- Developers
- Developer Blog
- Blockchain Development
- How To Build Your Own Blockchain Using Node.js
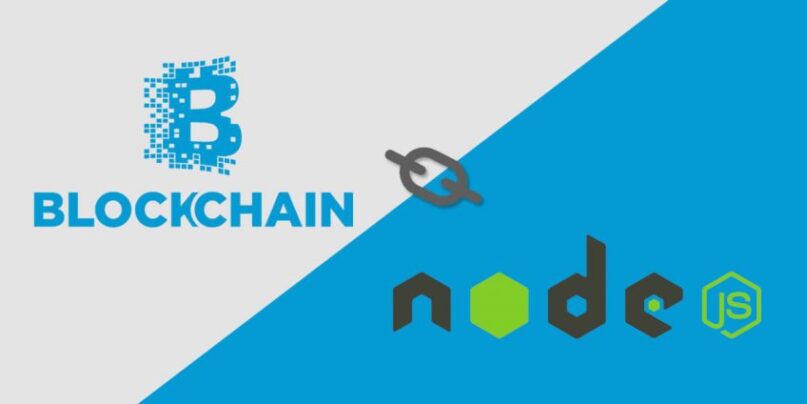
profile

By Aran Davies
Verified Expert
8 years of experience
Aran Davies is a full-stack software development engineer and tech writer with experience in Web and Mobile technologies. He is a tech nomad and has seen it all.
Want to know how to build your own blockchain using Node.js?
This is a great question which we will answer here.
What is Node.js: An introduction
Node.js is a highly popular runtime environment, with famous apps like Twitter, PayPal, and LinkedIn using it. Developed by Ryan Dahl, and first released in 2011, Node.js uses V8, which is Googles‘ open-source JavaScript engine. Node.js offers the following advantages:
- js enables asynchronous event-driven programming, which eliminated blocking processes. This improves scalability.
- It‘s a highly performant runtime environment.
- Developers with JavaScript expertise can easily learn Node.js, and it improves their productivity.
- Data streaming is easier to implement with Node.js.
- Thousands of sharable open-source modules and tools enrich the ecosystem, which also boasts of a vibrant developer community.
- Open-source NoSQL databases like MongoDB use JavaScript, therefore, a javaScript developer can easily implement these.
Read about these advantages in “10 great tools for Node.Js software development”.
Approaches for blockchain development with Node.js
As you can see from the above overview of blockchain and various platforms, there is a lot to plan for when undertaking blockchain development. These are as follows:
- Determine whether you need blockchain.
- Decide whether you need a crypto token.
- You need to choose between public vs private blockchain.
- There is a need to choose the right platform, which will expedite your project.
- You need to select the right tools for your development.
Read more about these aspects in “What to plan for when undertaking blockchain software development?”.
For blockchain development using Node.js, I recommend the following approaches:
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
- Use Lisk, if you are developing a DApp on a public blockchain.
- Use Fabric if your development project is in an enterprise environment.
Approach #1: Blockchain development on Lisk
You can use Lisk to develop a public blockchain DApp that utilizes Node.js, as follows:
Step #1: Build your development team
You need the following roles in your team:
- Business analysts;
- UI/UX designers;
- JavaScript developers;
- Solidity smart contract developers;
- Testers;
- A project manager (PM).
Step #2: Buy Lisk crypto tokens
You need to buy Lisk (LSK) crypto tokens to develop an app on the Lisk platform. This involves the following steps:
- You need to download and install the “Lisk Hub” desktop wallet. This offers secure storage for your Lisk tokens. You can send and receive Lisk easily. This also makes it easier for you to vote in the Lisk network decision-making process.
- Use “Lisk Hub” to create your Lisk ID and initialize your Lisk account.
- Buy Lisk from a crypto exchange. You can get a list of crypto exchanges selling Lisk in “5 best websites to buy LISK cryptocurrency at cheapest price”.
Step #3: Set up “Lisk Core”
“Lisk Core” is the program for implementing the “Lisk Protocol”. The following quick facts are relevant here:
- “Lisk Core” uses Node.js as the engine for code execution.
- It uses the PostgreSQL database to store all information on the Lisk main network.
- It also uses Redis as the database to cache API responses.
Read more about it here.
Your development project on Lisk requires you to run a Lisk node, and “Lisk Core” lets you do that. This requires the following steps:
- You need to set up “Lisk core”, by following the instructions here.
- Follow the “Lisk Core” user guide to know how you can do the required administration, configuration, and monitoring tasks.
Step #4: Set up “Lisk Commander”
“Lisk Commander” is a “Command Level Interface” (CLI) to use the “Lisk Core” APIs. You will use it in the development process. Take the following steps:
- Set it up using the instructions here.
- Check out their user guide. It covers the configuration, the list of commands, and the general usage of “Lisk Commander”.
Step #5: Set up “Lisk Elements”
“Lisk Elements” is a JavaScript library, which has quite a few Node.js packages that you can install separately. You can cover several functionalities in your app using these packages, e.g.:
- Cryptographic functions;
- Passphrase helpers;
- Creating, signing, and verifying transactions.
Set up “Lisk Elements” using the instructions here.
Hire expert developers for your next project
1,200 top developers
us since 2016
Step #6: Develop, test, and deploy your app
Now that you have installed the required Lisk SDK components, you can create your app. This involves the following steps:
- Build your sidechain specifically for your app.
- Use “Lisk Commander” to interact with the sidechain.
- Utilize appropriate modules from “Lisk Elements” to build your app functionalities.
- I assume you have coded Solidity smart contracts outside Lisk by now. You need to integrate them with your app. Max Kordek, one of the founders of Lisk has explained how to integrate Smart contracts with an app on Lisk. Read “What is Lisk? And what it isn‘t.” for more information.
