50 Angular Interview Questions and Answers for 2024
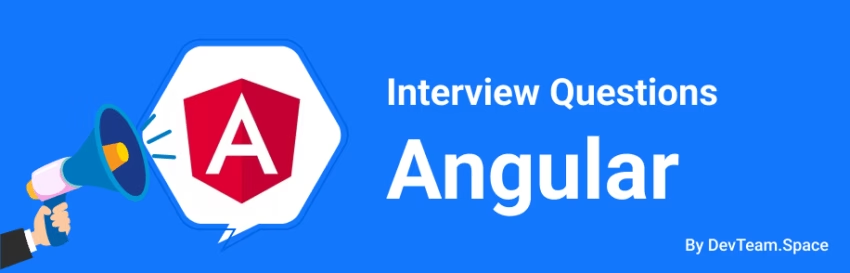
Why should a guide with 50 Angular interview questions and answers matter to you? Well, it has to do with the challenges of finding competent developers, especially for popular development frameworks like Angular.
Whether you're an entrepreneur, a project manager in a large enterprise, or a CTO, you are well aware that the success of your project rests on your ability to find top developers.
In this guide, you‘ll find example interview questions and answers you can refer to when seeking a new Angular developer to build your web applications. You‘ll also get some practical advice on how to use these basic Angular interview questions to reliably identify the best people for the job.
These interview questions are for Angular versions 2, 4, 5, 6, 7, 8, and 9 (simply referred to as ’Angular‘). If you are looking for developers for the original AngularJS JavaScript framework, click here for our client-side AngularJS interview questions.
You can also read our article on Angular 3: Release Date, Features, and Changes for more information on Angular 3.
Angular: A brief introduction
Why would you need Angular developers? Well, in our guide “10 biggest challenges when developing an app”, we have explained why you need to choose the right technology stack when developing an app.
Angular is a popular web development framework. You can also create mobile apps using it, however, remember that we aren’t talking about native apps here.
Businesses often want to keep their development and maintenance costs down, therefore, they develop hybrid apps. With this approach, they develop once and deploy on both Android and iOS. However, hybrid apps often fall short of the native experience. Modern frameworks like Angular and React have changed that quite a bit. They deliver a near-native experience. If you are planning to develop such a mobile app, Angular can be a great option.
The Angular team at Google has developed this framework, and it’s a complete rewrite of Angular JS. Angular is an open-source framework written in JavaScript code. You can read “Introduction to Angular docs” for more information.
Angular has both similarities and differences with JavaScript in many aspects. Take the example of Angular expressions and Javascript expressions. Angular expressions are code snippets placed in binding. However, Angular expressions are evaluated against a scope object. That’s different for JavaScript. JavaScript expressions are evaluated against the global window object.
The benefits of using Angular
What advantages can you get if you use Angular in your project? Let’s take a closer look at this. The benefits of using Angular are as follows:
- It supports the popular “Model-View-Controller” (MVC) architecture. You can isolate the application logic from the UI visual layer, which is important for ensuring the separation of concerns.
- The Angular architecture helps developers to locate the code that requires changes, which helps in development.
- Angular supports modules, which helps developers.
- Thanks to “Angular Injector”, Angular supports services and hierarchical dependency injection. This is another way in which it supports developers.
- Angular uses “TypeScript”, a superset of JavaScript. It eliminates common programming errors, resulting in better-quality code.
- Programmers can use the comprehensive documentation of Angular.
Read more about the advantages of Angular in “What are the advantages and disadvantages of Angular?”.
The popularity of Angular
You would surely want to use a framework that many businesses trust, wouldn’t you? It’s a highly popular framework.
The “Stack Overflow developer survey results 2019” report finds that Angular is one of the most popular web frameworks. 30.7% of the survey respondents stated that they use Angular, and it’s the 3rd most popular framework.
The “2019 HackerRank developer skills report” also indicates that Angular is a very popular framework. According to this report, 33.05% of the surveyed developers knew Angular. That’s an increase from 2017 when 32.46% of surveyed programmers knew Angular.
First: Select Your Job Requirements
The most common mistake interviewers make is to start questioning candidates without a clear definition of the type of developer they are looking for. Building Angular applications requires many different skills, and you need the developers with the right ones for your unique web app. You also need to define any ’soft‘ skills they will need to work effectively in your team.
