- Developers
- Developer Blog
- Blockchain Development
- How to Deploy Contract Ethereum?
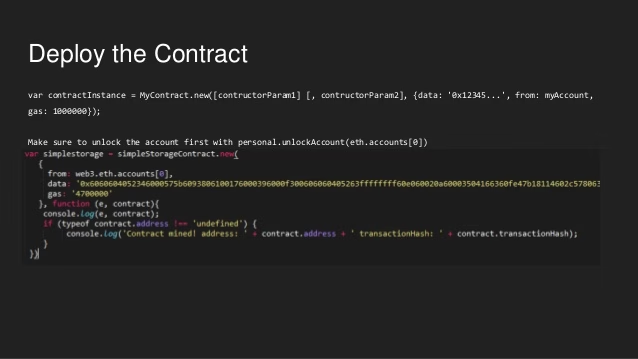
profile

By Aran Davies
Verified Expert
8 years of experience
Aran Davies is a full-stack software development engineer and tech writer with experience in Web and Mobile technologies. He is a tech nomad and has seen it all.
Want to know the process of deploying smart contracts? In this article, we will discuss how to deploy smart contracts on ethereum.
Knowing how to deploy a smart contract on Ethereum is a must if you want to create your own powerful blockchain-based solution.
Deploying smart contracts onto the Ropsten testnet using MetaMask and Remix IDE:
The first approach I describe for deploying smart contracts in Ropsten using MetaMask and Remix tools. I refer to a sample project that Merunas Grincalaitis undertook to convert a web app to a DApp.
He described his project in the article “Ultimate Guide to Convert a Web App To a Decentralized App Dapp”. I will refer to this article for commands and code details while outlining my approach. Let’s call it “Reference Article 1”.
Install MetaMask:
This is a tool that allows you to communicate with the blockchain via your smart contracts, even if you’re not running a full Ethereum node. You only need to get a browser extension, for which you need to visit the MetaMask website. Get Chrome or Firefox extensions.
After you install the extension, you will find a little icon in the top right corner of your browser. Click that, accept their terms & conditions, and set a password. You will have a 12-word private seed, which you need to retain since you will use it later.
Connect to Ropsten testnet:
You are now connected to the Ethereum mainnet in MetaMask, however, I will use a testnet connection so I can test smart contracts. You too should change the connection to a test blockchain.
There are lightweight Public Ethereum testnets like ethereumjs-testrpc. They are good for early-stage smart contract development and testing. In this guide, I will use Ropsten, which is a heavyweight Ethereum testnet.
Ethers in Ropsten don’t have any real value so you don’t have to spend any real money. Connect to Ropsten by clicking the MetaMask mainnet button, and change it to Ropsten. You have now completed your MetaMask set-up.
See reference article 1 for step-by-step instructions on how to do this.
Code smart contract using Remix IDE:
Remix integrated development environment (IDE) is a helpful tool that you can use to write smart contracts. It’s a web-based DApp so you don’t need to install any tool. You can access it directly on the Remix website.
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
After you have coded a smart contract in the IDE, you will need to deploy it.
Deploying smart contracts to the Ethereum testnet:
You need real Ether for deploying a smart contract in the Ethereum mainnet, however, for deploying in Ropsten you need to get Ropsten test Ether. Visit MetaMask faucet and request 1 Ether.
Keep in mind you will need an Ethereum wallet and that the Ethereum gasprice can change. While there is no gas limit, the Ethereum network does sometimes reach its maximum capacity so delays to smart contract processing do happen.
During the installation of the MetaMask browser extension, it added Web3.js to your browser. This is an Ethereum JavaScript API. Web3.js library allows you to interact with a local or remote Ethereum node. Read more about it in the web3.js reference documentation.
You will now use Web3.js. For this, ensure that the connection you had created from your MetaMask account to Ropsten is still open.
Return to the Remix IDE, and find the small ‘+’ sign at the top left of your browser. This is labeled as ‘Create’. Click that to deploy your smart contract to Ropsten, and confirm the transaction in MetaMask. Check reference article 1 for detailed instructions with screenshots.
You have now successfully deployed contract in Ropsten testnet using MetaMask.
Smart contracts deployment using Geth and Solidity compiler onto the Rinkeby testnet:
For this second approach, I will detail smart contract deployment using Geth and Solidity code compiling tools. Solidity is the main Ethereum smart contract programming language. It is possible to use other languages such as Python but Solidity was created especially with smart contracts in mind.
I will describe deployment in a different Ethereum testnet called Rinkeby. You can browse this testnet on the Rinkeby website.
