArray(arr, callback) {
arr.push(100);
callback();
}
var arr = [1, 2, 3, 4, 5];
modifyArray(arr, function() {
console.log("array has been modified", arr);
});
Question 4: Briefly describe ReactJS in one or two sentences.
Requirement: Foundational ReactJS knowledge
Answer: ReactJS is an open-source Javascript library. It is designed for building dynamic single-page applications with multiple components that require less coding than doing everything yourself in Javascript. Sometimes it is called a framework, but that‘s a question of terminology.
Question 5: What is ReactJS? How does it compare to other JavaScript frameworks?
Requirement: Foundational ReactJS knowledge
Answer: Here you are getting a feel for the candidate‘s knowledge, ideas, and opinions of what React is, and how it fits into the JavaScript ecosystem. Some points to look out for:
- React is an open-source JavaScript library maintained by Facebook;
- It‘s designed for building dynamic and interactive UIs (user interfaces) for web and mobile applications;
- It is used to help you build reusable UI components;
- Sometimes seen as the ’View‘ in the ’Model-View-Controller‘ (MVC) architecture.;
- This means it is a relatively small framework (compared to, say, Angular, which takes care of the whole front end) and can be used easily with other libraries and integrated into many applications;
- React isn‘t as opinionated about an application‘s architecture as other frameworks like Angular and Vue.js;
- Very strong community with many supporting tools and packages.
Question 6: How would you learn about a new Javascript library?
Requirement: Learning on the job
Answer: Web development is changing all the time, and developers need to be able to learn constantly. Here you are finding out how the candidate approaches the learning process. You want to see that they have an idea of what information they will need and where to find it. For Javascript libraries, that means looking up online tutorials and digging into the documentation.
Question 7: Describe a time you received feedback on a programming task. How did it help you become a better programmer?
Requirement: Following instructions and receiving feedback
Answer: This is a typical open-ended question. The candidate should demonstrate they can accept, understand and act on feedback.
Question 8: A common type of problem we have to solve at this company is _____. How might you think about finding a solution?
Requirement: Thinking like a programmer
Answer: In this question, the problem should be directly related to the work the candidate will actually be doing day-to-day for you. You aren‘t looking for a perfect answer or even necessarily a correct answer. Instead, listen to how they approach solving a problem, their ability to break a problem down into parts, and if they can anticipate problems.
ReactJS Mid-level Developer Interview Questions
Mid-level developers are the workhorses of the software development world. They are fairly experienced and you can rely on them to execute routine tasks with skill and efficiency. They can also mentor junior developers.
However, if you need a developer with high-level design skills to lead your project, you might want to skip down to the expert-level ReactJS programming interview questions.
Note: Important keywords are underlined in the answers. Look out for them in interviews!
Question 9: List some advantages and limitations of ReactJS.
Requirement: Expert ReactJS knowledge
Answer:
Advantages:
- Free and Open Source;
- Highly adaptable;
- Virtual DOM, a lightweight javascript object, makes it extremely efficient;
- JSX increases code reliability and makes mixing HTML and JavaScript much easier;
- Makes UI testing easier;
- Developer tools such as the React.js chrome extension for debugging;
- More SEO friendly than some other JavaScript frameworks;
- Large supporting community.
Limitations:
- Library, not a framework. It handles the UI only. You need to use other libraries for other parts of your application;
- No set way to structure applications, meaning you have to figure it out for yourself;
- Its flexibility can easily let developers make poor choices;
- JSX and inline templating can make the code quite complex, especially for novice developers;
- A different way of thinking and learning curve compared to other frameworks like Angular.
Question 10: Explain what the Virtual DOM is in ReactJS and why it is necessary.
Requirement: Expert ReactJS knowledge
Answer: Virtual DOM is one of the key concepts in React. A good candidate should be able to explain the problems with DOM manipulation and why the virtual DOM helps.
DOM (Document Object Model) manipulation is how web applications update the HTML on a webpage to make it dynamic and interactive. However, updating the DOM is slow and most JavaScript frameworks update it more than they need to. Usually, by updating the entire DOM every time a small change is made on a page.