Some example requirements include:
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
- Essential web development skills – E.g. HTML, CSS, JavaScript
- Angular-specific skills – E.g. TypeScript, Building Single Page Applications using the Index.html in a single-page application, the knowledge to create custom component directives, etc.
- Library/toolkit experience – E.g. Jasmine and Karma
- The knowledge of using the Angular CLI
- The knowledge of popular JavaScript libraries like jQuery
- Design skills – Performance optimization, Application Security
- Communication skills – Discussing problems and constraints
- Initiative – If they‘ll have to figure out solutions by themselves
- Node.js skills - using this runtime environment makes it easier to develop Angular apps.
Avoid making a laundry list of non-essential skills for your perfect Angular developer. Instead, focus on what your candidate will actually be doing day-to-day. Keep your requirements list as short as possible. Cut anything they can do without or learn on the job.
With clearly stated requirements, you‘ll be able to choose the right coding interview questions and have a much better idea of what kinds of responses you are looking for.
The questions in this guide are broken down into two sections: Junior Angular Developer Interview Questions and Senior Angular Developer Interview Questions. You should use the Angular coding interview questions for junior developers in this section if you‘re looking for a junior or entry-level developer with less experience.
If you are looking for a junior-level professional then read the section for junior-level programmers, and for experienced developers, skip to the Senior Developer sections)
Angular Junior Developer Interview Questions
These are some Angular basic interview questions for screening junior developers. It‘s important to know before hiring a Junior Angular developer that they will need guidance from more experienced developers.
Therefore, a great junior dev will be able to execute tasks given by senior developers. Skip to the Senior Developer section if you need someone who can lead your AngularJS application.
Skill Requirements for Junior Angular Developers
- Basic HTML, CSS, JavaScript, and TypeScript skills;
- Foundational Angular knowledge;
- Learning on the job;
- Following instructions and receiving feedback;
- Thinking like a programmer.
Example Angular Junior Developer Interview Questions and Answers
Note: Important keywords are underlined in the answers. Bonus points if the candidate mentions them!
Question 1: Write an example of a simple HTML document with some header information and page content.
Requirement: Basic HTML skills
Answer: HTML documents are all different, but they follow a basic structure of the head and body. Here you‘re checking the candidate has a good grasp of HTML document structure and basic HTML tags such as DOCTYPE, html, head, title, meta, body, h1, p, associated custom element tag, etc.
For example:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<meta charset="UTF-8">
<meta name="description" content="Page description">
</head>
<body>
<h1>Interview Example Web Page</h1>
<p>Some content goes here</p>
</body>
</html>
Note: Angular provides useful functions to manage the page content. An example is the “uppercase” filter, which converts a string to uppercase. Conversely, the “lowercase” filter can convert a string to lowercase. Developers should know about these, moreover, they should know how to use Angular “div”.
Question 2: Briefly explain the CSS box model. Write some code snippets to describe and show what you mean.
Requirement: Basic CSS skills
Answer: CSS along with HTML and JavaScript is one of the basic building blocks of the Internet. CSS describes how webpages look. Every front-end developer should have good CSS knowledge. Good candidates will be able to describe CSS concepts concisely.
The CSS box model refers to the layout and design of HTML elements. It‘s a box shape that wraps around each HTML element. A box is made up of its content, padding, border, and margin.
- Content of the box
- Padding
- Border
- Margin
(the same padding on all 4 sides)
padding: 25px;
(padding for the top, right, bottom, and left)
padding: 25px 50px 75px 100px;
(top/bottom padding 25 pixels, right/left padding 50 pixels)
padding: 25px 50px;
Question 3: In JavaScript, how can the style of an HTML element be changed?
Requirement: Basic JavaScript code skills
Answer: For example, to change the font size:
document.getElementById(“someElement").style.fontSize = "20";
Question 4: Write some code for a basic class in TypeScript with a constructor and a method.
Requirement: Basic TypeScript skills
Answer: Here‘s a simple class that is created with a greeting message which can be retrieved with the greet() function.
class Greeter {
greeting: string;
constructor(message: string) {
this.greeting = message;
}
greet() {
return "Hello, " + this.greeting;
}
}
let greeter = new Greeter("world");
Note: Angular developers should also know about conditionals. They need to know how to add a conditional class in Angular using ngClass.
Question 5: What are Single Page Applications? How do they work in Angular?