I will reference an article published by the Mercury Protocol team in Medium.
Read the full article: “How To: Deploy the Smart Contract Onto The Ethereum Blockchain”. I will refer you to this article for commands, hence I will call it “Reference article 2”.
Install Solidity compiler (solc):
For this approach, you need to install the Solidity compiler (solc). Solc is a command line compiler for Solidity, and it’s built using C++. This is useful because it creates opcodes as outputs that the Ethereum Virtual Machine (EVM) can interpret.
Read more about solc in “Solidity: Using solc on Windows”.
I recommend that you install solc using ‘npm’, i.e. the default package manager for the JavaScript runtime environment Node.js. Read more about npm in the npmjs documentation. Installing solc using npm is very easy.
You need to first install Node.js and npm, and you can do that from their package installation page. The command to install solc with npm is: “npm install -g solc”.
Install Geth:
Geth is a command line interface (CLI) used to run a full Ethereum node, and it’s implemented in the “Go” language. It offers the following interfaces:
- CLI subcommands and options;
- A JSON-rpc server;
- An interactive console.
Read more about Geth in “WHAT IS GETH?”.
Install it as follows:
- If you are a Mac user, install Geth using the instructions in the GitHub Geth installation instructions for Mac. However, you need to install Homebrew first, and you can do that from the Homebrew website.
- If you are a Windows or Linux developer, you can install Geth by following instructions in the Go Ethereum Downloads page.
- Install the CLI using instructions in the Ethereum Command line tools webpage.
Follow reference article 2 for details on commands.
Code and compile your smart contract:
Code a simple smart contract and save it in a file after noting down the filename. The last node of your file name will need to “.sol”.
Hire expert developers for your next project
1,200 top developers
us since 2016
You now need to compile your code into an application binary interface (ABI) and bytecode (bin) for deploying onto the blockchain.
Open a terminal window and navigate to the code file, and do the following:
- Compile your ABI and bin;
- Display contents of compiled files.
Read reference article 2 for the commands you need to use for these steps. Keep your terminal window open, because you will need to refer to the content on display there.
Start a Geth node:
Open a new command prompt, and then run commands mentioned in reference article 2 to start your Geth node. We want to connect to the Rinkeby testnet, hence the commands are focused on tthat.
You also need to create an account with the Rinkeby testnet. To do so, follow instructions from “How to get on Rinkeby Testnet in less than 10 minutes”.
The output you see in the console will show some information, for e.g. your ‘coinbase’ address, the data directory, etc. You need to get Rinkeby test Ether for your smart contracts deployment, and you can get that in the Rinkeby Faucet.
Assuming this is the first time you are using Geth, remember that it will take some time for the blockchain to sync. You need to wait until the ‘current block’ matches the ‘highest block’.
Deploying smart contracts from Geth:
You need to do the following:
- Unlock your account with the passphrase.
- Set up your ABI and bytecode variables using contents displayed in the terminal window when you had compiled your contract.
- Deploy your contract using the account information, bytecode information, and your contract filename.
Check out the detailed commands in reference article 2. This completes deploying a smart contract onto the Rinkeby testnet.
Implement smart contract in DApp onto the Ethereum mainnet:
In this third approach, I will describe deploying smart contracts onto the Ethereum mainnet using testrpc, web3js, and Truffle.
This is the approach I described in “How to Build Online Marketplace on Blockchain Like OpenBazaar?”, where I had detailed the steps to build a DApp marketplace.
Create an Ethereum account for yourself:
To deploy your smart contract onto the Ethereum mainnet, you will need to spend real Ether, hence you need to create an account first. There are two kinds of Ethereum account, namely ‘EOA’ and ‘Contract accounts’.
Contract accounts hold smart contracts and are entirely controlled by code. People joining the network use EOA account, and so you need to create this.
An easy way to do this is to use ‘eth-lightwallet’. You can create your public key-private key pair easily, and you can use the “eth-lightwallet” documentation in GitHub to get instructions. Remember that you should never share your private key with anyone or upload to any website or cloud platform.
Install and configure testrpc, web3js, and Truffle tools:
Let’s first install the required tools, as follows:
- testrpc: It’s a popular blockchain client for Ethereum DApp development, and is now part of the Truffle suit of tools. The ease of use is impressive, besides its range of tools, it boasts a CLI too. It will not mine block by default, but you can set block-interval using the CLI for your development. Check out their GitHub instructions to install and configure it.
