- Developers
- Developer Blog
- Mobile App Development
- How to Use Clean Architecture for Android?

profile

By Aran Davies
Verified Expert
8 years of experience
Aran Davies is a full-stack software development engineer and tech writer with experience in Web and Mobile technologies. He is a tech nomad and has seen it all.
Wondering how to use clean architecture for Android? Read this article to get an understanding of what clean architecture is and how to use it in Android development.
In this article
- Understand the specifics and value of clean architecture for Android apps
- Implement clean architecture in an Android app
- FAQs on Implementing Clean Architecture for Android
Clean architecture can help to develop and maintain software applications with fewer mobile developers. How to use clean architecture for Android, a mobile operating system that accounts for more than 70% of the global mobile OS market? Take the following steps:
Understand the specifics and value of clean architecture for Android apps
Why should you implement clean architecture in Android development? Thinking through the following series of questions will provide the clarity:
A. Why use software architecture at all?
You need a software architecture to manage the complexities of a software application. By choosing the right architecture, you can deliver functionality effectively. You also take care of the testability, maintainability, security, scalability, performance, etc. by choosing the right architecture.
You might choose to not focus on the software architecture in a simple project. However, you certainly need to choose the right architecture pattern for a complex project.
B. What prompted the creation of clean architecture?
An effective architecture pattern should follow a set of 5 principles to the maximum extent possible. These principles help to develop maintainable code. You can create a flexible app using them. They help in developing quality code, which makes it easier to understand the app.
Together, these 5 principles are known as the “SOLID principles”. These are as follows:
- Single responsibility: One software component should have one responsibility only. You should have only one function for each component.
- Open-closed: Programmers should be able to extend the functionality of a component without adversely impacting it. They shouldn’t have to modify its extensions for this.
- Liskov substitution: Assume you have a class that has a subclass in an app. You can represent the usage of the base class with the subclass, and you don’t need to disrupt the app for this.
- Interface segregation: Programmers should try to have many smaller interfaces. They should avoid having one large interface. Having many smaller interfaces will eliminate the need for a class to implement unnecessary methods.
- Dependency inversion: The dependency principle mandates that the higher-level modules shouldn’t have any dependency on lower-level modules. Software components should depend on abstractions. They shouldn’t depend on the concrete implementation details.
There are several software architecture patterns. However, they had a few gaps in implementing one or more of these principles. That created the following challenges:
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
- UI was coupled with other layers in the architecture in an ineffective manner.
- The business logic layer is often coupled with persistent storage.
- The application wasn’t sufficiently testable.
Maximizing the use of the SOLID principles would solve the above challenges. This quest prompted the creation of clean architecture.
C. What is clean architecture?
Robert C. Martin, also known as “Uncle Bob” created the clean architecture to maximize the use of the SOLID principles. He first presented his solution in 2011 in the form of an article named “Clean Architecture”.
The “Clean architecture” pattern is a software architecture pattern with layers. This pattern has a dependency rule that mandates that the inner layers can’t depend on the outer layers. Any code in the inner layer of an application can’t refer to any code in the outer layer. These layers will look like an onion if you try to visualize this dependency rule.
Clean architecture doesn’t mandate the number of layers that you should have in your app. You can choose the number of layers based on your requirements. However, you need to follow the above-mentioned dependency rule.
Apps using the clean architecture pattern often have the following 5 layers:
- Presentation: The presentation layer interacts with the user interface (UI).
- Use cases: This layer is also called “interactors”. This defines the actions that a user can trigger.
- Domain: The domain layer consists of the business logic of your app.
- Data: The data layer includes the abstract definitions of all relevant data sources.
- Framework: This layer consists of the interactions with the relevant SDKs and frameworks. This layer helps you to implement the data layer.
D. What are the advantages of using the Android clean architecture?
Clean architecture has several advantages. Many Android developers have embraced this pattern for their Android app architecture. The advantages are as follows:
- You can implement new features quickly.
- You can implement separation of concerns well.
- Developers can easily see the business use cases. It provides them clarity.
- Clean architecture provides flexibility since you aren’t tied to specific frameworks or databases.
- This architecture pattern helps you to use the “domain-driven design” (DDD). This design approach is useful in complex software development projects.
- You can test your application better with clean architecture. You can test various interfaces and external interactions easily.
- Project execution becomes quicker.
E. When should you use the Android clean architecture?
You can use the clean architecture pattern for complex projects. This architecture pattern helps to execute large projects.
If you have a large body of business logic to implement, then the clean architecture pattern is very useful. It provides clarity. Team members can collaborate more effectively.
