- Developers
- Developer Blog
- AI Software Development
- How to Use ChatGPT API?
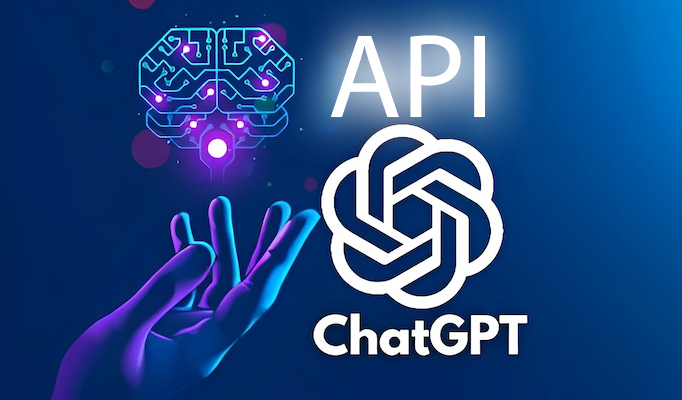
profile

By Faiza Khalid
Verified Expert
5 years of experience
Faiza is a CIS engineer with a keen interest in software development, AI research, and technology writing.
Wondering how to use ChatGPT API in your software application?
Developing a chatbot application using ChatGPT API requires a deep understanding of generative pre-trained models, transfer learning, deep learning, large language models, etc.
That is why hiring a professional team with relevant experience from a software development company like DevTeam.Space, with a vetted developers community instead of freelancers, is essential for successful software product development. More on this later.
Let’s discuss how to use ChatGPT API in your application in detail.
Using ChatGPT API
Take the following steps to use ChatGPT API in your software:
1. Determine the Scope of Using ChatGPT in Your Application
ChatGPT is a powerful generative AI text-based model. ChatGPT API allows developers to integrate ChatGPT capabilities in their applications, like natural language understanding, text generation, and chatbot functionality.
You need to identify the use cases of ChatGPT in your application to use it effectively. For instance, you might want to integrate ChatGPT for one or more of the following reasons:
- Content generation;
- Customer support;
- Natural language interface;
- Data extraction and categorization, etc.;
You need to determine the scope of your project, e.g., you want to integrate ChatGPT into your web app or mobile application. Assess the extent of customization you want in your ChatGPT-based features.
If you need extensive customization to meet specific business needs, you might want to opt for a custom ChatGPT integration approach.
Hire expert AI developers for your next project
Note: This blog is intended to explain the basic approach of integrating ChatGPT using an API. You can refer to our blog on how to conduct ChatGPT integration for details on custom ChatGPT integration.
Understand the Key Concepts of Using ChatGPT API
Get familiar with key terms and concepts associated with ChatGPT API to use it efficiently for any NLP-related task. Some key concepts include the following:
- Prompts: These are initial instructions given to the model to guide its response. When a user sends a prompt as a part of an API request, it helps frame the conversation and is a starting point for a response.
- Tokens: The model understands and processes text by breaking it down into tokens. A token can consist of complete words or a group of characters.
- Models: The OpenAPI API is powered by different models that have different capabilities and pricing. ChatGPT API is powered by GPT-3.5-Turbo. It is optimized for conversational features.
Fine-tuning for specific applications is currently available on gpt-3.5-turbo, cabbage, and davinci models of OpenAI. It is expected that gpt-4 will be available for fine-tuning by the end of this year. Moreover, gpt-4 API has a waitlist as of writing, while GPT 3.5 models are accessible to everyone.
You can refer to OpenAI documentation for more information. Read our article on navigating ChatGPT SDK documentation.
3. Get OpenAI API Keys
To start using ChatGPT API, you require API keys from OpenAI. Sign up on the website, click on the personal tab, select view API keys to go to the API keys page, and click on the Create new secret API key button to generate new API keys.
Make sure to save your API keys, as they won’t show again.
4. Choose a Programming Language and SDK
ChatGPT API has SDKs and libraries in multiple programming languages, like Python and JavaScript. Install libraries and SDKs in the programming language you want to work with using any package manager like pip or npm.
5. Setup an API Client
Now, you will set up the client application. Install the necessary dependencies and import the required libraries to interact with the API. Create an API instance by providing your API keys and required configurations.
6. Make API Requests
Once you have an API instance, you can start making requests. You can use ChatGPT APIs for chat completion and text completion tasks.
The API request construct typically includes the following:
- “model”: Specifies the OpenAI model, such as gpt-3.5-turbo.
- “messages”: Each message object in the messages list has role and content fields. The role field specifies the role of the message in the conversation. It has two possible values: user and system.
- The user role represents what a user would say or ask during the conversation. It provides context and specific instructions to the assistant.
- The system role sets the behavior and characteristics of the assistant.
- The content field represents the actual text of the message based on the role specified. For a system role, it can include instructions, like you are a helpful assistant. For a user role, it can contain user queries, statements, etc.
