- Developers
- Developer Blog
- Software Development
- List of Docker Commands with Examples
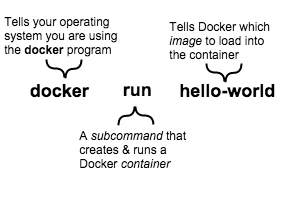
profile

By Sam Palmer
Verified Expert
1 year of experience
Sam is a professional writer with a focus on software and project management. He has been writing on software-related topics and building PHP based websites for the past 12 years.
Looking for a list of Docker commands with examples?
You’ve come to the right place.
Docker is becoming a popular technology for distributing and deploying web apps. It makes the whole development operations process easier, faster, and cheaper – all while reducing the number of servers needed. Why wouldn’t you want to use it?
I’m going to go through some of the most common Docker commands with examples and explain what they do, so you can start experimenting with them right away. We’ve already discussed Docker, its benefits, and some basic commands in a previous blog post.
Key Terms related to docker commands
Firstly, let’s get clear up some docker jargon terms to make sure you know what I’m talking about with each command:
- Container – These are what docker is built on. They encapsulate an application and all of its libraries and dependencies, so it can be run anywhere Docker is installed. There are other containerization platforms, so check out how Docker stacks up against Kubernetes.
- Image – A Docker Image is a file that is essentially a snapshot of a container. You can create a container by running a Docker Image
- Docker Hub – A public registry for developers to distribute their code.
- [OPTIONS] – Here’s where you can add options to your commands to get different results. Each command has lots of options associated with it. Refer to the documentation for all the details.
- [COMMAND] – Here’s where you put the parameters for your command. Depending on the command, it might be a file name or a container ID.
- [ARG…] – Here is where you can write additional arguments for a command.
Docker Terminal Command Basics
Every command has a basic structure. Here’s an example command:
Important Docker Commands List
Here are some commands to try out on the command line. Just make sure you have Docker running!
docker run
Description: docker run is probably the most important command on this Docker list. It creates a new container, then starts it using a specified command. It is the equivalent of using docker create
and then immediately using docker start
(we‘ll go through those below).
Usage: docker run [OPTIONS] IMAGE [COMMAND] [ARG...]
Example: docker run hello-world
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
This example will download, create and run the ‘hello-world’ container from the docker hub. Try it out!
docker create
Description: This command creates a new container and prepares it to run the specified command, but doesn’t run it. The ID is printed to STDOUT. This command is similar to docker run
but the container is never started. It can be started at any time with docker start <container_id>.
This can be useful if you want to create and prepare a container for running at a later time.
Usage: docker create [OPTIONS] IMAGE [COMMAND] [ARG...]
Example: docker create hello-world
This will download and create the hello-world container same as above, but it won’t run it.
docker start
Description: Starts container, either created with docker create
or one that was stopped. More info.
Usage: docker run [OPTIONS] IMAGE [COMMAND] [ARG...]
Example: docker start hello-world
docker stop
Description: Stops a running container. More info.
Usage: docker stop [OPTIONS] CONTAINER [...]
Example: docker start hello-world
This example will stop the container we started in the example above. You can use docker start
to start it back up again.
docker ps
Description: Use this command to list the docker containers available. By default, only running ones are listed, but you can add options such as -a to get a docker container list. More info.
Usage: docker ps [OPTIONS]
Example: docker ps -a
The above command will display all containers on your system. The output will look something like this:
docker rm
Description: Removes a container. Once you use docker ps
to list available containers, you can use this command to remove one or more of them.
Usage: docker rm [OPTIONS] CONTAINER [CONTAINER...]
Example: docker rm -f redis
Hire expert developers for your next project
1,200 top developers
us since 2016
This example will remove the container called ‘redis’. The -f
tag forces the action, meaning the container will receive a SIGKILL
command first.
docker rmi
Description: Docker RMI command stands for remove image. Similar to docker rm
but removes the docker image rather than a running instance of an image (called a container).
Usage: docker rmi [OPTIONS] IMAGE [IMAGE...]