React tries to reduce this waste by only updating the parts of the DOM that are actually updated. To do this, React keeps track of a corresponding lightweight ’Virtual DOM Object‘ that can be updated and checked for changes much faster than the real DOM.
React uses Virtual DOM snapshots to work out exactly which parts of a page need updating, and updates only those in the real DOM rather than creating a new DOM. By using a virtual DOM, web applications can be made much faster and more efficient.
Question 11: What is JSX? How does it relate to ReactJS? Give a quick example.
Requirement: Expert ReactJS knowledge
Answer: JSX (JavaScript XML) is a preprocessor that allows you to include XML syntax in your JavaScript. Its basic function is to make code more intuitive and easier to read. The React library realizes that often JavaScript and HTML are strongly related, and having them in separate HTML and JavaScript files makes things confusing. Here are some examples of JSX code:
const element = <h1>Hello, world!</h1>;
render() {
return (
<div>
<h1>”Hello, World!”</h1>
</div>
);
}
It isn‘t mandatory to use JSX with React, but it makes a lot of sense to do so.
Question 12: What are the Components in React?
Requirement: Expert ReactJS knowledge
Answer: ReactJS follows a component-based approach. That means that components make up the building blocks of a ReactJS application by splitting the UI up into many separate, reusable components. Components can be thought of like JavaScript functions. They accept inputs called props and return React elements that describe what should be presented to the user.
Question 13: What do the following two ReactJS code segments do?
Requirement: Expert ReactJS knowledge
1:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
2:
import react from 'react'
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}</h1>;
}
}
Answer: Both of the above define components in React. The first is a functional component and the second uses an ES6 class to define a component. However, from the perspective of ReactJS, the above two components are exactly equivalent.
Question 14: What does render() do?
Requirement: Expert ReactJS knowledge
Answer: Every React component has a render() function. Render() returns exactly one React element that represents a native DOM component. Multiple HTML elements must be grouped inside one tag, for example, <group>.
Question 15: What‘s your experience with the Flux architecture pattern?
Requirement: Experience using Flux architecture
Answer: Many ReactJS applications use Flux architecture rather than MVC. Its key feature is that it enforces unidirectional data flow. The three major parts of a flux application are the:
- Dispatcher
- Stores
- View (React components)
Question 16: What‘s your experience with the Redux library?
Requirement: Experience using libraries you use
Answer: Redux is a state management library commonly used with React. You‘re checking if your candidate can talk about using Redux (or another library you use) to build testable applications that can run across different development environments with predictable behavior.
- Single source of truth;
- The state is read-only;
- Changes are made with Pure functions.
Question 17: What are some testing tools you would use for unit testing in a ReactJS application?
Requirement: Unit testing UI components
Hire expert developers for your next project
1,200 top developers
us since 2016
Answer: Unit testing is a technique to test whether isolated segments of code are functioning properly. Some tools for testing ReactJS applications include Enzyme, Jest, react-testing-library, React, unit, Skin-deep, and Unexpected-react.
Question 18: What is the Children prop?
Requirement: Understanding JSX and composition.
Answer: A special prop that takes data between tags. For example:
<Header>Hello world</Header>
props.children in the component Header will be equal to “Hello world”.
Question 19: Can you describe the React Component lifecycle? When you should make an asynchronous call?
Requirement: React component’s lifecycle. React 16/17 breaking changes.
Answer:
The usual component lifecycle looks like this:
- Mounting
- constructor
- componentWillMount (should be mentioned, that in React 16/17 this hook is deprecated and replaced by getDerivedStateFromProps)
- render
- componentDidMount
- Updating
- componentWillUpdate (in React 16/17 it was replaced by getDerivedStateFromProps)
- shouldComponentUpdate
- render
- getSnapshotBeforeUpdate
- componentDidUpdate
- Unmounting
- componentWillUnmount
Before React 16 it was ok to make a call in componentWillMount. But now that componentWillMount has become deprecated the earliest hook for the asynchronous calls is componentDidMount. It‘s also ok to make calls from ’componentDidUpdate‘.
