- Developers
- Developer Blog
- Software Development
- Java Code Review Checklist – What to Include?
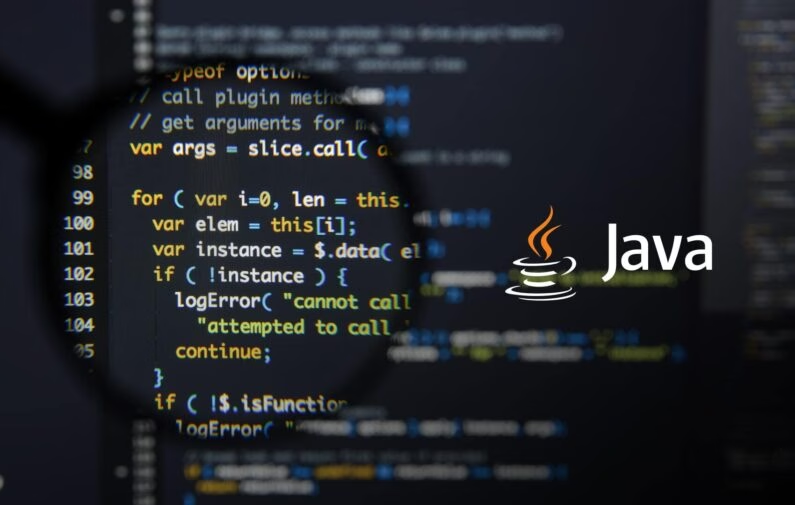
profile

By Faiza Khalid
Verified Expert
5 years of experience
Faiza is a CIS engineer with a keen interest in software development, AI research, and technology writing.
Are you looking for a Java code review checklist?
According to a survey, developers spend 45% of their time fixing bugs rather than writing new code. Strict code reviews allow developers to spend less time resolving bugs and delivering new features.
Developing a Java application requires a deep understanding of object-oriented programming, Java language, tools like Eclipse IDE, Java Standard Library, Git version control, and frameworks like Hibernate, Spring Boot, etc. You also require skills in database management, like JDBC (Java Database Connectivity) and ORM (object-relational mapping), application security practices, performance optimization, etc.
If you don’t have a professional team with this relevant expertise to take on the task, then submit a request for a complimentary discovery call, and one of our tech account managers who managed similar projects will contact you shortly.
Let’s discuss Java code review checklist items to ensure a well-written, functional, and maintainable software application.
Java Code Review Checklist
Check off the following when performing a Java code review:
Functional Check
Functional checks ensure the software works as intended. Review the following:
- The code logic correctly implements the required functionality.
- Check input validation and output accuracy. The code should validate input values to avert unexpected behavior, data corruption, etc., and verify the actual output matches the expected outcome.
- There are no functional regressions or inconsistencies in case of changes to the existing code.
- The functionality is implemented in a simple and reusable manner. Check for the following design principles:
- DRY (Don’t Repeat Yourself), SOLID (Single-responsibility Principle, Open-closed Principle, Liskov Substitution Principle, Interface Segregation Principle, and Dependency Inversion Principle), etc.
- Observe object-oriented principles of Abstraction, Polymorphism, Inheritance, and Encapsulation.
Clean Code
You should check for the following to ensure a clean code:
Get a complimentary discovery call and a free ballpark estimate for your project
Trusted by 100x of startups and companies like
Naming Conventions
- Follow Java code conventions to enhance code readability. All package names in Java are written in lower case, all constants in upper case, variables in CamelCase, etc.
- Use meaningful and descriptive names instead of relying on comments to understand code.
- For example, calculateTax(BigDecimal amount).
No Duplication
- There should be no code duplication. Classes and methods should focus on one functionality.
- Declare variables with the smallest scope. E.g., if a variable is used inside a loop, declare it inside the loop.
- Developers should follow a single code formatting template.
No One-Liners
- Minimize using one-liners, especially with variable initialization and operation in the same line.
For instance, write the following code:
System.out.println(attrs.get("offset") + "-" + Math.min(attrs.get("count"), attrs.get("offset") + attrs.get("max")) + " " + title + " " + attrs.get("count"));
As:
int start = attrs.get("offset");
int total = attrs.get("count");
int end = Math.min(total, start + attrs.get("max"));
System.out.println(start + "-" + end + " " + title + " " + total);
No White-Spaces
Use white spaces to separate code for better readability. For example, write this:
Integer.valueOf(params.offset?params.offset:attrs.offset)
As:
Integer.valueOf(params.offset ? params.offset : attrs.offset)
- Generally, white spaces are not added in parentheses. E.g., if (total > 0) instead of if ( total > 0 )
Clean Up
Consider the following for a clean code:
- Remove unnecessary comments or obsolete code.