Requirement: Foundational Angular knowledge
Answer: Single Page Applications (SPAs) are web applications that use only one HTML page. As the user interacts with the page, new content is dynamically updated on that master page. Navigation between pages happens without refreshing the whole page. Angular uses AJAX to dynamically update HTML elements. Angular Routing can be used to make SPAs. The result is an application that feels more like a desktop app rather than a webpage.
Angular provides useful ways to develop dynamic websites and display dynamic data on an HTML template at the user end. An example is “String Interpolation”. It helps to fetch angular component data from a component to an HTML template. Note that it uses double curly braces as its delimiter.
Question 6: How is an Annotation different from a Decorator and What‘s the basic syntax of a Decorator in Angular?
Requirement: Foundational Angular Knowledge
Answer: An Annotation is used to create an annotation array while a Decorator in angular refers to a design pattern to modify a class without changing the original source code.
Syntax of a Decorator: @() with optional parameters.
Question 7: What is [(ngModel)] used for?
Requirement: Foundational Angular Knowledge
Answer: Two-way data binding in Angular. If they say one-way binding they are incorrect.
You should expect an Angular developer to know about the Angular digest cycle. This process helps with Angular data binding.
An Angular developer should know about Angular event binding too. This helps an app to respond to user actions like keystrokes and clicks. Angular developers need a thorough knowledge of the event-binding syntax.
Question 8: What are the basic parts of an Angular application?
Requirement: Foundational Angular Knowledge
Answer: Modules, Components, Property Binding, Template expression, Structural Directives, Dependency Injection, Services, Routing.
Note: Angular developers should know about structural directives in Angular well. Structural directives are one of the 3 types of directives in Angular. The other 2 types are as follows:
- Components: These are directives with templates, for example, angular material is a UI component library. A component consists of HTML, CSS, and JavaScript for a specific portion of a user interface.
- Attribute directives: These are used for changing the appearance or behavior of an element or component.
Angular also uses an element named “selector”. The metadata for a directive associates a decorated class with a selector. This is used for inserting it into HTML.
Angular developers should know about the ng-app directive too. They need to know how to use it to signify the root module element. Developers should have sound knowledge of Angular “root scope”.
Question 9: Tell me about a time you received feedback on a task.
Requirement: Following instructions and receiving feedback
Answer: This is a typical open-ended question. The candidate should demonstrate they can accept, understand and act on feedback.
Question 10: Describe how you would approach solving (some problem) on a high level.
Requirement: Thinking like a programmer
Answer: In this question, the problem should be directly related to the work the candidate will actually be doing. You aren‘t looking for a perfect answer or even necessarily a correct answer. Instead, listen to how they approach solving a problem, their ability to break a problem down into parts, and if they can anticipate problems.
Note: An Angular developer should know how to make effective use of a DOM element. Look for this experience during the interview.
Question 11: What are some advantages of using the Angular framework for building web applications?
Requirement: Expert Angular components knowledge
Answer: Advantages of using the Angular framework include:
- Angular does lots of things for you under the hood. It saves time for developers by doing a lot of the work for them like writing tedious DOM elements manipulation tasks.
- TypeScript and the Angular framework allow you to catch errors much earlier.
- In many cases has faster performance than traditional web development techniques.
- Can give web apps the feel of a desktop application.
- It separates out the code of an application to make it easier for multiple developers to work on an app and test it.
- A more consistent code base that‘s easy to maintain.
- Big developer community.
Question 12: What function is called when an object is created in TypeScript? What is its basic syntax in TypeScript code?
Requirement: TypeScript knowledge
Answer: The constructor function is called. Its syntax is: Constructor(){}
Note: Angular developers should have sound knowledge of the scope object in Angular, which is a built-in object.
Question 13: In Angular, how can you interact between Parent and Child components?
Requirement: Expert Angular knowledge
Answer: When passing data from the Parent to Child component, you can use the @Input decorator in the Child component. When passing data from the Child to the Parent component, you can use the @Output decorator in the Child component.
Question 14: Write an example usage of ngFor for displaying all items from an array ’Items‘ in a list with
<li>.
Requirement: Expert Angular knowledge
Answer:
Hire expert developers for your next project
1,200 top developers
us since 2016
<li *ngFor=”let item of Items”>{{item}}</li>
Note: Angular developers should know about filters that select a subset of items from an array.
Question 15: What is the sequence of Angular Lifecycle Hooks?
Requirement: Foundational Angular knowledge
Answer: OnChange() - OnInit() - DoCheck() - AfterContentInit() - AfterContentChecked() - AfterViewInit() - AfterViewChecked() - OnDestroy().
