- Developers
- Developer Blog
- Mobile App Development
- How To Develop Ruby Android Apps?
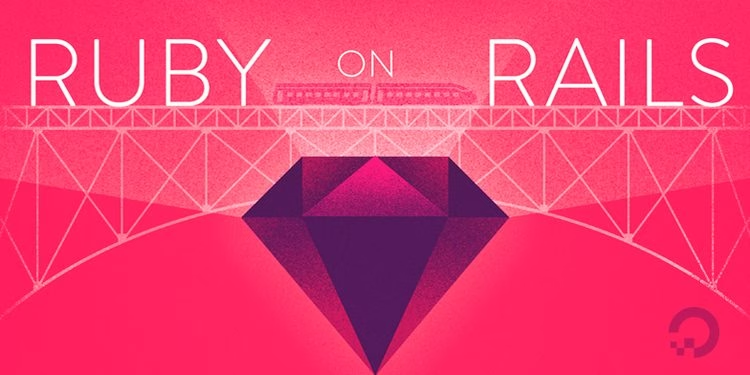
profile

By Aran Davies
Verified Expert
8 years of experience
Aran Davies is a full-stack software development engineer and tech writer with experience in Web and Mobile technologies. He is a tech nomad and has seen it all.
Interested in how to develop Ruby Android apps? That’s an excellent market with many opportunities to be explored.
In this article, we will see how you can develop ruby android apps. Let’s start.
How can you develop Ruby Android apps?
I will now take you through the development process in regards to Android applications using Ruby:
Step #1: Use the Agile methodology
Your first step should be to choose the right software development life cycle (SDLC) model. I recommend Agile, due to the following reasons:
- An Android app will require iterative development.
- You will benefit from launching a “Minimum Viable Product” (MVP) and getting real market feedback. This will help you in course correction if the need arises. You can implement the remaining features in the subsequent iterations.
You can refer to “What is software development life cycle and what you plan for?”, where I have explained the advantages of the Agile SDLC model.
Step #2: Form your team, choose an MBaaS provider
You will need the following roles in your team:
- Business analysts;
- UI/UX designers;
- Ruby developers;
- Android developers with Java expertise;
- Testers;
- A project manager (PM).
For this project, I recommend you use a “Mobile Backend as a Service” (MBaaS) provider. Google Firebase is a reputed MBaaS provider. You get the following advantages if you engage an MBaaS provider:
- MBaaS providers handle cloud infrastructure.
- They address persistent storage and database.
- An MBaaS provider deals with user management, security, 3rd party API integration, scalability, and push notifications.
- You don’t need to hire cloud infrastructure architects and backend developers. Your team can focus on writing Ruby code for the front-end.
I have explained the advantages of MBaaS in “How to choose the best Mobile Backend as a Service (MBaaS)?”.
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
Step #3: Design an elegant and user-friendly UI
The “User Interface” (UI) of a mobile app is important for attracting and retaining users. Your UI/UX design team needs to design a simple yet attractive UI. Consider the following:
- Designers need to choose the right mobile navigation menu design. There are several options, e.g., the “Hamburger” menu, “Tab bar”, “Priority +” menu, etc. Consult our guide “Mobile navigation menu examples”.
- The design team should select a mobile app color scheme that suits the features, functionalities, and target audience of the app. There are several options, e.g., minimal color usage with focused palettes, high-contrast colors, etc. You can read “8 trends in mobile app color scheme” for more insights.
- UI designers need to carefully design the icons for your app. There are several best practices, e.g., using restraint in design, designing recognizable icons that are easier to memorize, keeping the design simple, etc. Our guide “How to design the perfect icon for your mobile app?” can help your team.
Step #4: Use RubyMotion
RubyMotion is a Ruby language implementation. This can run on Android, moreover, it also works on iOS and OS X. Following are a few relevant facts concerning RubyMotion:
- It has a unified runtime approach. It also includes optimized binary support using a static compiler.