Example ReactJS Expert Interview Questions
Now for the most difficult ReactJS interview questions and answers for experienced ReactJS developers. These are for finding the developers that can design and build you a world-class React application.
Skill Requirements for Senior ReactJS Developers
- Expert ReactJS knowledge;
- Flux architecture;
- Designing for specific requirements (e.g. security, scalability);
- Asynchronous programming;
- Maintaining and upgrading applications;
- Experience in other JavaScript frameworks and libraries (e.g. Node JS);
- Efficient programming and clean code;
- Debugging;
- End-to-end and unit testing;
- Leadership skills;
- Clear communication skills;
- Mentoring less experienced developers.
Question 20: What React patterns do you know? Can you describe what is HOC or Render prop?
Requirements: React patterns and app architecture
Answer: There are a lot of patterns for React, such as:
- Higher Order Component (HOC);
- Render Prop;
- Function as children;
- Container and Presentational components.
HOC is the acronym of High Order Component, which is very similar to High Order Function. It‘s a function that takes a component and returns a new component with some changes. For example, Redux connect functions is the HOC, which takes a component and returns a component with Redux State and Actions.
Render Prop is the pattern for creating components with “render callback”. Usually, it is used for the same purpose as HOC. For example, it is used by React-Router 4 and Context API.
Question 21: Do you have any experience with React 16 Context API? What problems does it solve?
Requirements: React Context API
Answer: Context API provides a way to pass data through the component tree without having to pass props down manually at every level. It can be used to share global data (such as user, theme, or any other information) between child components.
Question 22: What is React Portal and React Fragment? What problems do they solve?
Requirements: React Portal and React Fragment.
Answer: Portals provide a first-class way to render a child component into a DOM node that exists outside the DOM hierarchy of the parent component. For example, it can simplify Modal window rendering or changing information inside <head></head> tags.
React Fragments are for grouping a list of children without adding extra nodes to the DOM. Because React forces us to return only one object from render, we should wrap a child component into a div or a similar container tag. Sometimes it‘s simpler to return several tags without a container to implement some features.
Question 23: What is React Reconciliation? How do you avoid performance issues?
Requirements: React rendering algorithm
Answer: Reconciliation is the process that React uses to efficiently update the DOM. It does this by only updating the parts that need it. At a single point in time, the render() function will create a tree of React elements. On the next state or props update, that render() function will return a different tree of React elements. React then needs to figure out how to efficiently update the UI to match the most recent tree. The process of figuring this out is reconciliation.
To avoid performance issues we should:
- Add ’key‘ prop;
- Use PureComponent with React.memo();
- Memoize functions (reselect, for example).
Question 24: What is the ’PropTypes‘ library?
Requirements: PropType library, data validation
Answer: PropTypes is the type-checking addition to React Library, which exports a range of validators to make sure the data component receive is valid. Using it is a good idea because it reduces the number of bugs and makes components self-documented.
Question 25: Do you have any experience in code splitting with React? Can you describe how a bundle can be split into smaller chunks?
Requirements: Webpack bundling, React.lazy or React Loadable
Answer: React has tools to avoid large bundle sizes. You can use React Loadable, because of its simplicity, but React 16.6 also has solutions such as React Lazy.
React Loadable is a small library for wrapping components into bundles. It provides nice features such as placeholders for “Loading” and “Error” components and flash delay. When a component is wrapped, Webpack will move it into a new bundle.
Open-Ended Questions
Once you‘ve established that your developer is an expert in ReactJS, you should continue the interview by asking some messy and open-ended questions to spark a discussion. Tailor these questions to fit your own job requirements and don‘t be afraid to ask follow-up questions.
Question 26: If you could use whatever tools you like to build our ____ application, what would you use?
Requirement: Design skills, understanding of requirements
Answer: In this question, you are getting a feel for the type of developer you are talking to and how they like to code. You are especially looking for developers that try to understand the requirements first. It‘s a big red flag if a developer gives a list of libraries and tools without understanding the task.
Question 27: How are you involved in the ReactJS community?