Question 16: If you provide a service in two components‘ “providers” section of @Component decorator, how many instances of an angular service shall get created?
Requirement: Foundational Angular knowledge
Answer: 2
Question 17: What is the main difference between Angular constructors and an import component oninit from Angular core?
Requirement: Foundational Angular knowledge
Answer: The constructor is a feature of the class itself, not Angular. The main difference is that Angular will launch ngOnInit after it has finished configuring the component. Meaning, it is a signal through which the @Input() and other banding properties and decorated properties are available in ngOnInit, but are not defined within the constructor by design.
Angular Senior Developer Interview Questions
Here are some more advanced and technical Angular interview questions and answers for experienced developers. Use them to pick out Angular developers with the right skills to build your Angular app.
An expert Angular developer has to know the Angular framework inside and out. In other words, your perfect hire should be able to answer your Angular 6 interview questions (or any other Angular version) easily.
They will also be able to design efficient Angular applications, write clean and robust code, work effectively with your team, develop business logic, and pass on their experience to junior developers. Remember to list your requirements before you choose your questions.
Skill Requirements for Senior Angular Developers
- Expert Angular knowledge and its different versions (2, 4, 5, 6, 7);
- Component-based architecture;
- Designing for specific requirements (e.g. security, scalability, optimization);
- Maintaining and upgrading applications;
- Experience in frameworks/toolkits/libraries you use;
- Efficient programming and clean code;
- Debugging;
- End-to-end testing and unit testing;
- Leadership skills;
- Clear communication skills;
- Mentoring less experienced developers.
Example Angular Senior Developer Interview Questions and Answers
Note: Important keywords are underlined in the answers. Look out for them in interviews!
Or save yourself time and request a team to match your needs right away.
Question 18: What modules should you import in Angular to use [(ngModel)] and reactive forms?
Requirement: Middle Angular knowledge, Tools/libraries
Answer: FormsModule and Reactiveforms Module.
Question 19: How similar is AngularJS to Angular 2?
Requirement: Middle Angular knowledge
Answer: Both are front-end frameworks maintained by Google, but Angular 2 is not a simple update of AngularJS, it is a new framework written from scratch. Updating an app from AngularJS to Angular 2 would require a complete rewrite of the code.
Question 20: What were some features Angular introduced in the different versions of Angular (2, 4, 5, and 6)?
Requirement: Expert Angular knowledge, Component-based architecture
Answer:
Angular 2:
- Complete rewrite of the Angular framework;
- Component-based rather than controllers/view/$scope. This allows more code to be reused, easier communication between multiple components, and easier testing;
- Much faster;
- Mobile support;
- More language choices such as TypeScript.
Angular 4:
- An update to Angular 2, not a complete rewrite. Updating from Angular 2 to 4 just requires updating the core libraries;
- Improvements to Ahead-of-time (AOT) generated code;
- Support for new versions of TypeScript;
- Animation packages are removed from the core package;
- Else block.
Angular 5:
- Focused on making Angular smaller and faster to use;
- HTTP is depreciated and the HttpClient API client is now recommended for all apps;
- Supports TypeScript 2.3;
- Introduction of a build optimizer;
- Angular Universal State Transfer API;
- Improvements to the Angular Compiler;
- Router Lifecycle Events;
- Better cross-browser standardization.
Angular 6:
- Better service worker support;
- Better URL serialization;
- Ivy rendering engine;
- ng update and ng add;
- <template> element completely removed;
- Angular-specific Elements/Custom Angular Elements;
- Form validation changes;
- Schematics.
Question 21: What is Transpiling in Angular?
Requirement: Middle Angular knowledge, TypeScript
Answer: Transpiling means converting the source code of one programming language into another. In Angular, that usually means converting TypeScript into JavaScript. You can write the code for your Angular application in TypeScript (or another language such as Dart) that is then transpiled to JavaScript for the application. This happens internally and automatically.
Question 22: What is AOT Compilation?
Requirement: Expert Angular knowledge, Optimization
Answer: AOT refers to an Ahead-of-time compilation. In Angular, it means that the code you write for your application is compiled at build time before the application is run in a browser. It‘s an alternative to Just-in-time compilation, where code is compiled just before it is run in the browser. AOT compilation can lead to better application performance.
Note: You should expect knowledge of JIT (Just-in-Time) compilation in Angular too when you hire Angular developers.
