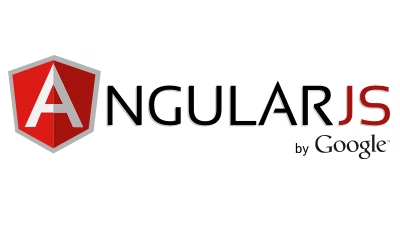
How to Build a Simple AngularJS Project for Beginners?
Wondering how to build a simple AngularJS Project for beginners?
If you are then you have come to the right place.
AngularJS currently powers over 350,000 websites around the world. This makes it a very common and reliable web framework.
Using Angular JS, you can build powerful and exciting applications that can help you make a big splash in your industry.
So you want to start building your first AngularJS app, but you don‘t know where to start. AngularJS is notorious for being difficult to learn. Most “AngularJS projects for beginners” manuals get insanely complicated very quickly.
Add to this the annoying Jargon words that get thrown in as if they are common knowledge, and you have what might seem like an insurmountable challenge.
In this guide, I‘ll make getting started as simple as possible. First I‘ll go through some of the difficulties of building web applications, and how AngularJS helps us solve some of those problems.
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
Then, we‘ll look at some AngularJS project examples, and how to create an AngularJS project from scratch.
If you‘re only interested in building the Angularjs project, you can skip ahead to the coding section.
Web App Challenges
Every website uses a client-server architecture. This means there are two main parts - your web browser, and the server that hosts the website. You go to a URL (the location of the server), and the server hands your browser the information it needs - the HTML, CSS, and Javascript - to display the web page.
Pretty simple so far. You can interact with the page by clicking buttons and links on the page. When you do, you are basically requesting a whole new page from the server.
This used to be fine when web pages were mainly static documents. Nowadays, web pages can be full applications, with interactive user interfaces.
This is where our traditional model runs into problems. Every time you click a button, the whole page is reloaded. Even the parts that don‘t need to change. This makes a page seem slow and unresponsive to the user.
Therefore, we need a different way of building web applications. One where each time a user interacts with a page, only new information is requested from our server. The rest of the page is left untouched.
Single Page Web Applications
The solution is to build a Single Page Application (SPA). When you visit a SPA website, your browser is sent only a single page. Then, only parts of the page are dynamically updated and manipulated in real-time. This results in a much faster and nicer feeling web app.
Many businesses use this approach for their web app projects, including large companies and startups. An example of a Single Page Application is the USA Today website. Go there and try to guess which parts are being reloaded when you click around.
Where Does AngularJS Fit In?
AngularJS is a front-end framework for building these Single Page Applications. It helps extend our HTML to be more dynamic, and suitable for building these sorts of applications. It‘s open-source and was built by independent developers, with support from Google.
By using Angular, we get a whole framework of tools to help us build better web applications.
For example, AngularJS, by separating the views from the logic of your application, helps us build more maintainable and testable code.
What You Should Know For This Tutorial?
Before we start to create the Angularjs project from scratch, I‘m assuming you know a little bit about each of the following
- HTML
- CSS
- Javascript Frameworks such as jQuery
AngularJS Important Concepts
There are a few important concepts you need to understand before you build your own AngularJS app. I‘ll give a brief overview and some links to more detailed information.
Model-View-Controller (MVC)
MVC is a design pattern for implementing user interfaces. It helps us separate the logic in our code from how it is displayed. By doing this, we end up with a much neater, more testable application. If you aren‘t familiar with MVC, check out this interesting explanation using lego.
2-Way Data Binding
With jQuery, making parts of our page to automatically update when other information is changed can be a hassle. AngularJS has an awesome feature called 2-way data binding. It allows you to bind things together, so that when one thing updates the other one does too - instantly.
We‘ll be using this concept in our application.
Directives
I said before that AngularJS extends HTML. It does this through directives.
AngularJS directives are HTML attributes that start with ng-. These tell Angular to attach a specific behavior to that HTML element. For example, our app is going to have
<html ng-app>
This is a directive that tells Angular to treat our HTML page as an application.
Building a Sample AngularJS Project
Now, we are ready to learn how to build the first AngularJS app. We are going to build probably the most simple AngularJS project possible.
Hire expert developers for your next project
1,200 top developers
us since 2016
All it is going to do is ask for your name. Then, as you type your name, it is going to display what you write on a different line.
Sounds super simple right? Yes. But, it does introduce us to some of the important AngularJS basics such as 2-way data binding and directives.
Setting Up
Our project is going to be about as simple as you can get in AngularJS. We are only going to have one file called index.html.
Because we aren‘t going to be using a server or any other Javascript or CSS files, you can just create this file anywhere on your computer, then open it in a web browser to test it out.
index.html
Firstly, to get our bearings, let‘s set up a very basic HTML structure for our page. I‘m going to call mine index.html. Here is the code.
<!doctype html>
<html>
<head>
</head>
<body>
<div>
<label>Name:</label>
<input type="text" placeholder="Enter a name here">
<hr>
<h1>Hello! Your Name</h1>
</div>
</body>
</html>
This doesn‘t do anything interesting (yet), but it gives us an idea of the structure of our page. Next, we need to add some functionality using AngularJS.
Adding in AngularJS
Now, we can start adding some AngularJS into our HTML. Firstly we‘ll need to get the AngularJS framework into our project.
The easiest way to do this is to use the hosted version. All you need to do is include these script tags between your head tags, like this:
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.2/angular.min.js"></script>
</head>
Now we‘ve got AngularJS installed in our app, we‘ll need to start adding in some Directives to let Angular know what to do. Remember those are ng- tags I was talking about before.
Adding Directives
In the <html>
tag, simply add ng-app
.
<html ng-app>
This is a directive (as I explained before) that tells AngularJS to be active on this section of our HTML. In our case, it‘s the whole HTML document.