- Use @deprecated annotation for variables or methods that are to be removed and will not be used in the future.
- Remove hard-coded variables.
- Use switch case instead of multiple if else conditions.
- Use logging instead of console print statements (SOPs).
Java Fundamental Code Practices
These include the following:
Using Immutable Classes
Make objects immutable where possible. Immutable objects are thread-safe and more secure.
- Declare classes as final to prevent their modification after initialization.
- There are no setter methods that allow modification of its internal states.
- Mutable objects in a class are deep copied to maintain immutable properties.
- All mutable objects are initialized within the constructor.
- Immutable variants are used from java.util package in case a class contains collections.
- Immutability is maintained in subclasses.
Use mutable objects when they serve the purpose better. For example, instead of creating a new string object for every concatenation operation, use a mutable object.
Protected Accessibility
Review that a field or a member is accessible within its own class, subclass, and classes within the same package. Ensure the following for protected accessibility:
- Use of a protected modifier when required by code design.
- Protected members are used for polymorphism and inheritance purposes for code reusability.
- The use of protected members does not introduce tight coupling.
- Keep everything private by default and only expose methods to a client code when necessary.
- Exposing classes or methods, for instance, via a public modifier, can affect code encapsulation. Another dev team could add functionality that you do not want, etc.
Code to Interfaces
The principle of code to interfaces avoids concrete implementations and supports programming against interfaces. Consider the following when verifying the code-to-interface principle in your code:
- Classes are referencing interfaces, e.g., List, Map, etc., instead of ArrayList, HashMap, etc., as they introduce tight coupling.
- Interfaces allow different implementations interchangeably (polymorphism).
- Dependency injections make it easier to modify implementations.
- There is easier integration of third-party libraries or new components.
- Interfaces adhere to interface segregation principle (ISP).
Overriding Hashcode
Objects that are equal due to their values should override the equals methods to return true in case of the same values. The equals method is usually used for comparison and equality checks. Therefore, overriding the equals method is essential.
Each Java object has a hash code value. Two equal objects must have the same hash code. Ensure the two objects are equal if they override both hashcode and equality methods.
Hire expert software developers for your next project
1,200 top developers
us since 2016
Direct References
Carefully review direct references to fields in the client code. Direct references can be manipulated even if you use ‘final’. Instead, clone a reference or create a new reference and then assign it to the field.
Consider the following example:
public static void main(String[] args) {
ImmutablePerson person = new ImmutablePerson("Alice");
System.out.println("Original name: " + person.getName());
// Attempt to modify the reference to the ImmutablePerson
modifyReference(person);
// Despite the field being final, the name can still be manipulated.
System.out.println("Name after modification: " + person.getName());
}
private static void modifyReference(ImmutablePerson person) {
// The final field "name" is not being modified here.
// Instead, a new reference to a different ImmutablePerson object is assigned.
person = new ImmutablePerson("Bob");
}
}
Although the field is declared final, the reference to an ImmutablePerson object can be reassigned to another object. It indicates the reference is mutable.
In the modifyReference method, a new ImmoytablePerson object is created and assigned to the person reference. This does not modify the original ImmutablePerson object referenced by the person reference in the main method.
Exception Handling
- Stay clear of NULL pointer exceptions. Avoid returning null values when possible. A good practice is to check for null values before calling a method to avoid NULL pointer exceptions. Consider the following code:
boolean isEven = item % 2 == 0; // throws NullPointerException
Write the above code as: boolean isEven = item != null && item % 2 == 0
- Consider using the OPTIONAL class for variables that may have invalid states, like this:
items.highest().ifPresent(integer -> {
boolean isEven = integer % 2 == 0;
});
- @NULL and @NonNull annotations also show NULL warnings to developers while building the code.
- Ensure closing all resources, such as database connections, in the Finally block of try-catch exception handling.
- Consider throwing custom exceptions instead of generic ones, which makes it easier to handle and debug code.
- Use checked exceptions for recoverable operations and runtime exceptions for programming errors.