Question 23: What is an HTTP Interceptor?
Requirement: Middle Angular knowledge
Answer: Interceptor is just a fancy word for a function that receives requests/responses before they are processed/sent to the server. You should use interceptors if you want to pre-process many types of requests in one way. For example, you need to set the authorization header Bearer for all requests:
token.interceptor.ts
import { Injectable } from '@angular/core';
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
@Injectable()
export class TokenInterceptor implements HttpInterceptor {
public intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
const token = localStorage.getItem('token') as string;
if (token) {
req = req.clone({
setHeaders: {
'Authorization': `Bearer ${token}`
}
});
}
return next.handle(req);
}
}
And register the interceptor as singleton in the module providers:
app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HTTP_INTERCEPTORS } from '@angular/common/http';
import { AppComponent } from './app.component';
import { TokenInterceptor } from './token.interceptor';
@NgModule({
imports: [
BrowserModule
],
declarations: [
AppComponent
],
bootstrap: [AppComponent],
providers: [{
provide: HTTP_INTERCEPTORS,
useClass: TokenInterceptor,
multi: true // < - - - - an array of interceptors can be registered
}]
})
export class AppModule {}
Question 24: How many Change Detectors can there be in the whole application?
Requirement: Expert Angular knowledge
Answer: Each component class consists of its own Change Detector. All Change Detectors are inherited from AbstractChangeDetector.
Question 25: What change detection strategies do you know?
Requirement: Expert Angular knowledge
Answer: There are two strategies - Default and OnPush. If all the components use the default strategy, Zone checks the entire tree regardless of where the change occurred. To inform Angular that we will comply with the performance improvement conditions, we need to use the OnPush change detection cycle strategy. This will tell Angular that our component depends only on component input properties and any object that is passed to it should be considered immutable. This is all built on the Principle of the mile automaton, where the current state depends only on the input values.
Question 26: What is Change Detection, and how does the Change Detection Mechanism work?
Requirement: Expert Angular knowledge
Answer: Change Detection algorithm is the process of synchronizing a model with a view. In Angular, the flow of information is unidirectional, even when using the ng Model to implement two-way binding, which is syntactic sugar on top of a unidirectional flow.
Change Detection Mechanism-moves only forward and never looks back, starting from the root (root) component to the last. This is the meaning of one-way data flow. The architecture of an Angular application has a very simplified structure — the tree of components. Each component points to a child, but the child does not point to a parent. One-way flow eliminates the need for a $digest loop.
Note: Expect in-depth knowledge of the best practices involved in implementing design patterns with Angular.
Question 27: How do you update the view if your data model is updated outside the 'Zone'?
Requirement: Expert Angular knowledge
Answer:
- Using the ApplicationRef.prototype.tick method, which will run change detection on the entire component tree.
- Using NgZone.prototype.run method, which will also run change detection on the entire tree. The run method under the hood itself calls tick, and the parameter takes the function you want to perform before the tick.
- Using the ChangeDetectorRef.prototype.detectChanges method, which will launch change detection on the current component and its children.
Note: Expect an expert Angular developer to know about other popular methods like ngOnChanges, ngAfterViewChecked, ngDoCheck, and ngOnDestroy (cleanup before Angular destroys the component) since they are important.
Question 28: Why do we need lazy loading of modules and how is it implemented?
Requirement: Middle Angular knowledge
Answer: Lazy loading of modules is needed to break the code into pieces. When downloading the app in the browser, it doesn't load all of the application code. During the transition to the route with lazy loading, the module has to load the code into a browser.
Example for using lazy loading modules:
{ path: 'example', loadChildren: './example/example.module#ExampleModule', component: PublicComponent },
Question 29: What are Core and Shared modules for?
Requirement: Middle Angular knowledge
Answer: A Shared module serves as a generic module for all modules, components, directives, pipes, etc., which are not required to be in a single copy for the application but need to be imported into many different modules.
A Core module is a place to store services that you need to have in the form of a singleton for the entire application (for example, a user authorization service with data storage about it).
Question 30: What are some points to consider when optimizing an Angular 6 application for performance?
Requirement: Application performance optimization
Answer: There are many ways, some ideas include:
AOT compilation, bundling and uglifying the application, tree shaking, lazy loading, separating dependencies and devDependencies, Using OnPush and TrackBy, removing unnecessary 3rd party libraries and import statements, avoid computing values within the template.