Binding Data Together
Here‘s where we get a glimpse at the power of the AngularJS framework.
We want our “Hello! Your Name” text to actually display the name we type, as we type it. With jQuery, we would have to set up some sorts of functions and listeners. Luckily, this is exactly where AngularJS makes things super simple.
What we need to do is bind the data from our text input field to the text in the “Hello! Your Name” field. This will mean one automatically updates when the other is changed.
To do this, first, we add the ng-model="yourName"
to our <input>
tag. This tells AngularJS that the data we type is called “yourName” and we want to bind it to something else.
<input type="text" ng-model="yourName" placeholder="Enter a name here">
Next, we need to tell Angular what to bind it to. Replace the “Your Name” text in the <h1>
tags with {{yourName}}
like so.
<h1>Hello! {{yourName}}!</h1>
The {{ }}
are how you declare where to bind something in AngularJS. Now, rather than just displaying hard-coded text, Angular will automatically update this text whenever yourName changes. Awesome.
Test It Out
That‘s it! You‘ve just created your first AngularJS project, including 2-way data binding, directives, and even using a simple MVP architecture. Congratulations.
Whatever you type into the name field should automatically be displayed below.
When you put it all together, it should look something like this.
Hire expert developers for your next project
And the complete code:
<!doctype html>
<html ng-app>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.2/angular.min.js"></script>
</head>
<body>
<div>
<label>Name:</label>
<input type="text" ng-model="yourName" placeholder="Enter a name here">
<hr>
<h1>Hello! {{yourName}}!</h1>
</div>
</body>
</html>
What Next
You know how to develop a simple AngularJS application. All you need to do now is step up the complexity a little bit. Check out AngularJS.org for a couple more simple apps like this. They also have some video tutorials on how to build them.
Other than that, just start experimenting with making your own custom web apps!
Getting an AngularJS application developed
If you‘ve gone through the tutorial and learning AngularJS seems a little too much for you, don‘t worry. There are plenty of developers around that can develop your app for you.
AngularJS is very popular, so you can usually find great developers for a good price. If you need a functioning web app, the cost of hiring developers might be easier than learning the process of building one yourself.
Finding developers with great experience in developing Angular Projects
Look for a trustworthy software development company that has considerable experience in developing Angular apps. You will find experienced developers there. At DevTeam.Space, we have the necessary Angular expertise.
Judge our capabilities by reviewing the following projects that we executed:
1. An eSports tournament project where we used Angular and Node.js
In this web app development project, the DevTeam.Space team worked on both the client-side programming and backend development. The eSports tournament app would allow players to set up tournaments for popular eSports games. Our team used Angular and Node.js, furthermore, we used various APIs, e.g., PayPal, Stripe, etc.
2. Adventure Aide: A tourism and recreation Angularjs project
The DevTeam.Space development team took over a project that was left incomplete by the earlier developers including freelancers. In addition to refactoring the source code completely, we rebuilt the application architecture.
Instead of two applications planned earlier, we developed one unified application named Adventure Aide. The app allows users to book outdoor adventures. We used Angular, Java, and MariaDB, furthermore, we utilized the AWS cloud platform. Furthermore, we integrated APIs from Google Maps, Stripe, etc.
3. Starshell: Keeping students safe on social media
Starshell is an app to monitor sensitive social media content to keep students safe on social media. Observers will monitor the content of the social media account of students depending on their consent.
The DevTeam.Space development team used Angular, Python, MongoDB, PyMongo, Flask, TensorFlow, etc. in this project. We also used APIs of key social media platforms like Facebook, Twitter, Instagram, etc.
4. MyTime: A scheduling, payment, and customer engagement app
Our DevTeam.Space development team has developed MyTime, an Angular app. This app enables multi-location chains and franchises to achieve greater operational efficiencies and customer satisfaction.
This was a high-stakes project, where our skills and commitments helped the client to meet stringent deadlines. Our expertise in developing web pages was important. Our expertise in TypeScript, JavaScript, PHP, plugins, etc. made a positive difference in this project.
5. A learning solution for the Chinese market
We, at DevTeam.Space, have developed a learning solution for the Chinese market. It’s an e-learning platform with features like scheduling classes, conducting online classes, collaboration, etc. Our deep knowledge of the following was useful in this project:
- AngularJS and Angular 2;
- Dependency injection;
- JSON, markup, etc.;
- Bootstrap development;
- Tools like GitHub;
- Document Object Model (DOM);
- Directives like ng-controller, ng-repeat, etc.;
- Angular input types.
6. The “Sidekick” education platform
The DevTeam.Space development team has created “Sidekick”, a project-based learning platform using Angular. This platform enables students to work with community partners to solve real-life problems using their skills. It enables teachers to cover modern skills, furthermore, teachers can evaluate students using this platform.
We utilized our skills in tools like Karma. Our knowledge of “script src”, routing, etc. helped us in this project. The project utilized our knowledge to initialize an Angular directive. Our knowledge of Angular syntax helped us, furthermore, our expertise in making a web app load faster on a web browser was useful.
Frequently Asked Questions on AngularJS Project
You should start your AngularJS project by reading AngluarJS’s conceptual overview, which can be found on its website. Next, do the AngularJS tutorial which will walk you step by step through the process. The next step is to download the Seed App project template which you can use to create your application.
Unless you are planning to create a simple AngularJS project, in which case you should complete the AngluarJS tutorial before you start creating your project, the process is to create a project specification plan in order to onboard the development team or developer that will build your project for you.
Provided that you have a reasonable understanding of coding and how to build a software application, building a simple application is achievable by even the most inexperienced person. However, if you want to build the kind of AngularJS product that will attract users and make money then you will most likely need full-time developers to help you.