Requirement: Passion for web development
Answer: This is a popular question for coding interviews because community and open source involvements are clear indicators of a passionate developer.
Question 28: Describe a time you fixed an error in a web application. How did you approach the problem? What debugging tools did you use? What did you learn from this experience?
Requirement: Debugging, Breaking down a problem into parts
Debugging is one of the key skills for any web developer. However, the real skill is in breaking the problem down practically rather than finding small errors in code snippets. Debugging often takes hours or even days, so you don‘t have time in an interview setting. Asking these questions will give you an idea of how your candidate approaches errors and bugs.
Answer: In the candidate‘s response you should look out for things like:
- A measured, scientific approach;
- Breaking down the problem into parts;
- Finding out how to reproduce the error;
- Expressing and then testing assumptions;
- Looking at stack traces;
- Getting someone else to help/take a look;
- Searching the internet for others that have had the same problem;
- Writing tests to check if the bug returns;
- Checking the rest of the code for similar errors;
- Turn problems into learning experiences.
Question 29: What’s the most important thing to look for or check when reviewing another team member’s code?
Requirement: Mentoring less experienced developers, Leadership skills
Answer: Here you‘re checking for analysis skills, knowledge of mistakes that less experienced developers make, keeping in mind the larger project, and attention to detail.
A good answer might mention code functionality, readability and style conventions, security flaws that could lead to system vulnerabilities, simplicity, regulatory requirements, or resource optimization.
Question 30: What tools & practices do you consider necessary for the Continuous Integration and Delivery of a web application using ReactJS?
Requirement: DevOps systems design, Maintain, and upgrade applications
Example Answer: One example of React applications is create-react-app. It provides a lot of features for building. It is also good to separate config files for development and production.
Hire expert developers for your next project
As for deployment, it depends on what hosting we are using. For example, for Firebase there is nothing complicated: usually, we run the build script and run the firebase deployment script. Same for GitHub pages, Heroku, and Netlify.
For deployment to basic hosting with client-side rendering they might mention nginx and node server.
Question 31: What is your favorite method to fill a React Component with CSS? Do you have an experience with CSS-In-JS libraries?
Requirement: React component styling
Answer: There are several options to style components:
- by className;
- by inline styles;
- CSS-in-JS;
- third-party dom libraries for styling components such as StyledComponents, Radium, CssModules, etc.
Question 32: What is the purpose of React Refs? Can you describe a problem you can solve by using it?
Requirement: React DOM interactions.
Answer: Refs is the method to access a DOM node or React component inside a parent component. A common problem it solves is to focus input or get the child component‘s fields. It‘s better to use refs as little as possible.
Important knowledge areas of ReactJS and software development where you should focus on
We talked about the skillsets and ReactJS interview questions. While these give you a guideline about the specifics that you should look for during the interview, there are general aspects to consider too. These carry plenty of importance since you want effective developers.
We recommend that you look for the following general skills of ReactJS:
1. The knowledge of ReactJS lifecycle methods
ReactJS components have their “lifecycle methods”. You can override them to run your code at particular times during the processing of your application. These lifecycle methods belong to various categories, e.g.:
- Mounting;
- Updating;
- Unmounting;
- Error-handling.
Common examples of lifecycles are the following:
- “ComponentWillUpdate”;
- “ComponentDidUpdate”.
Note that ReactJS developers should understand how the re-rendering a component happens. With ReactJS, the default is to re-render every time the state changes.
2. The knowledge of using the “PureComponent” method
ReactJS developers will need to prevent rendering in some cases. The application might receive new props or a new state. Pure components being the fastest components can replace any component with only a render() function. A developer might think of using the “ShouldComponentUpdate” method in such cases, however, this needs careful consideration.
The “ShouldComponentUpdate” method helps more with performance optimization. If a ReactJS developer uses it to prevent re-rendering, then this might lead to bugs in the program.
ReactJS developers should use the “PureComponent” method for this purpose. It performs a shallow comparison of props and the state. This reduces the likelihood of skipping a required update, which is safer.