Security Checks
Consider the following security checks when reviewing Java code:
- Do not log sensitive information;
- Use immutable objects;
- Release resources after use, such as streams, connections, etc., to prevent issues like resource leaks, denial of service, etc.
- Include sensitive information in exception blocks, like file paths, server names, etc.
- Implement security practices like authorization, authentication, SSL encryption, etc.
General Programming Checks
These include the following:
Using Frameworks and Libraries
Leverage ready-to-use frameworks and libraries instead of writing all the code from scratch.
Some popular Java libraries and frameworks include:
- Apache Commons;
- Guava;
- Jackson;
- Logback;
- Hibernate Validator;
- Spring Boot;
- Spark;
- Grails;
- Apache Struts, etc.
Choice of Data Structures
Use appropriate data structures. Java programming language provides collections like ArrayList, LinkedList, Vector, Stack, HashSet, HashMap, etc. Your developers should know the pros and cons of using each. For example:
- Map data structures are useful for unordered items that require efficient retrieval, insertion, etc.
- The list structure is commonly used for ordered items that could contain duplicate values.
- The set is similar to the List but without duplicates.
Also, ensure the use of the appropriate data types in your code. For example, use BigDecimal instead of float or double, enums for int constants, etc. Use static values, dynamic values, database-driven values, etc., appropriately.
Unit Testing
Testing code modules form an essential part of a Java code review checklist.
Hire expert software developers for your next project
- Review the use of unit testing. Developers should use unit test cases with mock objects for easy and repetitive testing.
- Unit test cases should run independently of each other.
- Do not include external services, such as data retrieval from a database, in unit testing.
Implementing Non-Functional Requirements
Ensure non-functional requirements, such as performance, scalability, monitoring, etc., are met. Consider the following:
- Check for potential bottlenecks, excessive resource utilization, etc.
- Review caching mechanisms for performance.
- Check if the code is adjustable for future scalability requirements, like a surge in user traffic.
- Ensure the application handles failures gracefully with proper recovery processes in place.
- Check if the code is modular without excessive complexity for easy maintainability in the future.
Separation of Concerns
Assess if your Java code follows the separation of concerns principle, which confirms that a program divides into separate sections, each responsible for a specific functionality. Consider the following under separation of concerns:
- Single responsibility principle in which each class or method has a single well-defined responsibility.
- The layered architecture ensures the separation of presentation, business logic, data access, etc.
- Ensure modules follow loose coupling, where a change in one component does not affect others.
- Aspect-oriented programming is where cross-cutting aspects of an application, such as security, logging, etc., are separate from core features.
Planning for a Java Code Review?
These Java code review checklist items will help you deliver high-quality code that meets all the functional and non-functional requirements.
Code review is a critical process as it ensures the successful deployment of software. Expert developers conduct successful code review processes. You need exceptional Java developers to plan Java application design, development, testing, and deployment.
If you do not find high-quality Java developers on your team, DevTeam.Space can help you via field-expert software developers.
These developers can help you with smooth product development and comprehensive code reviews owing to their extensive experience building cutting-edge software solutions using the latest technologies and tools.
If you wish to know more about how we can help you with Java application development and code reviewing, send us your initial project specifications via this quick form. One of our account managers will contact you shortly for further discussion.
FAQs on Java Code Review Checklists
A good Java code review process ensures adherence to the standards, code quality, performance, etc. Check for programming errors, code modularity, functionality, code conventions, security practices, runtime exceptions, unit tests, etc.
Developers can check Java code quality through manual code reviews, code metrics (complexity, number of lines, etc.), established programming guidelines, dependency management, code refactoring, etc.
Some tools to review Java code include SonarQube, CheckStyle, ESLint with Java Plugin, Lint4j, etc. You need expert developers to use these tools and conduct static code analysis. Find experienced developers at DevTeam.Space for detailed code reviews.
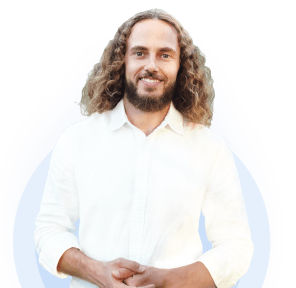
Alexey Semeney
Founder of DevTeam.Space
Hire Alexey and His Team To Build a Great Product
Alexey is the founder of DevTeam.Space. He is award nominee among TOP 26 mentors of FI's 'Global Startup Mentor Awards'.
Alexey is Expert Startup Review Panel member and advices the oldest angel investment group in Silicon Valley on products investment deals.