Hire expert developers for your next project
Question 31: What are some important practices to secure an Angular application?
Requirement: Designing for security
Answer: Some basic guidelines include:
- Check that all requests come from within your own web app and not external websites;
- Sanitize all input data;
- Use Angular template expressions instead of DOM (document object model) APIs;
- Content Security Policies;
- Validate all data with server-side code;
- Compile using an offline template compiler;
- Avoid including external URLs in your application;
- Make JSON responses non-executable;
- Keep all libraries and frameworks up-to-date.
Note: Keep in mind the importance of managing angular templates effectively. Break a template into its HTML file, and load it using the “templateUrl” option. You don’t need to do this for very small templates.
Question 32: What‘s the difference between unit testing and end-to-end testing? What are some testing tools you would use for an Angular application?
Requirement: End-to-end and unit testing
Answer: Unit testing is a technique to test whether isolated segments of code are functioning properly. End-to-end testing involves checking that entire sets of components to make sure they are working together properly and that the application is working as you would expect. End-to-end tests often simulate user interactions to test that an app is functioning as it should. Jasmine and Karma are all great testing tools.
Question 33: What are the differences between promises and observables?
Requirement: Expert-level Angular knowledge
Answer: The differences are as follows:
Promises emit a single value. However, observables emit multiple values over time.
Promises aren’t lazy, however, observables are lazy. Observables are called only when there are subscriptions to them.
One can’t cancel a promise. However, the “unsubscribe()” method allows you to cancel observables.
Question 34: What are the differences between RouterOutlet and RouterLink?
Requirement: Expert-level Angular knowledge
Answer: The differences are as follows:
RouterOutlets are directives from the router library. RouterOutlets are placeholders. They are the spots in templates where the router displays the components for that outlet. RouterOutlets are components.
RouterLinks are directives on the anchor tags. They give the router control over the tags. Developers can assign string values to RouterLink directives.
Question 35: List the different router events in the Angular router.
Requirement: Expert-level Angular knowledge
Answer: The router events are as follows:
- NavigationStart;
- RouteConfigLoadStart;
- RouteConfigLoadEnd;
- RoutesRecognized;
- GuardsCheckStart;
- ChildActivationStart;
- ActivationStart;
- GuardsCheckEnd;
- ResolveStart;
- ResolveEnd;
- ActivationEnd;
- ChildActivationEnd;
- NavigationEnd;
- NavigationCancel;
- NavigationError.
Question 36: How will you make an Angular application render on the server side instead of the client side?
Requirement: Expert-level Angular knowledge
Answer: You can use a technology called Angular Universal to do this. Angular Universal is a tool as a part of Angular. It allows the server to pre-render the Angular app when a user visits the web app for the first time.
Question 37: Why would you “AsyncPipe” in Angular?
Requirement: Expert-level Angular knowledge
Answer: You can use “AsyncPipe” to subscribe to an observable or promise. It can then return the latest value emitted by the promise or observable. “AsyncPipe” marks an Angular component that has been checked for new values emitted.
Question 38: Categorize data binding types in Angular.
Requirement: Expert-level Angular knowledge
Answer: There are 3 categories of data binding types in Angular. They are as follows:
From-the-source-to-view: This uses one-way data binding.
From-view-to-source: It utilizes one-way data binding.
View-to-source-to-view: This category uses two-way data binding.
Question 39: State the different types of Angular filters.
Requirement: Expert-level Angular knowledge
Answer: The different types of Angular filters are as follows:
- Currency;
- Filter;
- Date;
- Lowercase;
- Uppercase;
- orderBy;
- JSON;
- Number;
- LimitTo.
Question 40: Mention a few activities that you can do using the Angular CLI.
Requirement: Expert-level Angular knowledge
Answer: You can use the Angular Command Line Interface (Angular CLI) to create components, add components, deploy components, test, create services to share data between components, and many more activities.
Question 41: Briefly explain the digest cycle process in Angular.
Requirement: Expert-level Angular knowledge
Answer: The digest cycle process in Angular helps to monitor the watch list. This process tracks changes in the watch variable value. You can use it to compare multiple previous and current values. The process includes a comparison between the previous and present versions of the scope model values.
Question 42: Describe the steps to create directives using the Angular CLI.