- Programmers can create apps from the terminal command-line interface (CLI). They can write code and see the changes in real-time, using the console.
- There is robust integration with the Android runtime. RubyMotion supports the latest Android version.
- RubyMotion has testing tools and supports interactive debugging.
- Developers can access the entire collection of Android APIs, moreover, they can use 3rd party Java Archive files (JARs).
- RubyMotion makes it easy to submit the app to Google Play.
Read more on the RubyMotion “Features” page. I recommend you use RubyMotion for this project.
Step #5: Install and configure the required development tools
You need to install and configure the following to use RubyMotion effectively:
- Download RubyMotion: Follow the instructions that you find here.
- Install Java: RubyMotion needs a Java compiler. Follow the instructions in “How do I install Java?” to install Java.
- Set up the Android environment: You need to follow the instructions in “Getting started with RubyMotion for Android”.
- Download and install the Genymotion Android emulator: You can follow the instructions on their website.
- Configure a device for development: Follow the instructions in “Getting started with RubyMotion for Android”.
- Install the RubyMine text editor: This is from JetBrains, i.e., the company behind the famous IDE IntelliJ IDEA. It works well with RubyMotion. You can download it from here, and follow their instructions for installation.
Step #6: Create a project for your app using RubyMotion
Setting up a project for your app using RubyMotion is the next step, and involves the following:
- Creating a new project using RubyMotion, including the appropriate directories;
- Configuring the project for subsequent development;
- Using the configuration options, setting custom values, declaring file dependencies, etc.
Follow the guidelines in “RubyMotion project management guide for Android”.
Step #7: Use the RubyMotion runtime guide for coding
You are now in the coding stage, and you will likely need some assistance with mobile development. Note the following:
- RubyMotion includes a runtime for Android, moreover, it includes the core Java classes.
- The object model of RubyMotion is based on Java. It‘s an implementation of Ruby on “Java Native Interface” (JNI), which the Android runtime uses.
- All RubyMotion objects are Java objects. Therefore, we also get all Java classes and methods available in Ruby.
- Java types interface with Ruby, additionally, RubyMotion also has some built-in classes based on the Java class library.
- RubyMotion can access Java methods in the same way as Ruby methods.
- Developers using RubyMotion can define methods to implement interfaces in classes like they do in Java. You have already likely inducted Android developers with Java expertise, however, if the team needs guidance then they can read “Java Interfaces”.
- RubyMotion uses common Android memory management best practices.
You can get more detailed guidance in the “RubyMotion runtime guide for Android”. RubyMotion also has several sample projects. You can access them here, and you can read the code.
Step #8: Build-management using RubyMotion
You need to use RubyMotion for the build management of your app. This involves the following:
- Creating development and release versions;
- Compilation of the code into machine code;
- Linking the machine code with the RubyMotion runtime;
- Generating the appropriate Android bytecode;
- Creation of “.apk” archive for copying the files into it;
- Make the bundle ready with the Java Android Keystore.
Read more in “RubyMotion project management guide for Android”.
Step #9: Debug using RubyMotion
It‘s time to debug the code. RubyMotion offers detailed guidance for debugging, which includes the following:
- Using GDB, i.e., “The GNU Project Debugger”;
- Attaching the debugger, including when you are working with an emulator;
- Managing breakpoints;
- Checking the execution backtrace once the developer hits a breakpoint;
- Inspecting the objects like local variables and instance variables;
- Controlling the execution flow of the program.
You can read “RubyMotion debugging guide for Android” for guidance.
Hire expert developers for your next project
1,200 top developers
us since 2016
Step #10: Test the Android app using RubyMotion
The next step is to write functional test cases and test your app. This involves the following:
- Writing test cases including test specifications;
- Organizing the test cases in the appropriate RubyMotion directories;
- Running tests using appropriate RubyMotion commands.
RubyMotion provides detailed instructions and commands for writing and running tests, and you can find them in “RubyMotion testing guide for Android”.