- Use LSK stored in “Lisk Hub” to implement your app, and for other operations.
You can use the extensive documentation provided by Lisk, for further guidance. This completes blockchain development using Node.js on Lisk.
Approach #2: Blockchain development using Node.js on Fabric
I will now explain blockchain development using Node.js on Hyperledger Fabric. This approach involves the following steps:
Step #1: Form a development team
Like the earlier approach, your team needs BAs, UI designers, testers, Node.js developers, and a PM. Additionally, you need Fabric developers.
Step #2: Choose a “Blockchain as a Service” (BaaS) platform
As I had mentioned earlier, a key advantage of Fabric is that several technology giants offer it as part of their BaaS platforms. I recommend you use “IBM Blockchain Platform”. Following are a few relevant facts about this BaaS platform:
- It expedites the development since IBM handles the blockchain infrastructure-related complexities.
- You can get all features of Fabric, e.g., the pluggable architecture, “channels” for confidential transactions, SDKs, improved security, etc.
- The platform offers sample codes and tools.
- Governance tools will help you in managing the network rules, scaling, and setting up membership.
- This platform uses Kubernetes, the renowned open-source orchestration system for deployment, scaling, and application management. This makes deployment easier.
Sign-up for “IBM Blockchain Platform” here.
Step #3: Familiarize yourself with the Fabric Node.js SDK
Fabric offers a Node.js SDK for blockchain app development. The important facts about this SDK are as follows:
- You can access this comprehensive documentation for setting up this SDK.
- The SDK works with a Node.js runtime, and it provides robust APIs to communicate with the Fabric blockchain network.
- There is robust documentation to help you with network configuration using this SDK. You can access it here.
- Developers can use the SDK to submit transactions to Fabric chaincodes, i.e., smart contracts in Fabric parlance.
- The SDK offers querying features for smart contracts. Various queries are possible, e.g., block-by-number, block-by-hash, transaction-by-id, etc.
- The client component of the SDK enabled the creation of new channels for confidential transactions. You can access this tutorial to know more about it.
- A “Peer” is a kind of node on Fabric with a specific role in the consensus algorithm. Developers can use the Node.js SDK to send channel information to peers so that they can join the channel.
- The SDK helps with installing chaincodes on peers and instantiating them.
- Monitoring various events like block events and transaction events is possible with this SDK.
- The SDK enables easy registration of new users and revoking of existing users.
This is not an exhaustive list of the features. Read more about the Fabric Node.js SDK here.
Step #4: Use the Fabric Node.js SDK on “IBM Blockchain Platform”
Using the Fabric Node.js SDK on the “IBM Blockchain Platform” involves the following:
Hire expert developers for your next project
- Choose the right “IBM Blockchain Platform” offering according to your requirements. There are various options, e.g., enterprise plan, “IBM Blockchain Platform” for AWS, etc. Read about them in “Getting started with IBM Blockchain Platform”.
- Deploy “IBM Blockchain Platform” in accordance with the offering you chose.
- Install cURL for downloading Fabric sample code, by following the instructions here.
- Install Node.js runtime and NPM. You can use these instructions.
- Install Fabric samples, using these instructions.
- Use the network monitor to install the chaincodes from the samples most suited to your requirements. Follow these instructions to do this.
- Code your app using the Fabric Node.js SDK.
- Add network API endpoints to your app.
- Prove the authenticity of your app by enrolling it.
- Register your app using the client-side certificates you generated during the enrolment.
You can now execute various functions by invoking and querying chaincodes. Read detailed instructions in “Developing applications with the Fabric SDKs”. This completed blockchain development using Node.js on Fabric.
Exploring Node.js for an important blockchain development project?
Blockchain technology is new, and blockchain development can be tricky. Node.js is a relatively new option for blockchain development, which is why it’s essential to hire experienced Node.js developers who will be able to build a reliable product.
You need to carefully choose the right platform. Moreover, need to hire a capable team with several niche skills. You might need to engage a professional development partner for projects like this. Check our guide “How to find the best software development company?” before you select a partner.
If you are still unsure, contact DevTeam.Space via this form describing your initial requirements for a blockchain project. One of our technical managers will get back to you to link you with experienced node.js and blockchain developers.
Frequently Asked Questions
The initial block in a blockchain is called the genesis block. It is hardcoded and a new block is created on this first block. A valid transaction data or block has a unique cryptographic hash, the previous block’s hash, and the timestamp of verified transactions. The data contained in all the blocks include an encrypted hash based on some cryptographic function. It includes the previous hash value from the previous block’s data and the current block hash.
- Ethereum
- IBM blockchain
- Ripple
- OpenChain
Node.js is a JavaScript runtime environment that allows JavaScript code to be run outside of a web browser.
Every block has the stored hash value of the previous block and if a node is missing the correct hash value, it makes the entire blockchain invalid. The standard cryptographic hash function such as SHA256 is used by a block for encryption of both its own hash and of a prior block.
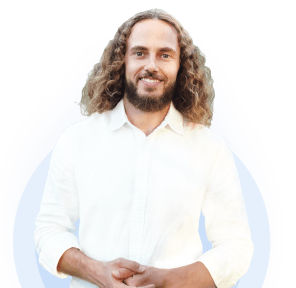
Alexey Semeney
Founder of DevTeam.Space
Hire Alexey and His Team To Build a Great Product
Alexey is the founder of DevTeam.Space. He is award nominee among TOP 26 mentors of FI's 'Global Startup Mentor Awards'.
Alexey is Expert Startup Review Panel member and advices the oldest angel investment group in Silicon Valley on products investment deals.