Example: docker rmi test
This example will remove the image name ‘test’. Use the docker images
command to get a list of images and ids.
docker login
Description: Let’s you login to a docker registry. You can use this command to log into any public or private registry. You’ll probably use this to log into Docker Hub.
Usage: docker login [OPTIONS] [SERVER]
Example: docker login localhost:8080
Here we are logging into a self-hosted registry by specifying the server name. Otherwise, just use docker login
to log into your Docker Hub registry.
docker tag
Description: Use this command to create a tag TARGET_IMAGE that refers to SOURCE_IMAGE.
Usage: docker tag SOURCE_IMAGE[:TAG] TARGET_IMAGE[:TAG]
Example: docker tag 7d9495d03763 maryatdocker/docker-whale:latest
Here we are tagging a local image with ID “7d9495d03763″ into the “maryatdocker/docker-whale” repository with the tag “latest”
docker push
Description: docker push is the command you’ll use to share your images to Docker Hub or another registry. It’s a key part of the deployment process, and you’ll need to use it with docker tag
and docker login
to work properly.
Usage: docker push [OPTIONS] NAME[:TAG]
Example: docker push maryatdocker/docker-whale
This example will push the image we tagged above to the repository. It’s actually the example from the docs, check out the full page here.
Combine it with the tag command like this:
docker exec
Description: The exec command can be used to run a command in a container that is already running. docker run
lets us run a command when starting a container, and docker exec
can be used to feed subsequent commands to a container.
Hire expert developers for your next project
Usage: docker exec [OPTIONS] CONTAINER COMMAND [ARG...]
Example:
First, start a container with docker run --name ubuntu_bash --rm -i -t ubuntu bash
Now we can execute a command on that container to create a new file with docker exec -d ubuntu_bash touch /tmp/execWorks
docker container logs
Description: Getting deeper into using docker, the docker logs
command lets you see the log file for a running container. However, it can only be used with containers that were started with the json-file
or journald
logging driver
Usage: docker logs [OPTIONS] CONTAINER
Example: docker logs -f MyCntr
docker kill
Description: Sometimes you just need to kill one or all running containers. Similar to SIGKILL
(this is actually the message it will receive), docker kill
will stop a container from running.
Usage: docker kill [OPTIONS] CONTAINER [CONTAINER...]
Example: docker kill MyCntr
docker swarm
Description: Use docker swarm
to manage a swarm. You can initialize a swarm, add manager and worker nodes, and update a swarm. This command is actually a whole bunch of commands in one – take a look at all of the child commands here.
Example: docker swarm init --advertise-addr <MANAGER-IP>
This will initialize a swarm and give us back the address to add workers to the swarm later. For a better understanding of how to use docker swarms, take a look at the create a swarm tutorial in the docs.
Start Experimenting with these docker commands!
This docker reference should be enough to get you started using docker on the command line. Signup for a Docker hub account if you don’t have one and have a go at deploying your own app. Once you get used to the process, you’ll never go back to your old deployment habits.
If you want experienced software developers to handle your software product development and deployment and do not find such talent in your project team, partner with a reputed software development agency.
DevTeam.Space is one such community of field-expert software developers. You can outsource these vetted and managed software developers by writing to us your project requirements. One of our account managers will get back to you for further guidance on the process.
Frequently Asked Questions on docker commands
Docker is a cloud-based PaaS platform that allows OS-level virtualization.
Docker run command allows a container to be created from an image so that it can be run.
Docker can be run either in default ‘attached mode’ or in ‘detached mode’. The attached mode runs the program in the foreground while detached runs it in the background.
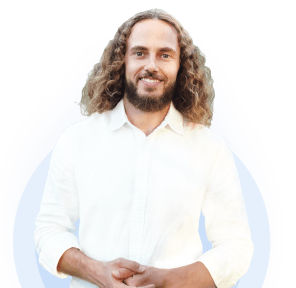
Alexey Semeney
Founder of DevTeam.Space
Hire Alexey and His Team To Build a Great Product
Alexey is the founder of DevTeam.Space. He is award nominee among TOP 26 mentors of FI's 'Global Startup Mentor Awards'.
Alexey is Expert Startup Review Panel member and advices the oldest angel investment group in Silicon Valley on products investment deals.