3. The knowledge of “setState”
ReactJS developers should know how to use “setState”. It provides an important capability since it schedules updates of the “state” object of a component.
Developers should know that calls to “setState” are asynchronous. This means that ReactJS developers can’t expect the app to reflect the new value immediately after calling “setState”. They need to use an “updater” function too.
ReactJS developers should remember that “setState” calls are in batches. They should schedule updates in a chain by using this property, which prevents conflicts between updates.
4. The knowledge of ReactJS “class components”
ReactJS developers need to have a thorough knowledge of functional and class components. Being a JavaScript framework, ReactJS conforms to JavaScript standards and practices greatly. Programmers need to write a JavaScript function to define a class component.
only class components were used for state management and lifecycle methods before React hooks were introduced. Components in ReactJS help developers to manage the UI effectively. They can divide the UI into independent parts, moreover, they can make these parts reusable.
ReactJS provides detailed API reference documentation. Developers should be familiar with it, which will help them to work with class and react function components. They should know how to use a JavaScript object.
5. Familiarity with “controlled components”
ReactJS developers should know the concept of “controlled components” very well. These are specific types of input form elements. In the case of these elements, a ReactJS component would render the form. It also controls the events in the form upon receiving the next user input.
The React state will drive the value of the input. ReactJS developers should know that they need to write more lines of code to use a “controlled component”. They should know the advantages though, i.e., passing the values to other UI elements.
6. The knowledge of using “stateless components”
ReactJS programmers should know the concept of “stateless components”. JavaScript functions play a part here since a stateless component is a functional component. Such functional components take props as inputs, subsequently, they return a ReactJS element.
“Stateless components” don’t have a lifecycle. ReactJS calls the “MyStatelessComponent” function to render a “stateless component”, and developers should know about it.
7. The knowledge of using “Higher-Order Components” in ReactJS
ReactJS developers should know how to reuse component logic effectively. This makes their work easier, and “Higher-Order Components” (HOCs) can help. These are advanced techniques available to ReactJS programmers.
HOCs are not part of the ReactJS APIs but are patterns derived from react’s compositional nature. They are functions to take one component and return a new component.
8. The knowledge of ReactJS “stateful components”
We talked about “stateless components”, however, ReactJS also have “stateful components”. Such a component contains the state object. States allow the creation of dynamic and interactive components. ReactJS developers should know how to use these components. They also need to know how to initialize “this.state”.
9. The knowledge of using ReactJS “arrow functions”
ReactJS developers should know how to pass functions to components. In this context, knowing an “arrow function” becomes important. These functions present easy ways to pass parameters to “callback” functions.
The knowledge of passing functions has other benefits too. E.g., developers can pass an event handler like “onClick” to a component.
10. The knowledge of “React Router” and routing in ReactJS
ReactJS programmers need knowledge of routing. More specifically, they need the knowledge of “React Router”. This standard library enables routing.
Routing makes it easy to navigate among views in various components in a ReactJS app. It allows one to change the browser URL, furthermore, it keeps the user interface synchronized with the URL.
11. The knowledge of synthetic events in React
You need a ReactJS developer to know about the browser’s native event and event handling. ReactJS provides useful wrappers that make up the event system of ReactJS. The “SyntheticEvent” wrapper is a key one of these wrappers, and developers should know about it.
12. Miscellaneous ReactJS skill areas
Look for ReactJS developers with skills in a few other aspects. They should know about “babel”, “createElement“, controlled and uncontrolled components, “mutable state”, “reducer”, templates, “classname”, “createclass”, “DOM elements”, “getInitialState”, HTML element, inline styles, internal states, lifecycle hooks, the difference from react native, etc. ReactJS developers should have significant debugging skills. Well-rounded skills in all of these areas can help your project significantly.
Summary
Hiring the right people for your development team is critical to the success of your project. Remember that you should not be aiming to hire the perfect ReactJS developer, but rather the right person for the job at hand.
With the help of this information and sample ReactJS interview questions, you can ensure that the hiring process goes smoothly and successfully – allowing you to hire a great programming team to get your project completed on time and on budget.