You can choose from the following two methods to use ChatGPT API:
Using the API Endpoint
You can use the /v1/ chat/completions endpoint to connect to the gpt-3.5-turbo and gpt-4 ChatGPT models. You can directly use endpoints by using the requests library in Python, as shown in the following example:
import requests
import json
endpoint_url = “https://api.openai.com/v1/chat/completions”
api_key = “YOUR_API_KEY”
headers = {
“Content-Type”: “application/json”,
“Authorization”: f”Bearer {api_key}”
}
data = {
“model”: “gpt-3.5-turbo”,
“messages”: [
{“role”: “system”, “content”: “You are an assistant who tells random fun facts.”},
{“role”: “user”, “content”: “Tell me a joke.”}
]
}
response = requests.post(endpoint_url, headers=headers, json=data)
json_response = response.json()
reply = response_json[“choices”][0][“message”][“content”]
print(reply)
Hire expert AI developers for your next project
1,200 top developers
us since 2016
Using the Openai Library
You can use pip to install the openai Python library (pip install openai). Next, you can generate text or chat completion calls. Consider the code below:
import openai
openai.api_key = “YOUR_API_KEY”
response = openai.ChatCompletion.create(
model = “gpt-3.5-turbo”,
temperature = 0.4,
max_tokens = 1000,
messages = [
{“role”: “user”, “content”: “Who won the last US presidential election?”}
]
)
print(response[‘choices’][0][‘message’][‘content’])
Synchronous and asynchronous calls
The ChatGPT API supports both synchronous and asynchronous methods. In a synchronous API, you send a single message to the API and receive a response within the same request.
You can construct an API call by specifying the model and providing messages as the input. The following code snippet shows a synchronous API call using the openai library:
import openai
openai.api_key = ‘YOUR_API_KEY’
response = openai.Completion.create(
engine=”gpt-3.5-turbo”,
prompt=’Tell me a joke.’,
max_tokens=30
)
reply= response.choices[0].text.strip()
print(reply)
In an asynchronous method, you maintain a stream of conversation by sending a list of messages to the API. The list of messages allows the model to understand the context of a conversation and provide more accurate responses.
For an asynchronous API, you will specify the model, the conversation history, and any additional parameters, such as max_tokens, temperature, etc.
A higher temperature value indicates the model response will be more random and creative, while a low value shows the response is more deterministic.
Consider the following example of an asynchronous call in Python using the ChatGPT API endpoint:
import requests
api_url = “https://api.openai.com/v1/chat/completions”
headers = {
“Content-Type”: “application/json”,
“Authorization”: “Bearer YOUR_API_KEY”
}
data = {
“model”: “gpt-3.5-turbo”,
“messages”: [
{“role”: “system”, “content”: “You are an assistant who gives useful information.”},
{“role”: “user”, “content”: “How can you assist me?”}
],
“max_tokens”: 40,
“temperature”: 0.6
}
response = requests.post(api_url + “/completions”, headers=headers, json=data)
response_json = response.json()
Utilize the Response of ChatGPT API
After you receive the response, you can extract the information provided by the assistant based on your prompts and utilize the text data in your application as required.
The API returns a response in JSON format. As shown in the example, you can parse the JSON response to extract the text generated by the model.
Hire expert AI developers for your next project
You can hold an iterative conversation by maintaining the conversation history and including the previous messages in the subsequent API request to generate responses. It allows the model to provide contextually relevant responses.
ChatGPT API has usage limits. It is important to monitor your API calls and stay within the limits. You can learn more about ChatGPT API usage and pricing plans.
Planning to Utilize ChatGPT API in Your Application?
The procedure to use ChatGPT API in your application is not very complex. However, if you plan to integrate ChatGPT into your business or commercial software, we suggest you hire a small team of professionals to integrate ChatGPT into your application seamlessly.
The team will consist of a mobile or web developer, an app tester, a business analyst, and a project manager.
Your developer will create necessary requests and handle responses. The app tester will validate outcomes and test your application.
A business analyst will help you decide on the required specifications requiring ChatGPT integration. A capable project manager will oversee the project execution and implement course corrections whenever necessary.
If you are developing a software application with extensive ChatGPT integrations app and need to scale your team with additional skills and expertise then take a moment to tell us about your project requirements here.
One of our dedicated tech account managers will be in touch to show you similar projects we have done before and share how we can help you
FAQs
You can use OpenAI’s API to integrate ChatGPT capabilities such as to generate human-like text into your application. You will need the ChatGPT API key for authentication and authorization. After you get API access, you can create requests to interact with specific ChatGPT API endpoints and generate human-like responses. You will handle the response by extracting relevant information from the response JSON and utilizing the information in your application.
No, ChatGPT API is not free. However, API users get a free credit of $18 when they open an account on OpenAPI. The GPT 3.5-turbo API costs $0.002 for 1000 tokens. You can consider tokens as pieces of words. 1K tokens are about 750 words.
You can use ChatGPT with your data by fine-tuning GPT 3 base models based on your data collection setup. The fine-tuning technique allows you to create a custom ChatGPT model by training a base model on business-specific data. This process requires specialized expertise in AI model development. We suggest you partner with expert AI developers, such as those available at DevTeamSpace, for a custom ChatGPT development project.
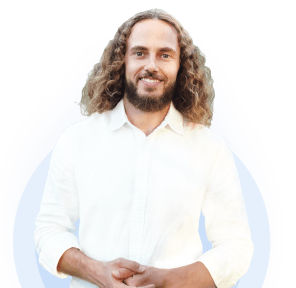
Alexey Semeney
Founder of DevTeam.Space
Hire Alexey and His Team
To Build a Great Product
Alexey is the founder of DevTeam.Space. He is award nominee among TOP 26 mentors of FI's 'Global Startup Mentor Awards'.
Hire Expert Developers