Clean architecture has a steep learning curve. That could offset its benefits in small projects. Therefore, think carefully before implementing it in simple projects.
Implement clean architecture in an Android app
Do the following to implement the clean architecture pattern in an Android app:
A. Set up the project structure
Note: You can refer to the clean architecture tutorial for Android for details.
Hire expert developers for your next project
1,200 top developers
us since 2016
Open a project on Android Studio. Divide the project into two modules, which are as follows:
- The “app” module: It’s an existing module.
- The “core” module: This consists of the code that doesn’t depend on any Android SDK.
Create the “core” module by taking the following steps:
- Use the “File > New > New Module” menu on Android Studio.
- Select “Java Library” in Android Studio, and name the library “core”.
- Edit the Java package name field and enter a package name.
- Click “Finish”, which will start the Gradle synchronization operation.
- Open “build.gradle” in the core module to apply the relevant plugins and dependencies.
- Update “build.gradle” to create a reference to the “core” module from the “app” module.
- Let the Gradle synchronization process run.
- Create packages for domain, data, and interactors.
B. Create the domain layer
The domain layer of your app will consist of all the business rules. Do the following to create this:
- Move the “bookmark” and “document” models to the core module from the starter project.
- Move the “bookmark” and “document” files from the app module to the core module.
- Complete the process by clicking on the “Refactor” button.
C. Create the data layer
This layer contains the abstract definitions for accessing data sources, e.g., databases. Create this layer by taking the following steps:
- Use the “repository pattern”.
- Add one “data source” interface and “repository class” for each model.
By using the repository pattern, you can implement the dependency inversion principle effectively.
D. Add repositories
Select the project file in the core module. Add a Kotlin or Java file/class by using the menu options in the IDE. Assign the relevant name to it and click “Finish”. Add relevant code for the above-mentioned interface like “import”, “add”, “read”, and “remove”.
Repeat the above process to add more interfaces and data sources. Type in the relevant code in these files.
E. Create the use cases layer
This layer will contain the code to convert user actions and pass them to the inner layers of your app. Create a class for each use case. For this, create a Java/Kotlin file in your project.
Add the relevant code to this file. Ensure that one use case has one function only. Repeat this process for each use case.
F. Create the framework layer
The framework layer consists of the code that implements the interfaces specified in the data layer. In your Android app, the framework layer will depend on the Android SDK. You need to create this layer in the “app” module.
Create a new Java/Kotlin file in the “app” module of the project. Identify a data source from the data layer. In the new Java/Kotlin file, type in the code required to implement that data source. Repeat this process for all the data sources specified in the data layer.
Hire expert developers for your next project
G. Create the presentation layer
The presentation layer in your Android app contains the UI-related code. This layer is in the same outer circle as the framework layer. Therefore, the presentation layer can use the classes specified in the framework layer.
You can use well-known design patterns like MVP, MVI, or MVVM. Many Android developers understand the MVVM pattern very well, therefore, you can opt for it.
The MVVM pattern has the following 3 components:
- View: It brings the UI to users.
- Model: This component consists of the data and business logic.
- ViewModel: It’s the link between the UI and data.
When you implement the MVVM framework in clean architecture, you will not rely on models. Instead, the app will communicate with the interactors that you created in the use cases layer. Continue with the MVVM implementation. This completed the implementation of the clean architecture in your Android app.
Trying to implement the clean architecture in your Android development project? Contact DevTeam.Space to hire competent mobile developers.
FAQs on Implementing Clean Architecture for Android
You can use either Java or Kotlin. Clean architecture helps you to write quality code, and it’s useful in complex projects. It doesn’t depend on one particular programming language.
You should use Android Studio, the popular IDE for native Android development. Clean architecture doesn’t depend on any other Android SDK.
Clean architecture helps you to write software applications that are easier to test and maintain. Your codebase will certainly be easier to understand if you implement this architecture pattern.
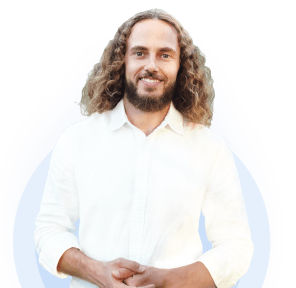
Alexey Semeney
Founder of DevTeam.Space
Hire Alexey and His Team To Build a Great Product
Alexey is the founder of DevTeam.Space. He is award nominee among TOP 26 mentors of FI's 'Global Startup Mentor Awards'.
Alexey is Expert Startup Review Panel member and advices the oldest angel investment group in Silicon Valley on products investment deals.