- Web3js: By now you’re already familiar with it a bit because of the first approach I described, where MetaMask was automatically added web3.js to your browser. In this approach, we will install and configure it using their GitHub instructions. Update the ‘aconfig.js’ file following the instructions. As a tool to communicate with the blockchain, they also provide web APIs, and provide instructions on how to configure them.
- Truffle: You will use this tool to test and deploy your smart contracts. It allows you to deploy your smart contracts in the test environment and test them. There are folders for you to maintain your contracts. Deployment is easy with Truffle. Install and configure it by following their GitHub instructions.
The next step is to modify your config.js file. You need to open testrpc and run an instance. Take the private and public key information from eth-lightwallet and update the config.js file.
While describing this approach, I will ask you to refer to “Getting Started as an Ethereum Web Developer” for commands. Let’s call it “Reference article 3”. You can check this article for instructions to update your config.js file.
Deploying smart contracts using Truffle:
I assume that you have now coded your smart contracts, so it’s time for you to buy some real Ether for your eth-lightwallet. You need to spend it to deploy your contracts onto the mainnet, and you also need to pay ‘gas’ fees to the miner.
Check to make sure that your testrpc instance is still running. Then navigate to your Truffle directory and use the following command: “truffle deploy“. This deploys your contract and provides you with its’ address.
Hire expert developers for your next project
You need to retain the address because you will need it when interacting with your smart contract later. Etherscan is a tool that allows users to search addresses.
Read this reference article 3 for more details about the commands.
This completes the third and final deployment approach in this guide.
Pay special attention to make your smart contracts bug-free through thorough testing, before you deploy them. You can run your smart contracts in ethereum test network using tools such as Ganache. Immutable code and irreversible execution make developing bug-free smart contracts is imperative. Read more about it in “What Is A Smart Contract?”.
That’s it. You should now have a deployed smart contract on Ethereum.
Interested in Developing Smart Contracts?
Blockchain smart contract technology is changing the working landscape of almost all industries from finance, healtcare, supply chain, etc. The emerging blockchain technology is decentralized, transparent, and more secure.
Although, blockchain network development comes with its challenges, its benefits are still found attractive by businesses.
If you, as a business CEO or CTO, are planning to engage in smart contract technology for your business processes, be sure to adopt the right development tools, platforms, and methodologies. No doubt, you will need skilled software developers with expertise in niche blockchain skillset.
If you want to partner with experienced blockchain developers, why not fill out a DevTeam.Space project specification form and one of our dedicated account managers will get in touch to answer any questions you might have.
DevTeam.Space platform offers top developers, dev teams, managers, and designers who are expert in all the major tech stacks. We have a unique development methodology that allows our developers to smoothly onboard onto any project.
Further Reading
Here are a few articles that might also interest you:
https://www.devteam.space/blog/what-is-an-initial-exchange-offering/
Interested in some tips and tricks for VR application development? This is a great market that offers huge profits for original apps. Let's start by discussing some of the essential features of virtual reality applications. Desired...
Continue reading
Interested in virtualization techniques in cloud computing? You've come to the right place. 'Virtualization' is defined as the act of “creating a virtual (rather than actual) version of something, including virtual computer hardware...
Continue reading
Interested in Typescript vs Javascript: Which One is the Best? This is a hotly debated issue that we will cover in this article. The question of which one is best in the Typescript vs Javascript battle is a difficult one. As with any...
Continue readingFrequently Asked Questions
Smart contracts are added to the blockchain where the Ethereum Virtual Machine executes a series of commands in order to fulfill the criteria outlined by the smart contract once the conditions for its execution have been fulfilled.
You will need to code your new smart contract using Solidity. After you have done this you will need to deploy smart contracts to the Ethereum network. If you don’t have the knowledge on how to do this then you should onboard specialist smart contract developers from DevTeam.Space.
It is the specific address on the Ethereum blockchain where your smart contract code resides.
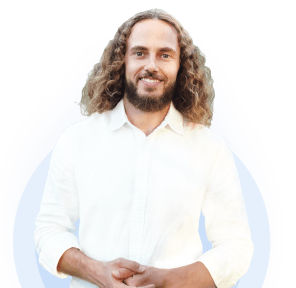
Alexey Semeney
Founder of DevTeam.Space
Hire Alexey and His Team To Build a Great Product
Alexey is the founder of DevTeam.Space. He is award nominee among TOP 26 mentors of FI's 'Global Startup Mentor Awards'.
Alexey is Expert Startup Review Panel member and advices the oldest angel investment group in Silicon Valley on products investment deals.