Step #11: Run your Android app with an emulator
Now that you have tested your app thoroughly, it‘s time to run it. Do the following:
- Install Genymotion, which is a popular Android emulator.
- You can run this on the cloud, or on your desktop.
- Create and start a virtual device.
Execute appropriate commands to run your app on the emulator. You can find the commands in “RubyMotion project management guide for Android”.
Step #12: Publish your Android app on Google Play
Publishing your app on Google Play involves quite a few steps, e.g.:
- Creating an application in “Google Play Console”;
- Preparing an app store listing;
- Answering the content rating questionnaire;
- Managing the APK files;
- Publishing your app.
Read about this process in “Upload an app”.
Step #13: Use the “Scrum” technique to manage your project
While there are several Agile project management techniques, “Scrum” is a time-tested one, and I recommend you use it for this project. This involves the following:
- The PM performs the role of a “Scrum master” and creates a cross-functional “Scrum team”.
- A “Product owner” provides the features, i.e., requirements in a document called the “Product Backlog”.
- The team estimates the features, considers the priorities, and slots them into “Sprints”, i.e., iterations.
- The team conducts a set of meetings during the project execution, as follows:
- “Daily stand-up meeting” to discuss the project status;
- “Sprint review meeting” to demonstrate the app, where the project stakeholders approve the sprint provided the features work;
- “Sprint retrospective meeting” to learn lessons from a sprint.
You can read our guide “How to build a Scrum development team?” for more details.
Embarking on a Ruby Android app development project?
Android development using Java or Kotlin is common, however, Ruby Android apps development is less common.
You will need to find Ruby developers experienced with Android development, moreover, you need to manage the project end-to-end.
If you don’t have the expertise then consider getting professional help. Read our guide “How to find the best software development company?” before you engage a development partner.
Hire expert developers for your next project
DevTeam.Space can also help you here with its field-expert software developer community experienced in developing market-competitive cross-platform native apps for businesses using cutting-edge technologies.
You can easily partner with these competent app developers by writing to us your initial ruby android apps specifications via this quick form and one of our account managers will get back to you to provide further assistance with the onboarding process.
Further Reading
Here are a few articles that might also interest you:
Interested in knowing how to use AI to improve quality control? This is a great question about an excellent market that still is in its infancy. According to a study, "AI is poised to reach over US6.4 Billion by the year...
Continue reading
Want to know how to use a safe VPN or a virtual private network to secure your Android phone? Online privacy and data security are increasingly becoming a thing of the past. As the recent Facebook scandals prove, your data is available...
Continue reading
Do you want to know how to turn around a failing software project? There are huge sums of money to gain by launching a successful software project. Naturally, all product owners and developers hope to succeed with their software...
Continue readinghttps://www.devteam.space/blog/how-to-save-money-when-building-a-mobile-app/
Frequently Asked Questions on Ruby Android Apps
The most popular apps that use Ruby include Airbnb, GitHub, Hulu, and Shopify. Thanks to its popularity among developers, Ruby has been used to build 10,000s of software applications.
Ruby on Rails was designed to create performative apps for both iOS and Android apps. While Ruby is an excellent programming language, the Rails framework allows a range of default settings to be applied to speed up development and reduce errors.
Create a project specification. Onboard managed developers from DevTeam.Space. Set up the infrastructure and communication channels. Outline tasks and sprints. Set the ball rolling.
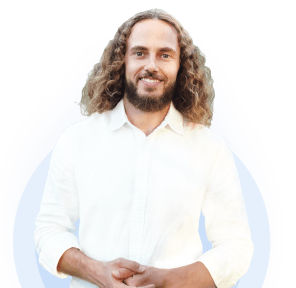
Alexey Semeney
Founder of DevTeam.Space
Hire Alexey and His Team To Build a Great Product
Alexey is the founder of DevTeam.Space. He is award nominee among TOP 26 mentors of FI's 'Global Startup Mentor Awards'.
Alexey is Expert Startup Review Panel member and advices the oldest angel investment group in Silicon Valley on products investment deals.