Requirement: Expert-level Angular knowledge
Answer: The steps to create directives using the Angular CLI are as follows:
- Use the following command to start a new project:
‘ng new [application-name]’ - Change the directory into a new directory by using the following command:
‘cd [application-name]’ - Generate a new directive by issuing the following command:
‘ng generate directive [path-to-directives/test-directive]’.
Question 43: What does the PipeTransform interface do in Angular?
Requirement: Expert-level Angular knowledge
Answer: The PipeTransform interface receives an input value. It then uses the “transform()” method to transform the input value into the desired format.
Example:
import { Pipe, PipeTransform} from ‘@angular/core’;
@Pipe({
name: ‘testpipe’
})
export class testpipePipe implements Pipe Transform {
transform(value: unknown,…args: unknown): unknown {
return null;
}
}
Question 44: What does “ng-content” does in Angular?
Requirement: Expert-level Angular knowledge
Answer: ”ng-content” in Angular inserts content dynamically inside components. You can reuse components better. “ng-content” passes content inside the component selector.
Question 45: What is “multicasting” in Angular?
Requirement: Expert-level Angular knowledge
Answer: Assume that you want to fetch data by communicating with a backend service by using the HttpClient module. You want to broadcast the data to multiple subscribers. Assume that you want to do all of these tasks in one execution. “Multicasting” refers to responding to multiple subscribers with data.
Question 46: What will happen if you don’t provide handlers for some notification types?
Requirement: Expert-level Angular knowledge
Answer: The Angular observers ignore all notification types that don’t have handlers. The observer instance will publish a value only if there’s a subscription.
Question 47: What is RouterState in Angular?
Requirement: Expert-level Angular knowledge
Answer: The RouterState in Angular is a tree of activated routes. Nodes in this structure know the “consumed” URL segment. These nodes also know about the extracted parameter and resolved data. One can use the router service and routerState property to access the current RouterState.
Open-Ended Questions
Once you‘ve established that your developer is an expert with some Angular coding interview questions, you should ask some less technical questions. These should spark a discussion and you should tailor them to fit your own job requirements. Don‘t be afraid to ask follow-up questions!
Question 48: Describe a time you fixed a bug/error in an application. How did you approach the problem of ? What debugging tools did you use? What did you learn from this error handling experience?
Requirement: Debugging, Breaking down a problem into parts
Debugging is one of the key skills for any software developer. However, the real skill is in breaking the problem down in a practical way rather than finding small errors in code snippets. Given that debugging often takes hours or even days, you don‘t have time in an interview setting. Asking these questions will give you an idea of how your candidate approaches errors and bugs.
Answer: In the candidate‘s response you should look out for things like:
- A measured, scientific approach;
- Breaking down the problem into parts;
- Finding out how to reproduce the error;
- Expressing and then testing assumptions;
- Looking at stack traces;
- Getting someone else to help/take a look;
- Searching the internet for others that have had the same problem;
- Writing tests to check if the bug returns;
- Checking the rest of the code for similar errors;
- Turn problems into learning experiences.
Question 49: What's the most important thing to look for or check when reviewing another team member's code?
Requirement: Mentoring less experienced developers, Leadership skills
Answer: Here you‘re checking for analysis skills, knowledge of mistakes that less experienced developers make, keeping in mind the larger project, and attention to detail.
A good answer might mention code functionality, readability and style conventions, security flaws that could lead to system vulnerabilities, simplicity, regulatory requirements, or resource optimization.
Question 50: What tools & practices do you consider necessary for the Continuous Integration and Delivery of an Angular application?
Requirement: DevOps systems design, maintain, and upgrade applications
Hiring Angular developers
How do you hire competent Angular developers? The above interview questions will certainly help. However, depending on the local labor market, you could still find it hard to find competent people.
After all, you will need much more than just Angular skills, won’t you? You need professionalism, a deep knowledge of coding best practices, and an ability to collaborate with the larger team.
Need help hiring experienced Angular developers? We at DevTeam.Space can help! Read our guides “How to find a good software developer” and "How to hire a remote software developer for a successful product" for more information.
In Summary
Hiring the right people for your development team is critical to the success of your project. Remember that you should not be aiming to hire the perfect Angular developer, but rather the right person for the job at hand.
With the help of this information and sample interview questions on Angular, you can ensure that the hiring process goes smoothly and successfully - allowing you to hire a great programming team to get your project completed on time and on budget.
Finally, here is a BONUS for you:
Click here to download a ready-to-use cheat sheet of questions and answers. Simply print it out and bring it with you to the interview.
Happy Hiring!